-
Bitcoin
$108,802.0448
0.59% -
Ethereum
$2,556.7655
1.66% -
Tether USDt
$1.0001
-0.02% -
XRP
$2.2765
2.15% -
BNB
$662.6901
1.16% -
Solana
$151.4936
2.68% -
USDC
$0.9999
0.00% -
TRON
$0.2857
0.49% -
Dogecoin
$0.1704
4.33% -
Cardano
$0.5847
1.63% -
Hyperliquid
$39.2227
-0.47% -
Sui
$2.9110
0.60% -
Bitcoin Cash
$491.8681
1.55% -
Chainlink
$13.4311
2.12% -
UNUS SED LEO
$9.0273
0.09% -
Avalanche
$18.1653
1.64% -
Stellar
$0.2442
2.69% -
Toncoin
$2.8966
5.36% -
Shiba Inu
$0.0...01180
2.95% -
Litecoin
$87.8955
1.49% -
Hedera
$0.1573
1.30% -
Monero
$316.6881
0.88% -
Polkadot
$3.3938
1.37% -
Dai
$0.9999
-0.01% -
Ethena USDe
$1.0001
-0.01% -
Bitget Token
$4.3976
0.08% -
Uniswap
$7.4020
6.83% -
Pepe
$0.0...01000
3.22% -
Aave
$276.6854
2.05% -
Pi
$0.4586
-0.62%
How to use algorithmic trading on Kraken?
Use Kraken's robust API to set up algorithmic trading, enhancing efficiency with Python and libraries like ccxt and pandas for optimal trading strategies.
Apr 27, 2025 at 06:56 am
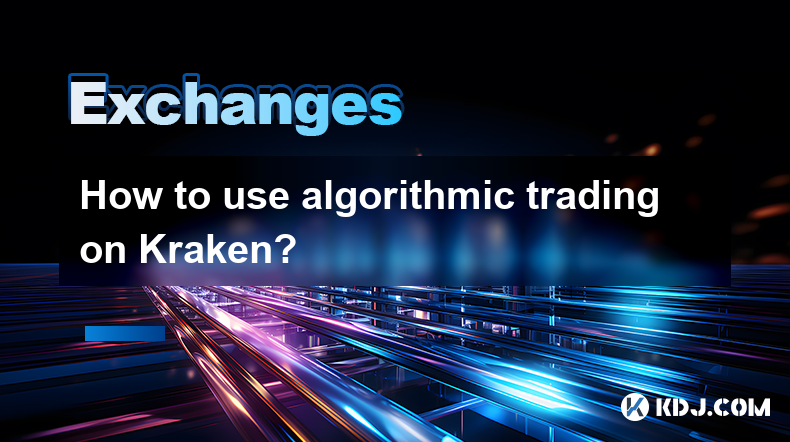
Algorithmic trading on Kraken can significantly enhance your trading efficiency and effectiveness. This method involves using computer programs to execute trades based on predefined criteria, such as timing, price, and volume. Kraken, one of the leading cryptocurrency exchanges, supports algorithmic trading through its robust API. In this article, we will guide you through the process of setting up and using algorithmic trading on Kraken, ensuring you can leverage this powerful tool to optimize your trading strategy.
Understanding Kraken's API
Before diving into algorithmic trading, it's essential to understand Kraken's API. The API, or Application Programming Interface, allows you to interact with Kraken's trading platform programmatically. Kraken offers a REST API for executing trades, retrieving account information, and querying market data, and a WebSocket API for real-time data streaming. Familiarizing yourself with these APIs is crucial for setting up algorithmic trading.
To access Kraken's API, you need to generate an API key from your Kraken account. Here's how you can do it:
- Log in to your Kraken account.
- Navigate to the 'Settings' section.
- Click on 'API' and then 'Generate New Key'.
- Set the permissions for your API key, ensuring you have the necessary permissions for trading and data retrieval.
- Confirm the key generation and securely store your API key and secret.
Setting Up Your Trading Environment
Once you have your API key, the next step is to set up your trading environment. You will need a programming language and a suitable development environment to write and run your trading algorithms. Python is a popular choice for algorithmic trading due to its ease of use and extensive libraries like ccxt and pandas.
To set up your Python environment:
- Install Python on your computer if you haven't already.
- Use a package manager like pip to install necessary libraries:
pip install ccxt
pip install pandas
pip install numpy
- Set up your IDE (Integrated Development Environment) such as PyCharm or VS Code.
With your environment set up, you can start writing your trading algorithms.
Writing Your First Trading Algorithm
Writing a trading algorithm involves defining the logic for when to buy and sell based on market conditions. Let's create a simple example using the ccxt library to interact with Kraken's API.
Here's a basic example of a trading algorithm that buys Bitcoin (BTC) when the price drops below a certain threshold and sells when it rises above another threshold:
import ccxt
import timeInitialize Kraken exchange
kraken = ccxt.kraken({
'apiKey': 'YOUR_API_KEY',
'secret': 'YOUR_SECRET_KEY',
})
Define trading parameters
buy_threshold = 20000 # Buy when BTC price drops below this
sell_threshold = 22000 # Sell when BTC price rises above this
while True:
try:
# Fetch the current BTC/USD price
ticker = kraken.fetch_ticker('BTC/USD')
current_price = ticker['last']
# Check if the current price meets our buy or sell criteria
if current_price < buy_threshold:
order = kraken.create_market_buy_order('BTC/USD', 0.01) # Buy 0.01 BTC
print(f"Bought BTC at {current_price}")
elif current_price > sell_threshold:
order = kraken.create_market_sell_order('BTC/USD', 0.01) # Sell 0.01 BTC
print(f"Sold BTC at {current_price}")
# Wait before checking the price again
time.sleep(60) # Check every minute
except Exception as e:
print(f"An error occurred: {e}")
time.sleep(60) # Wait before retrying
This script continuously checks the BTC/USD price and executes trades based on the defined thresholds. Make sure to replace 'YOUR_API_KEY'
and 'YOUR_SECRET_KEY'
with your actual Kraken API credentials.
Backtesting Your Algorithm
Before running your algorithm live, it's crucial to backtest it using historical data to ensure its effectiveness. Backtesting involves simulating how your algorithm would have performed in the past. You can use libraries like Backtrader or Zipline to backtest your algorithms.
Here's a simple example of backtesting using Backtrader:
import backtrader as bt
import ccxt
Initialize Kraken exchange
kraken = ccxt.kraken()
Fetch historical data
data = kraken.fetch_ohlcv('BTC/USD', '1d', limit=365) # Fetch 1-year daily data
Convert data to Backtrader format
data = bt.feeds.PandasData(dataname=pd.DataFrame(data, columns=['date', 'open', 'high', 'low', 'close', 'volume']))
class MyStrategy(bt.Strategy):
params = (
('buy_threshold', 20000),
('sell_threshold', 22000),
)
def __init__(self):
self.dataclose = self.datas[0].close
def next(self):
if self.dataclose[0] < self.p.buy_threshold:
self.buy(size=0.01)
elif self.dataclose[0] > self.p.sell_threshold:
self.sell(size=0.01)
Create a cerebro entity
cerebro = bt.Cerebro()
Add a strategy
cerebro.addstrategy(MyStrategy)
Add the data feed
cerebro.adddata(data)
Set our desired cash start
cerebro.broker.setcash(100000.0)
Add a FixedSize sizer according to the stake
cerebro.addsizer(bt.sizers.FixedSize, stake=0.01)
Set the commission
cerebro.broker.setcommission(commission=0.001)
Print out the starting conditions
print('Starting Portfolio Value: %.2f' % cerebro.broker.getvalue())
Run over everything
cerebro.run()
Print out the final result
print('Final Portfolio Value: %.2f' % cerebro.broker.getvalue())
This script fetches historical data from Kraken, sets up a Backtrader strategy based on the same buy and sell thresholds, and runs the backtest to see how the strategy would have performed over the past year.
Deploying Your Algorithm
Once you're satisfied with your backtesting results, you can deploy your algorithm to run live. You can run your algorithm directly on your local machine, or for more reliability, you can use a cloud service like Amazon Web Services (AWS) or Google Cloud Platform (GCP).
To deploy on a cloud service:
- Set up a cloud instance with Python installed.
- Upload your script and necessary libraries to the instance.
- Configure the instance to run your script continuously, possibly using a tool like screen or tmux to keep it running in the background.
Here's an example of how to set up a script to run continuously using screen
:
- Open a terminal and start a new screen session:
screen -S trading_bot
- Run your Python script inside the screen session:
python your_script.py
- Detach from the screen session by pressing
Ctrl+A
followed byD
. - Your script will continue running in the background.
Monitoring and Adjusting Your Algorithm
After deploying your algorithm, it's essential to monitor its performance and make adjustments as needed. You can use tools like Grafana or Kibana to set up dashboards that display real-time data and performance metrics.
To monitor your algorithm:
- Set up logging in your script to record all trades and important events.
- Use a tool like Grafana to create dashboards that visualize your trading data.
- Regularly review the performance of your algorithm and adjust the trading parameters as market conditions change.
Here's a basic example of how to add logging to your trading script:
import loggingSet up logging
logging.basicConfig(filename='trading_log.txt', level=logging.INFO, format='%(asctime)s - %(message)s')
Inside your trading loop
if current_price < buy_threshold:
order = kraken.create_market_buy_order('BTC/USD', 0.01)
logging.info(f"Bought BTC at {current_price}")
elif current_price > sell_threshold:
order = kraken.create_market_sell_order('BTC/USD', 0.01)
logging.info(f"Sold BTC at {current_price}")
This will log all buy and sell orders to a file named trading_log.txt
, which you can review to monitor your algorithm's performance.
Frequently Asked Questions
Q: Can I use Kraken's API for algorithmic trading on other platforms?
A: Kraken's API is specifically designed for use with Kraken's platform. While you can use the data and insights gained from Kraken to inform trading decisions on other platforms, the API itself is not compatible with other exchanges.
Q: Are there any limitations to the number of API requests I can make on Kraken?
A: Yes, Kraken has rate limits on API requests to prevent abuse. The limits vary depending on the type of request and your account tier. It's important to check Kraken's documentation for the most current limits and to implement rate limiting in your algorithms to avoid hitting these limits.
Q: How can I ensure the security of my API key when using algorithmic trading on Kraken?
A: To ensure the security of your API key, never share it with anyone, use it only on trusted devices, and consider using environment variables or a secure key management system to store and retrieve your keys. Additionally, regularly review and revoke keys that are no longer in use.
Q: Can I backtest my trading strategies using real-time data on Kraken?
A: No, backtesting requires historical data, not real-time data. Kraken provides historical data through its API, which you can use to backtest your strategies. Real-time data is used for live trading and monitoring, not for backtesting.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Litecoin Breakout Watch: What Traders Need to Know Now
- 2025-07-06 16:50:13
- Bitcoin, Solana, Ethereum: Decoding the Latest Buzz on the Blockchain
- 2025-07-06 16:50:13
- Widnes Resident's 50p Could Be Your Ticket to Easy Street: Rare Coin Mania!
- 2025-07-06 16:55:13
- Bitcoin, Solaris Presale, and Token Rewards: What's the Buzz?
- 2025-07-06 16:55:13
- Ethereum Under Pressure: Price Drop Amid Global Uncertainties
- 2025-07-06 17:00:13
- XRP, SEC Case, and Prosperity: A New Era for XRP Holders?
- 2025-07-06 17:10:13
Related knowledge
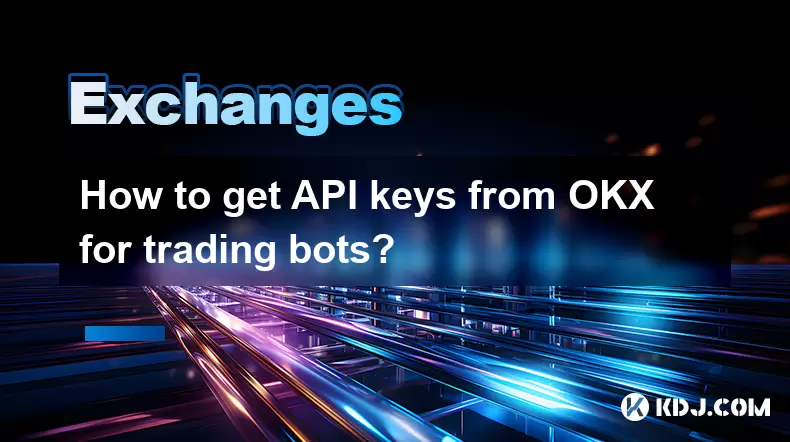
How to get API keys from OKX for trading bots?
Jul 03,2025 at 07:07am
Understanding API Keys on OKXTo interact with the OKX exchange programmatically, especially for building or running trading bots, you need to obtain an API key. An API (Application Programming Interface) key acts as a secure token that allows your bot to communicate with the exchange's servers. On OKX, these keys come with customizable permissions such ...
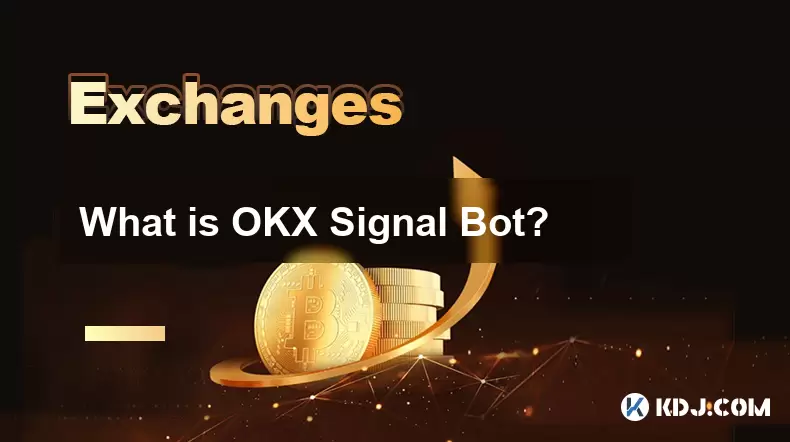
What is OKX Signal Bot?
Jul 02,2025 at 11:01pm
Understanding the Basics of OKX Signal BotThe OKX Signal Bot is a feature within the OKX ecosystem that provides users with automated trading signals and execution capabilities. Designed for both novice and experienced traders, this bot helps identify potential trading opportunities by analyzing market trends, technical indicators, and historical data. ...
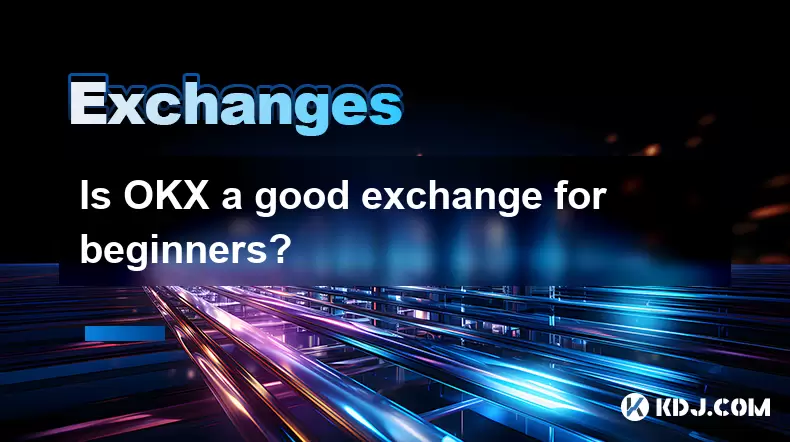
Is OKX a good exchange for beginners?
Jul 03,2025 at 05:00pm
What Is OKX and Why Is It Popular?OKX is one of the leading cryptocurrency exchanges globally, known for its robust trading infrastructure and a wide variety of digital assets available for trading. It supports over 300 cryptocurrencies, including major ones like Bitcoin (BTC), Ethereum (ETH), and Solana (SOL). The platform has gained popularity not onl...
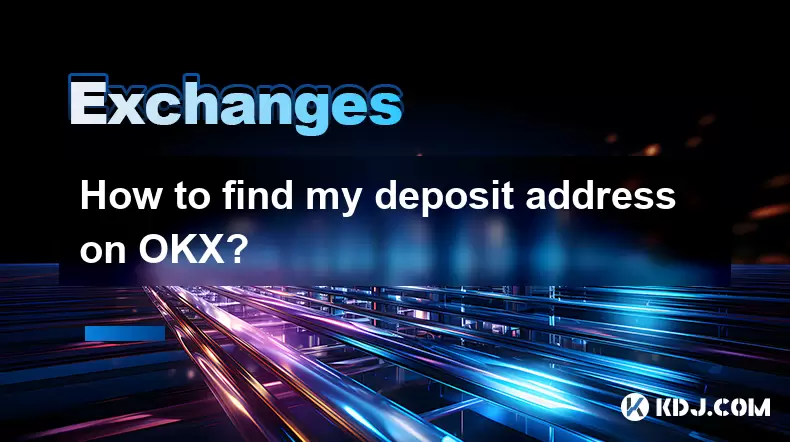
How to find my deposit address on OKX?
Jul 06,2025 at 02:28am
What is a Deposit Address on OKX?A deposit address on OKX is a unique alphanumeric identifier that allows users to receive cryptocurrencies into their OKX wallet. Each cryptocurrency has its own distinct deposit address, and using the correct one is crucial to ensure funds are received properly. If you're looking to transfer digital assets from another ...
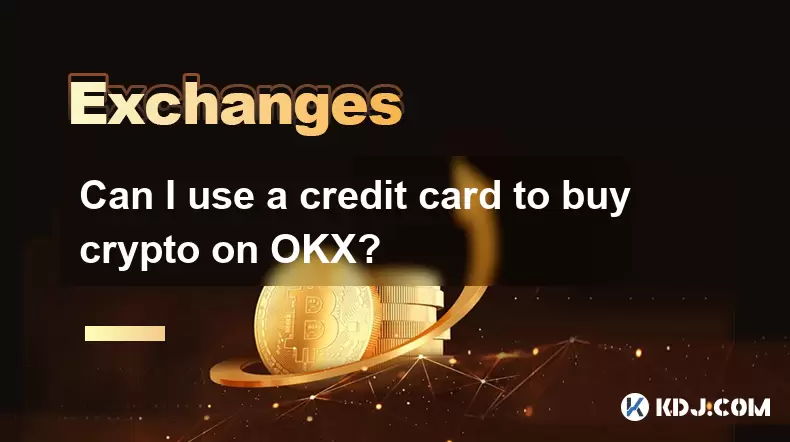
Can I use a credit card to buy crypto on OKX?
Jul 04,2025 at 04:28am
Understanding OKX and Credit Card PaymentsOKX is one of the leading cryptocurrency exchanges globally, offering a wide range of services including spot trading, derivatives, staking, and more. Users often wonder whether they can use a credit card to buy crypto on OKX, especially if they are new to the platform or looking for quick ways to enter the mark...
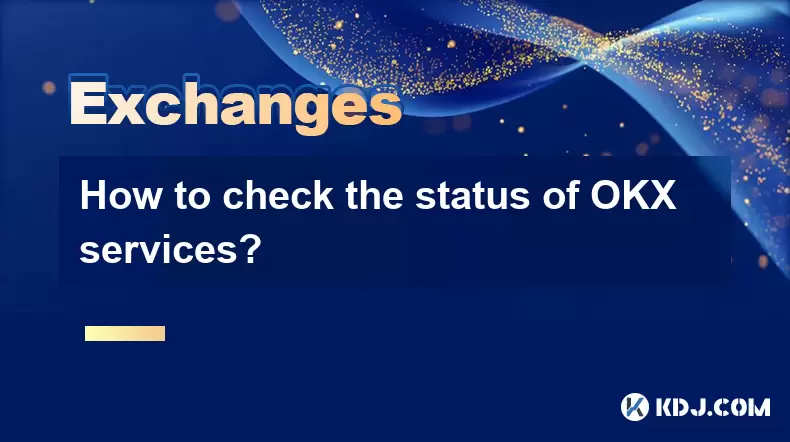
How to check the status of OKX services?
Jul 02,2025 at 11:14pm
What is OKX, and Why Checking Service Status Matters?OKX is one of the world’s leading cryptocurrency exchanges, offering services such as spot trading, futures trading, staking, and more. With millions of users relying on its platform for daily transactions, it's crucial to know how to check the status of OKX services. Downtime or maintenance can affec...
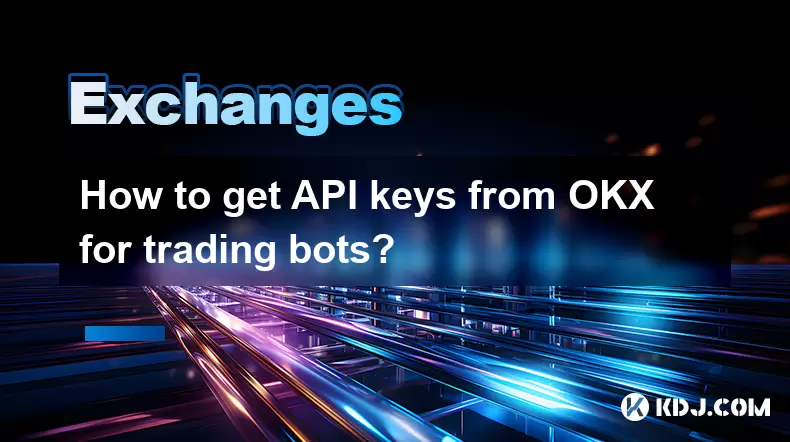
How to get API keys from OKX for trading bots?
Jul 03,2025 at 07:07am
Understanding API Keys on OKXTo interact with the OKX exchange programmatically, especially for building or running trading bots, you need to obtain an API key. An API (Application Programming Interface) key acts as a secure token that allows your bot to communicate with the exchange's servers. On OKX, these keys come with customizable permissions such ...
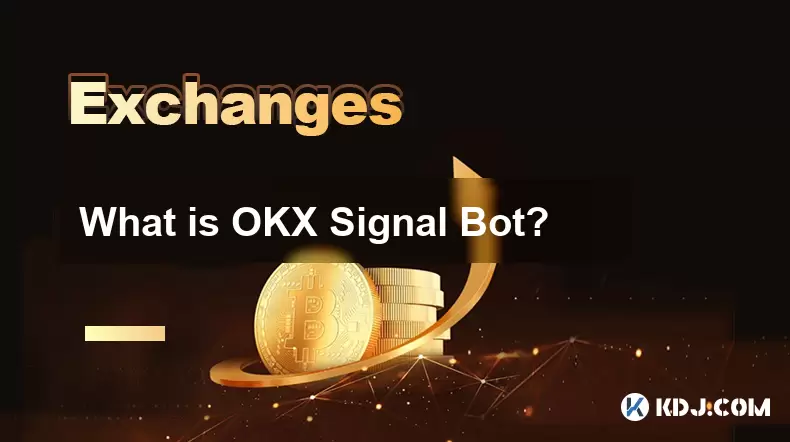
What is OKX Signal Bot?
Jul 02,2025 at 11:01pm
Understanding the Basics of OKX Signal BotThe OKX Signal Bot is a feature within the OKX ecosystem that provides users with automated trading signals and execution capabilities. Designed for both novice and experienced traders, this bot helps identify potential trading opportunities by analyzing market trends, technical indicators, and historical data. ...
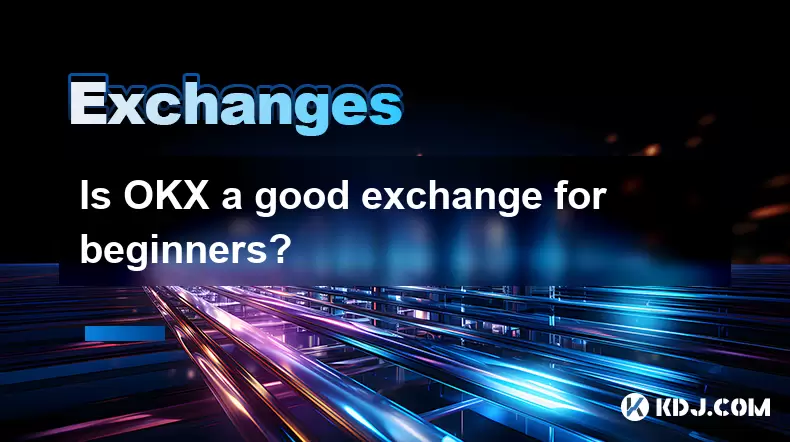
Is OKX a good exchange for beginners?
Jul 03,2025 at 05:00pm
What Is OKX and Why Is It Popular?OKX is one of the leading cryptocurrency exchanges globally, known for its robust trading infrastructure and a wide variety of digital assets available for trading. It supports over 300 cryptocurrencies, including major ones like Bitcoin (BTC), Ethereum (ETH), and Solana (SOL). The platform has gained popularity not onl...
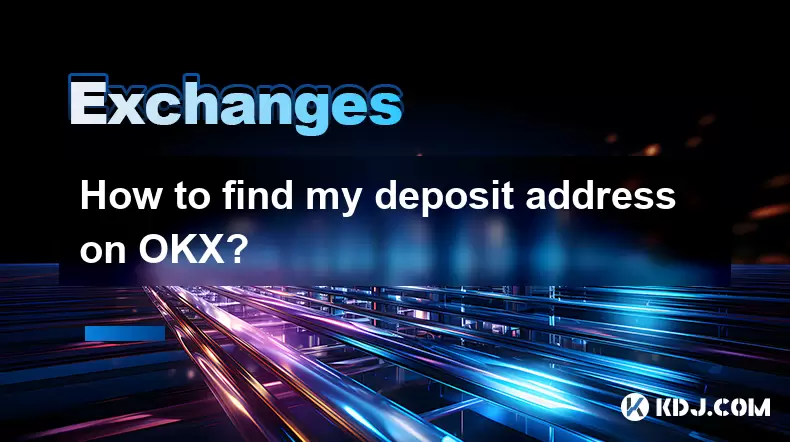
How to find my deposit address on OKX?
Jul 06,2025 at 02:28am
What is a Deposit Address on OKX?A deposit address on OKX is a unique alphanumeric identifier that allows users to receive cryptocurrencies into their OKX wallet. Each cryptocurrency has its own distinct deposit address, and using the correct one is crucial to ensure funds are received properly. If you're looking to transfer digital assets from another ...
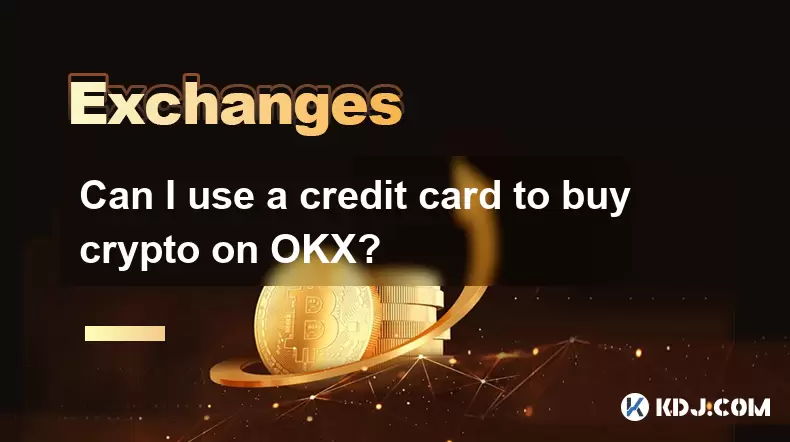
Can I use a credit card to buy crypto on OKX?
Jul 04,2025 at 04:28am
Understanding OKX and Credit Card PaymentsOKX is one of the leading cryptocurrency exchanges globally, offering a wide range of services including spot trading, derivatives, staking, and more. Users often wonder whether they can use a credit card to buy crypto on OKX, especially if they are new to the platform or looking for quick ways to enter the mark...
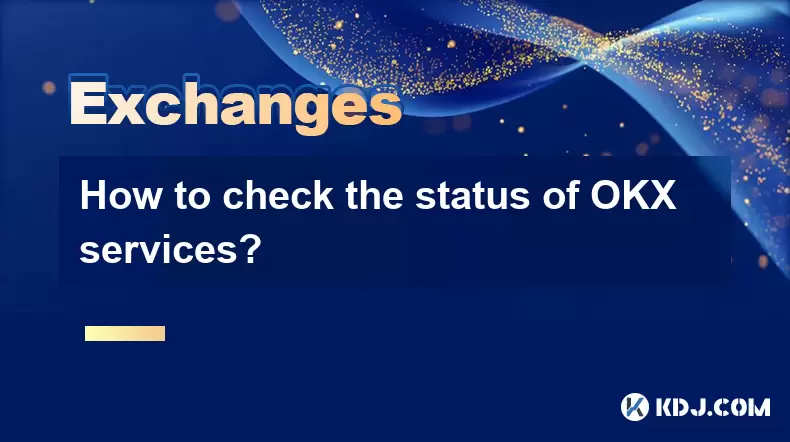
How to check the status of OKX services?
Jul 02,2025 at 11:14pm
What is OKX, and Why Checking Service Status Matters?OKX is one of the world’s leading cryptocurrency exchanges, offering services such as spot trading, futures trading, staking, and more. With millions of users relying on its platform for daily transactions, it's crucial to know how to check the status of OKX services. Downtime or maintenance can affec...
See all articles
