-
Bitcoin
$94,837.8932
0.65% -
Ethereum
$1,807.2327
-0.09% -
Tether USDt
$1.0005
0.00% -
XRP
$2.3425
7.71% -
BNB
$606.2193
1.04% -
Solana
$151.2638
2.21% -
USDC
$0.9998
-0.01% -
Dogecoin
$0.1815
0.40% -
Cardano
$0.7252
3.33% -
TRON
$0.2470
-1.64% -
Sui
$3.6926
1.53% -
Chainlink
$15.0603
3.04% -
Avalanche
$22.3490
-0.03% -
Stellar
$0.2928
2.53% -
Toncoin
$3.3269
1.02% -
Hedera
$0.1977
4.40% -
UNUS SED LEO
$8.9974
-0.10% -
Shiba Inu
$0.0...01399
1.01% -
Bitcoin Cash
$355.2322
0.55% -
Polkadot
$4.2390
2.19% -
Litecoin
$87.9136
2.48% -
Hyperliquid
$18.3407
4.55% -
Dai
$1.0001
0.01% -
Monero
$289.1502
26.24% -
Bitget Token
$4.4127
0.33% -
Ethena USDe
$0.9996
0.01% -
Pi
$0.6241
-2.15% -
Pepe
$0.0...09020
-0.55% -
Aptos
$5.6495
2.20% -
Uniswap
$5.5347
-3.67%
How to use Python API on Bitfinex?
The Bitfinex Python API enables programmatic trading and portfolio management, requiring setup of the environment, authentication, and careful handling of requests and errors.
Apr 23, 2025 at 12:36 am
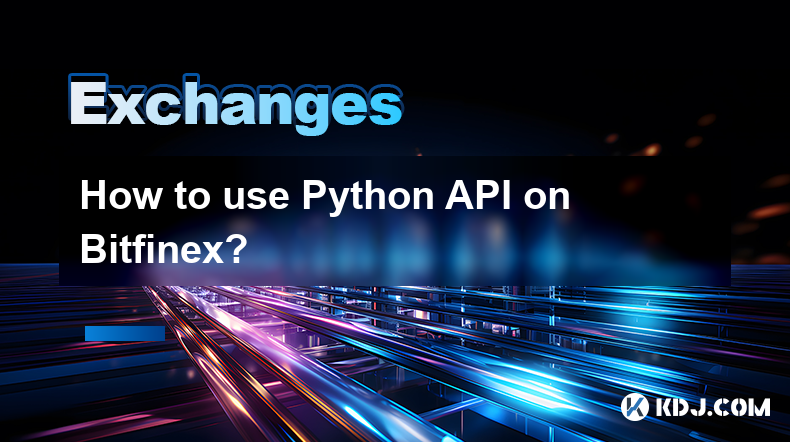
Using the Python API on Bitfinex allows traders and developers to interact with the Bitfinex exchange programmatically. This article will guide you through the process of setting up and using the Bitfinex Python API, covering authentication, making API requests, and some common use cases.
Setting Up the Environment
Before you can start using the Bitfinex API, you need to set up your development environment. This involves installing the necessary Python package and setting up your API keys.
Install the Bitfinex Python client: The first step is to install the Bitfinex Python client. You can do this using pip, Python's package installer. Open your terminal or command prompt and run the following command:
pip install bitfinex
Obtain API Keys: To interact with the Bitfinex API, you need to have API keys. Log into your Bitfinex account, navigate to the API section, and generate a new API key. Make sure to keep your API key and secret safe and never share them with anyone.
Set up Environment Variables: It's a good practice to store your API keys as environment variables. You can set them up in your operating system or in your Python script. Here's how you can do it in a Python script:
import os
os.environ['BITFINEX_API_KEY'] = 'your_api_key'
os.environ['BITFINEX_API_SECRET'] = 'your_api_secret'
Authenticating with the API
Once your environment is set up, you need to authenticate with the Bitfinex API. This involves creating a client object using your API key and secret.
Create a Client Object: Use the following code to create a client object:
from bitfinex import ClientV2 as Client
api_key = os.environ['BITFINEX_API_KEY']
api_secret = os.environ['BITFINEX_API_SECRET']client = Client(api_key, api_secret)
This client object will be used to make authenticated requests to the Bitfinex API.
Making API Requests
With the client object created, you can now make various API requests to interact with the Bitfinex exchange. Here are some common types of requests you might want to make.
Fetch Account Balances: To get your account balances, use the following code:
balances = client.balances()
for balance in balances:print(f"Currency: {balance['currency']}, Amount: {balance['amount']}")
Place an Order: To place an order, you can use the following code:
order = client.place_order( symbol='tBTCUSD', amount='0.01', price='50000', side='buy', type='exchange limit'
)
print(f"Order ID: {order['id']}")Fetch Order History: To retrieve your order history, use the following code:
orders = client.orders()
for order in orders:print(f"Order ID: {order['id']}, Symbol: {order['symbol']}, Side: {order['side']}")
Handling Responses and Errors
When making API requests, it's important to handle responses and errors properly. The Bitfinex API returns JSON responses, which you can parse and handle in your Python script.
Parsing Responses: Here's how you can parse and handle a response:
try: response = client.balances() for balance in response: print(f"Currency: {balance['currency']}, Amount: {balance['amount']}")
except Exception as e:
print(f"An error occurred: {e}")
Error Handling: Make sure to handle errors gracefully. The Bitfinex API might return errors for various reasons, such as invalid parameters or authentication issues. Use try-except blocks to handle these errors:
try: order = client.place_order( symbol='tBTCUSD', amount='0.01', price='50000', side='buy', type='exchange limit' ) print(f"Order ID: {order['id']}")
except Exception as e:
print(f"Failed to place order: {e}")
Common Use Cases
Here are some common use cases for using the Bitfinex Python API.
Automated Trading: You can use the API to automate your trading strategies. For example, you can implement a simple moving average crossover strategy:
import time
def moving_average_crossover(client, symbol, short_window, long_window):
while True: try: # Fetch the latest candlestick data candles = client.candles(symbol=symbol, timeframe='1m', limit=1000) # Calculate moving averages short_ma = sum([candle[2] for candle in candles[-short_window:]]) / short_window long_ma = sum([candle[2] for candle in candles[-long_window:]]) / long_window if short_ma > long_ma: # Place a buy order client.place_order( symbol=symbol, amount='0.01', price=str(candles[-1][2]), # Current price side='buy', type='exchange market' ) print(f"Bought {symbol} at {candles[-1][2]}") elif short_ma < long_ma: # Place a sell order client.place_order( symbol=symbol, amount='0.01', price=str(candles[-1][2]), # Current price side='sell', type='exchange market' ) print(f"Sold {symbol} at {candles[-1][2]}") time.sleep(60) # Wait for 1 minute before checking again except Exception as e: print(f"An error occurred: {e}") time.sleep(60) # Wait for 1 minute before retrying
Example usage
moving_average_crossover(client, 'tBTCUSD', 50, 200)
Portfolio Management: You can use the API to manage your portfolio by regularly checking your balances and adjusting your positions accordingly:
def manage_portfolio(client):
while True: try: balances = client.balances() for balance in balances: if balance['currency'] == 'USD' and float(balance['amount']) > 1000: # If you have more than $1000 in USD, buy more BTC client.place_order( symbol='tBTCUSD', amount=str(float(balance['amount']) / 50000), # Assuming BTC price is $50,000 price='50000', side='buy', type='exchange limit' ) print(f"Bought BTC with {balance['amount']} USD") time.sleep(3600) # Wait for 1 hour before checking again except Exception as e: print(f"An error occurred: {e}") time.sleep(60) # Wait for 1 minute before retrying
Example usage
manage_portfolio(client)
FAQs
Q: Can I use the Bitfinex Python API to trade multiple cryptocurrencies at once?
A: Yes, you can use the Bitfinex Python API to trade multiple cryptocurrencies. You can loop through different symbols and place orders for each one as needed. Make sure to handle each request separately and manage your API rate limits.
Q: How do I handle rate limiting when using the Bitfinex API?
A: Bitfinex has rate limits to prevent abuse. You can handle rate limiting by implementing a delay between API requests or by using the API's rate limit headers to dynamically adjust your request frequency. Always check the API documentation for the latest rate limit information.
Q: Is it safe to store my API keys in environment variables?
A: Storing API keys in environment variables is generally considered safer than hardcoding them in your script. However, ensure that your environment variables are properly secured and not accessible to unauthorized users. Consider using a secrets manager for added security.
Q: Can I use the Bitfinex Python API for real-time data streaming?
A: Yes, the Bitfinex API supports real-time data streaming through WebSockets. You can use the bitfinex
library to set up a WebSocket connection and receive real-time updates on trades, order books, and more.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Lightchain AI (LCAI) Prepares to Surpass Cardano (ADA) as It Continues Its Progress
- 2025-04-28 16:20:13
- Web3 Carnival From April 6 to 9, the 2025 Web3 Carnival initiated by Wanxiang Blockchain Lab and HashKey Group was successfully held in Hong Kong
- 2025-04-28 16:20:13
- Nothing's sub-brand CMF is all set to launch the CMF Phone 2 Pro today
- 2025-04-28 16:15:13
- Bitcoin (BTC) Price Starts Downside Correction From the $95,000 Zone
- 2025-04-28 16:15:13
- Nike Faces Lawsuit From Its NFT Customers Due to 'Rug Pull' of RTFKT
- 2025-04-28 16:10:13
- Rising Macro Tensions and Institutional Support Fuel Bitcoin's Rebound
- 2025-04-28 16:10:13
Related knowledge
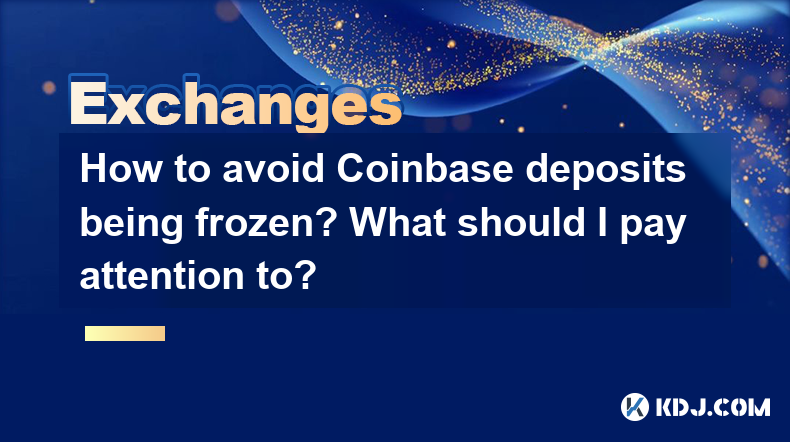
How to avoid Coinbase deposits being frozen? What should I pay attention to?
Apr 27,2025 at 11:57pm
Understanding Coinbase Deposit FreezingCoinbase, one of the largest cryptocurrency exchanges, occasionally freezes deposits for various reasons. Understanding why your deposits might be frozen is crucial for preventing such occurrences. Common reasons include suspicious activity, account verification issues, or failure to comply with regulatory requirem...
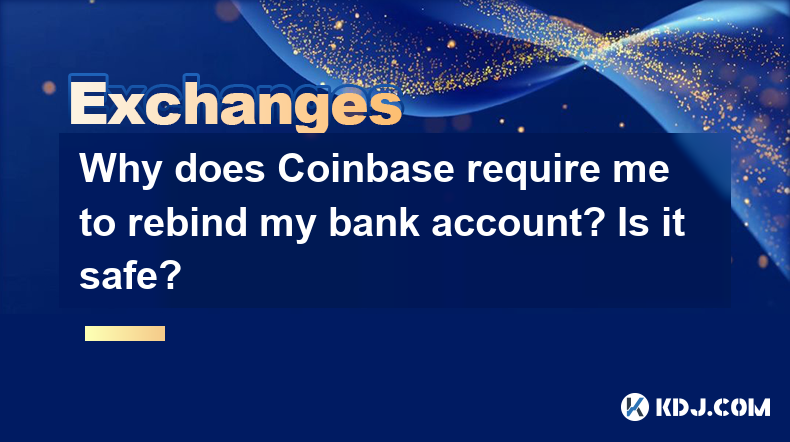
Why does Coinbase require me to rebind my bank account? Is it safe?
Apr 28,2025 at 12:07am
Why Does Coinbase Require Me to Rebind My Bank Account? Coinbase, one of the leading cryptocurrency exchanges, occasionally requires users to rebind their bank accounts. This process involves re-verifying and updating the connection between your Coinbase account and your bank account. The primary reasons for this requirement are to enhance security, com...
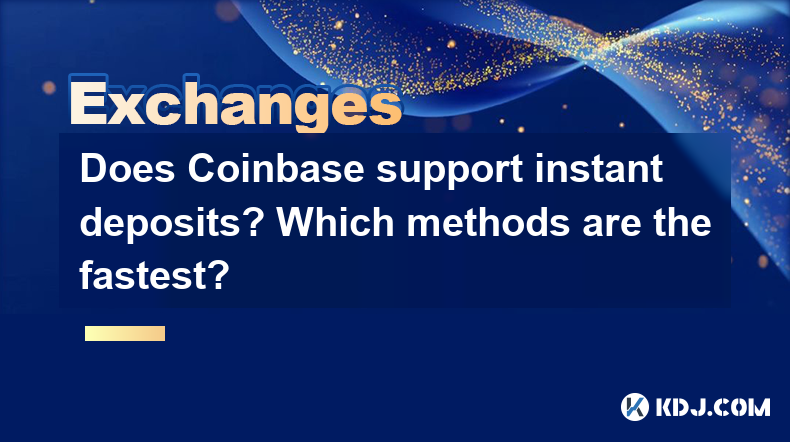
Does Coinbase support instant deposits? Which methods are the fastest?
Apr 28,2025 at 03:35pm
Coinbase, one of the leading cryptocurrency exchanges, offers various methods for users to deposit funds into their accounts. Many users are keen to understand whether Coinbase supports instant deposits and which methods are the fastest. This article will delve into these topics, providing a comprehensive overview of the deposit options available on Coi...
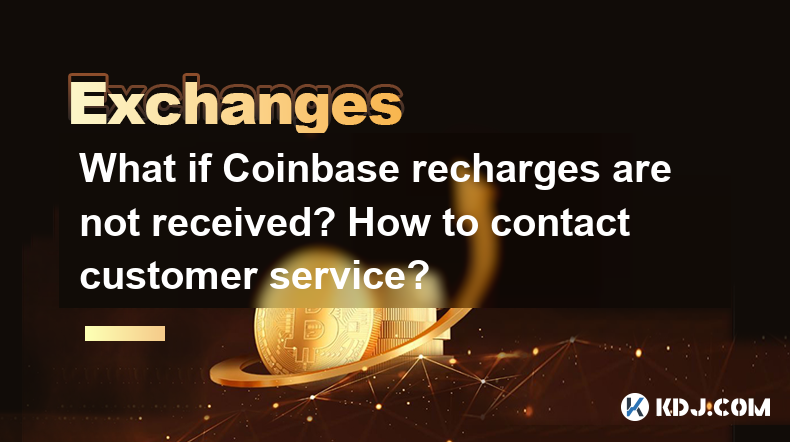
What if Coinbase recharges are not received? How to contact customer service?
Apr 28,2025 at 08:22am
When you send cryptocurrency to your Coinbase account and the recharge is not received, it can be a frustrating experience. This situation can arise due to various reasons, such as network congestion, incorrect address input, or delays in transaction processing. Understanding how to address this issue and contact Coinbase customer service effectively is...
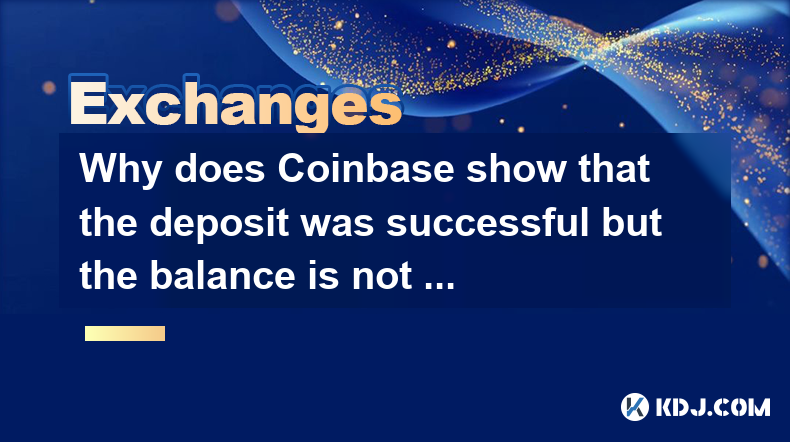
Why does Coinbase show that the deposit was successful but the balance is not updated?
Apr 28,2025 at 03:14pm
Introduction to the IssueWhen using Coinbase, one common issue users face is that their deposit appears to be successful, but their balance does not reflect the deposited amount. This discrepancy can be frustrating and confusing. Understanding why Coinbase shows a successful deposit but does not update the balance is crucial for users to manage their fu...
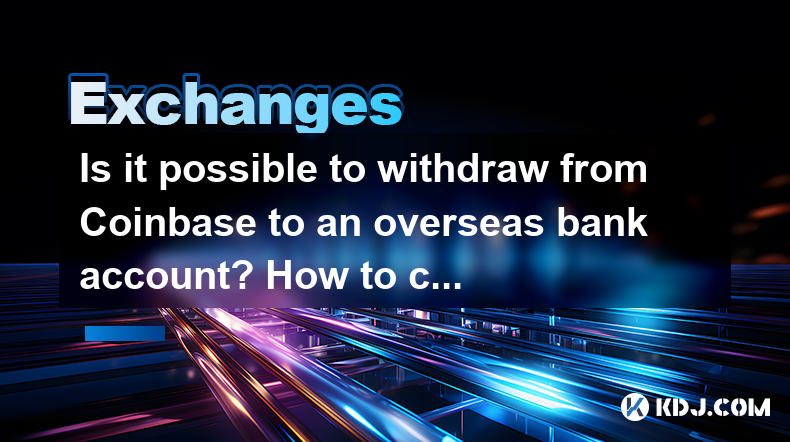
Is it possible to withdraw from Coinbase to an overseas bank account? How to calculate the exchange rate?
Apr 28,2025 at 09:14am
Introduction to Coinbase Withdrawals to Overseas Bank AccountsCoinbase is one of the leading cryptocurrency exchanges that allows users to buy, sell, and store various cryptocurrencies. One of the common queries users have is whether it's possible to withdraw funds from Coinbase directly to an overseas bank account. The answer is yes, it is possible, bu...
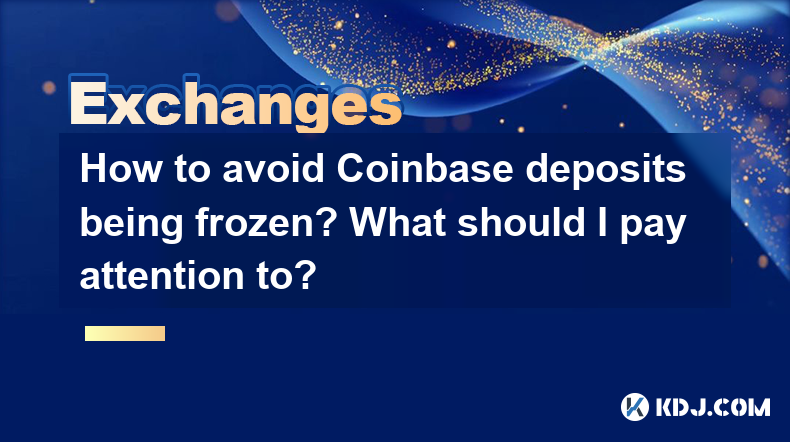
How to avoid Coinbase deposits being frozen? What should I pay attention to?
Apr 27,2025 at 11:57pm
Understanding Coinbase Deposit FreezingCoinbase, one of the largest cryptocurrency exchanges, occasionally freezes deposits for various reasons. Understanding why your deposits might be frozen is crucial for preventing such occurrences. Common reasons include suspicious activity, account verification issues, or failure to comply with regulatory requirem...
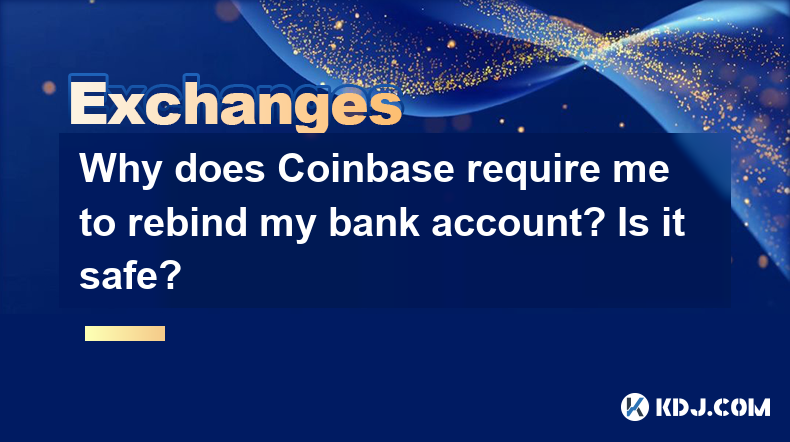
Why does Coinbase require me to rebind my bank account? Is it safe?
Apr 28,2025 at 12:07am
Why Does Coinbase Require Me to Rebind My Bank Account? Coinbase, one of the leading cryptocurrency exchanges, occasionally requires users to rebind their bank accounts. This process involves re-verifying and updating the connection between your Coinbase account and your bank account. The primary reasons for this requirement are to enhance security, com...
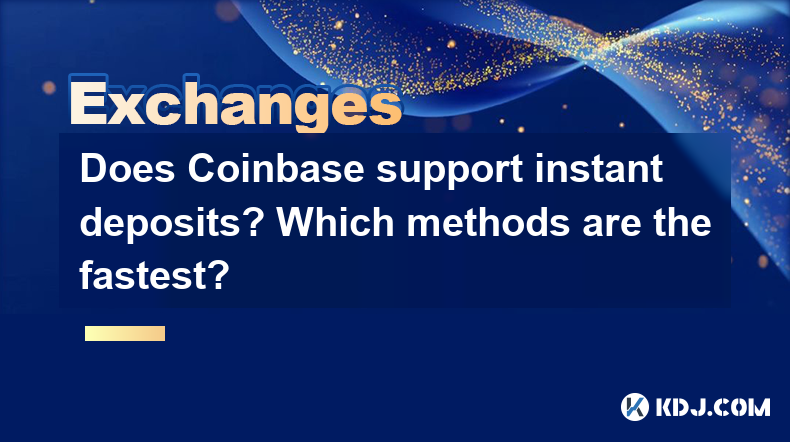
Does Coinbase support instant deposits? Which methods are the fastest?
Apr 28,2025 at 03:35pm
Coinbase, one of the leading cryptocurrency exchanges, offers various methods for users to deposit funds into their accounts. Many users are keen to understand whether Coinbase supports instant deposits and which methods are the fastest. This article will delve into these topics, providing a comprehensive overview of the deposit options available on Coi...
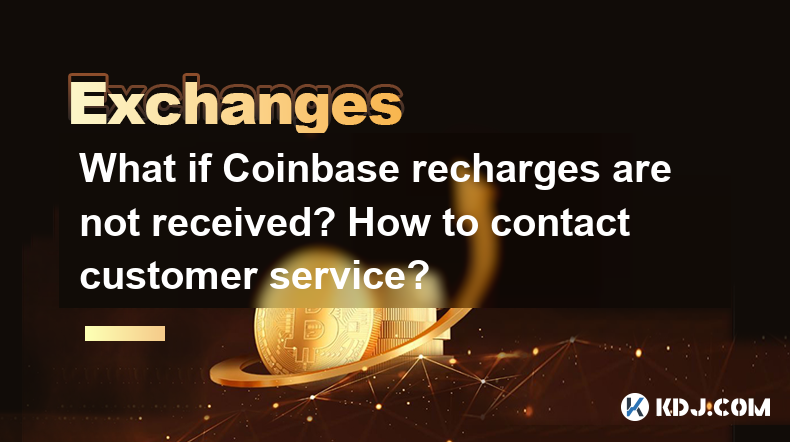
What if Coinbase recharges are not received? How to contact customer service?
Apr 28,2025 at 08:22am
When you send cryptocurrency to your Coinbase account and the recharge is not received, it can be a frustrating experience. This situation can arise due to various reasons, such as network congestion, incorrect address input, or delays in transaction processing. Understanding how to address this issue and contact Coinbase customer service effectively is...
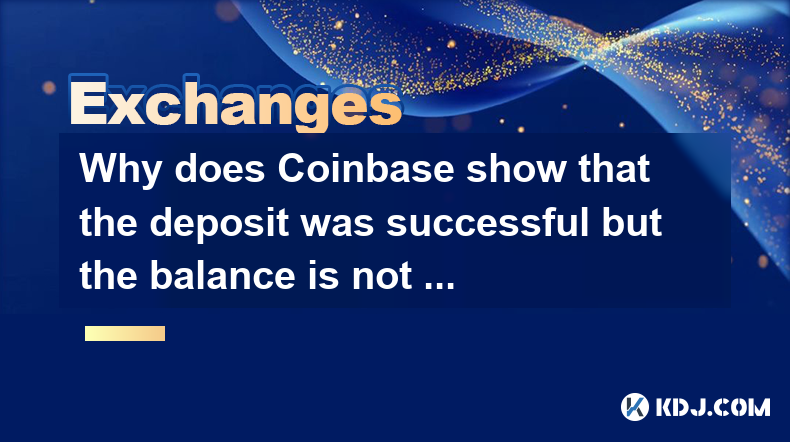
Why does Coinbase show that the deposit was successful but the balance is not updated?
Apr 28,2025 at 03:14pm
Introduction to the IssueWhen using Coinbase, one common issue users face is that their deposit appears to be successful, but their balance does not reflect the deposited amount. This discrepancy can be frustrating and confusing. Understanding why Coinbase shows a successful deposit but does not update the balance is crucial for users to manage their fu...
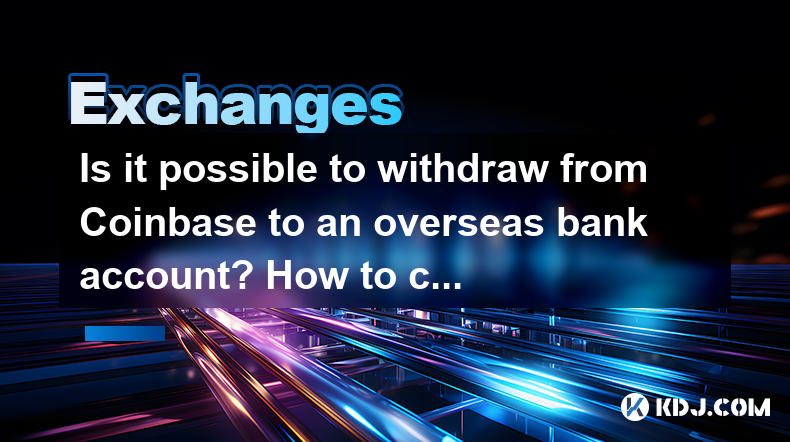
Is it possible to withdraw from Coinbase to an overseas bank account? How to calculate the exchange rate?
Apr 28,2025 at 09:14am
Introduction to Coinbase Withdrawals to Overseas Bank AccountsCoinbase is one of the leading cryptocurrency exchanges that allows users to buy, sell, and store various cryptocurrencies. One of the common queries users have is whether it's possible to withdraw funds from Coinbase directly to an overseas bank account. The answer is yes, it is possible, bu...
See all articles
