-
Bitcoin
$95,023.0224
1.68% -
Ethereum
$1,799.2469
1.59% -
Tether USDt
$1.0007
0.03% -
XRP
$2.1959
-1.25% -
BNB
$604.5938
0.81% -
Solana
$152.2406
1.16% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1819
0.78% -
Cardano
$0.7169
-2.37% -
TRON
$0.2436
-1.35% -
Sui
$3.6583
10.99% -
Chainlink
$15.1070
0.66% -
Avalanche
$22.4883
0.25% -
Stellar
$0.2855
2.44% -
UNUS SED LEO
$9.0263
-2.12% -
Hedera
$0.1974
5.32% -
Shiba Inu
$0.0...01395
2.70% -
Toncoin
$3.2074
0.53% -
Bitcoin Cash
$374.8391
6.31% -
Polkadot
$4.2932
2.96% -
Litecoin
$86.0567
2.94% -
Hyperliquid
$18.4569
1.13% -
Dai
$1.0000
0.00% -
Bitget Token
$4.4446
-0.16% -
Ethena USDe
$0.9997
0.03% -
Pi
$0.6487
-0.81% -
Monero
$228.5578
0.87% -
Pepe
$0.0...08829
1.26% -
Uniswap
$5.8867
0.11% -
Aptos
$5.5171
-0.01%
How to use Bitstamp's REST API?
Bitstamp's REST API enables programmatic trading, data retrieval, and account management on one of the oldest crypto exchanges.
Apr 25, 2025 at 01:57 pm
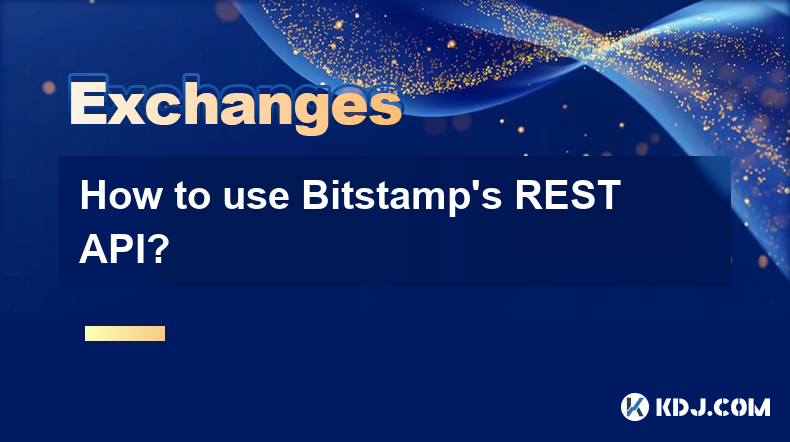
Using Bitstamp's REST API can be a powerful way to interact with one of the oldest and most reputable cryptocurrency exchanges. Whether you're looking to automate trading, fetch real-time data, or manage your account programmatically, understanding how to use Bitstamp's REST API is crucial. This guide will walk you through the process step-by-step, ensuring you have all the information you need to get started.
Understanding Bitstamp's REST API
Bitstamp's REST API is a set of endpoints that allow developers to interact with the Bitstamp exchange programmatically. It supports various operations such as trading, retrieving account information, and accessing market data. The API uses standard HTTP methods like GET, POST, and DELETE to perform these operations.
To use the API, you'll need to have a Bitstamp account and generate API keys. These keys will authenticate your requests and ensure that only you can access your account data.
Setting Up Your Bitstamp API Keys
Before you can use the Bitstamp REST API, you need to set up your API keys. Here's how to do it:
- Log in to your Bitstamp account and navigate to the "Account" section.
- Click on "API Access" from the dropdown menu.
- Click on "New API Key" to start the process of generating a new key.
- You will be prompted to enter a name for your API key. This helps you manage multiple keys if needed.
- You will also need to set permissions for the key. Choose the permissions that match your intended use of the API.
- After setting the permissions, click "Generate" to create the key.
- Save the API key and secret securely, as you will need them for all API requests.
Making Your First API Request
Once you have your API keys, you can start making requests to the Bitstamp API. Let's start with a simple GET request to retrieve the current ticker for Bitcoin (BTC/USD).
- Choose your programming language and set up an HTTP client. For this example, we'll use Python with the
requests
library. - Install the
requests
library if you haven't already, usingpip install requests
. - Write the code to make the API request:
import requestsReplace with your actual API key and secret
api_key = "your_api_key"
api_secret = "your_api_secret"
The endpoint for the ticker
url = "https://www.bitstamp.net/api/v2/ticker/btcusd"
Make the request
response = requests.get(url)
Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to retrieve data")
This code will fetch the current ticker data for BTC/USD and print it to the console.
Authenticating API Requests
For operations that require authentication, such as placing orders or retrieving your account balance, you need to sign your requests with your API key and secret. Here's how to do it:
- Generate a nonce, which is a unique number for each request. This prevents replay attacks.
- Create a signature using the nonce, the API key, and the API secret.
- Include the signature in the request headers.
Here's an example of how to authenticate a request to retrieve your account balance:
import requests
import hmac
import time
import hashlib
api_key = "your_api_key"
api_secret = "your_api_secret"
Generate a nonce
nonce = str(int(time.time() * 1000))
Create the message to sign
message = nonce + api_key + api_secret
Generate the signature
signature = hmac.new(
api_secret.encode('utf-8'),
msg=message.encode('utf-8'),
digestmod=hashlib.sha256
).hexdigest().upper()
Set the headers
headers = {
"X-Auth": "BITSTAMP " + api_key,
"X-Auth-Signature": signature,
"X-Auth-Nonce": nonce,
"X-Auth-Timestamp": str(int(time.time())),
"Content-Type": "application/x-www-form-urlencoded"
}
The endpoint for the balance
url = "https://www.bitstamp.net/api/v2/balance/"
Make the request
response = requests.get(url, headers=headers)
Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to retrieve data")
This code will fetch your account balance and print it to the console.
Placing Orders with the API
To place orders using the Bitstamp REST API, you'll need to use the appropriate endpoints and include the necessary parameters. Here's how to place a market order to buy Bitcoin:
- Prepare the parameters for the order. For a market order, you'll need to specify the amount of Bitcoin you want to buy.
- Sign the request as described in the previous section.
- Send the POST request to the appropriate endpoint.
Here's an example of how to place a market order:
import requests
import hmac
import time
import hashlib
api_key = "your_api_key"
api_secret = "your_api_secret"
Generate a nonce
nonce = str(int(time.time() * 1000))
Prepare the order parameters
amount = "0.01" # Amount of BTC to buy
Create the message to sign
message = nonce + api_key + api_secret
Generate the signature
signature = hmac.new(
api_secret.encode('utf-8'),
msg=message.encode('utf-8'),
digestmod=hashlib.sha256
).hexdigest().upper()
Set the headers
headers = {
"X-Auth": "BITSTAMP " + api_key,
"X-Auth-Signature": signature,
"X-Auth-Nonce": nonce,
"X-Auth-Timestamp": str(int(time.time())),
"Content-Type": "application/x-www-form-urlencoded"
}
The endpoint for placing a market order
url = "https://www.bitstamp.net/api/v2/buy/market/btcusd/"
Prepare the data to send
data = {
"amount": amount
}
Make the request
response = requests.post(url, headers=headers, data=data)
Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to place order")
This code will place a market order to buy 0.01 BTC and print the response to the console.
Handling Errors and Rate Limits
When using the Bitstamp REST API, it's important to handle errors and respect rate limits to ensure smooth operation. Here are some tips:
- Check the status code of each response. A status code of 200 indicates success, while other codes indicate errors.
- Read the error messages provided in the response body. They can give you more information about what went wrong.
- Respect the rate limits. Bitstamp has rate limits in place to prevent abuse. If you exceed these limits, your requests may be blocked.
Here's an example of how to handle errors:
import requests
Make the request
response = requests.get("https://www.bitstamp.net/api/v2/ticker/btcusd")
Check if the request was successful
if response.status_code == 200:
data = response.json()
print(data)
else:
print("Failed to retrieve data. Status code:", response.status_code)
print("Error message:", response.text)
This code will print the status code and error message if the request fails.
Frequently Asked Questions
Q: Can I use Bitstamp's REST API for automated trading?
Yes, you can use Bitstamp's REST API for automated trading. By placing orders programmatically, you can implement trading strategies that execute automatically based on market conditions.
Q: Is there a limit to the number of API requests I can make?
Yes, Bitstamp has rate limits in place to prevent abuse. The specific limits depend on your account type and the type of request you're making. You should check Bitstamp's documentation for the most current information on rate limits.
Q: How secure is it to use the Bitstamp REST API?
Using the Bitstamp REST API can be secure if you follow best practices. Always keep your API keys and secrets secure, use HTTPS for all requests, and implement proper error handling and logging. Additionally, Bitstamp uses encryption and other security measures to protect your data.
Q: Can I use the Bitstamp REST API to manage multiple accounts?
Yes, you can use the Bitstamp REST API to manage multiple accounts by generating separate API keys for each account. This allows you to keep your operations organized and secure.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- XYO Network Rallies Over 50% Ahead of Bithumb Listing, Targeting New Market Segments
- 2025-04-26 01:00:13
- On April 25, 2025, the crypto market witnessed significant whale movement around the SUNDOG token
- 2025-04-26 01:00:13
- Initia Launches Mainnet with 1 Billion INIT Supply and Community-First Airdrop
- 2025-04-26 00:55:13
- Crypto Lost Its Moral Compass. We Must Return It to Its Decentralized Roots
- 2025-04-26 00:55:13
- Surf the Surge: Arctic Pablo Presale Explodes at Frostbite City – Best Crypto Coin with 100x Potential Alongside Brett and Fartcoin Updates
- 2025-04-26 00:50:13
- Fantasy Pepe (FEPE) Aims to Break New Ground in the Meme Coin Market by Combining Football and AI
- 2025-04-26 00:50:13
Related knowledge
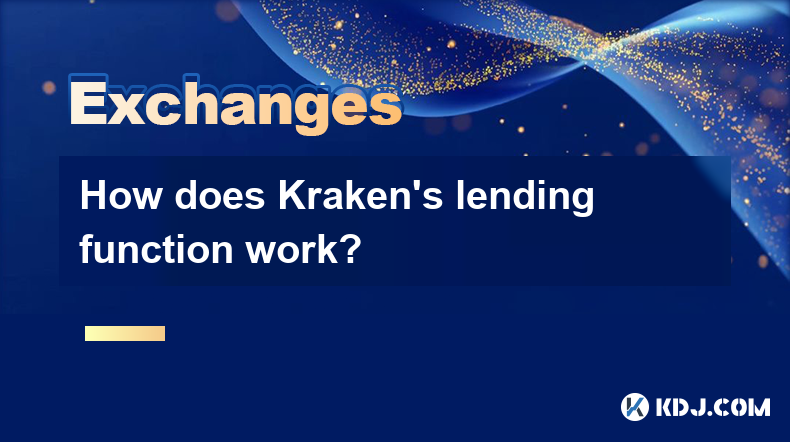
How does Kraken's lending function work?
Apr 25,2025 at 07:28pm
Kraken's lending function provides users with the opportunity to earn interest on their cryptocurrency holdings by lending them out to other users on the platform. This feature is designed to be user-friendly and secure, allowing both novice and experienced crypto enthusiasts to participate in the lending market. In this article, we will explore how Kra...
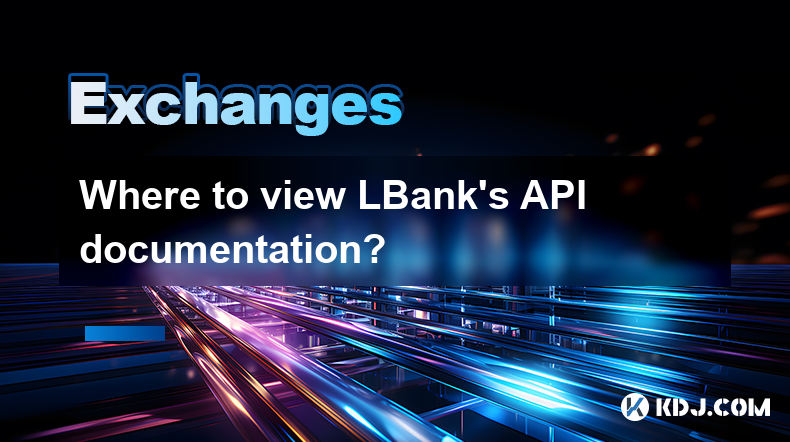
Where to view LBank's API documentation?
Apr 24,2025 at 06:21am
LBank is a popular cryptocurrency exchange that provides various services to its users, including trading, staking, and more. One of the essential resources for developers and advanced users is the API documentation, which allows them to interact with the platform programmatically. In this article, we will explore where to view LBank's API documentation...
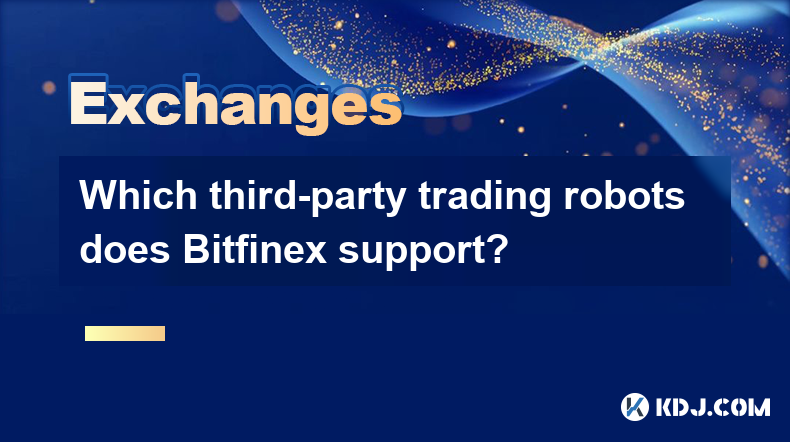
Which third-party trading robots does Bitfinex support?
Apr 24,2025 at 03:08am
Bitfinex, one of the leading cryptocurrency exchanges, supports a variety of third-party trading robots to enhance the trading experience of its users. These robots automate trading strategies, allowing traders to execute trades more efficiently and potentially increase their profits. In this article, we will explore the different third-party trading ro...
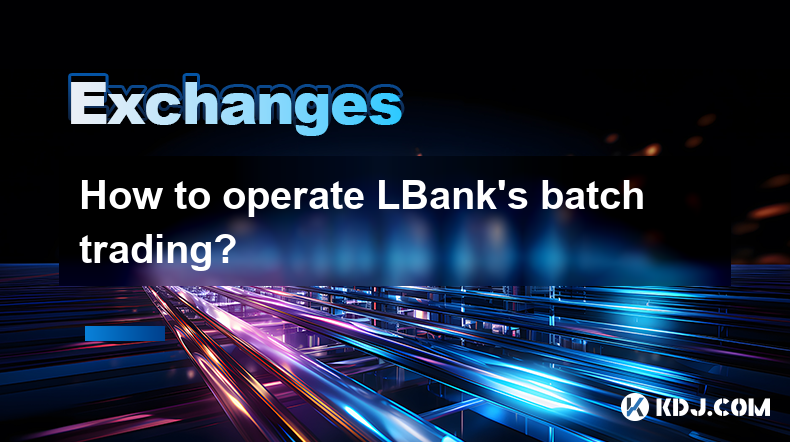
How to operate LBank's batch trading?
Apr 23,2025 at 01:15pm
LBank is a well-known cryptocurrency exchange that offers a variety of trading features to its users, including the option for batch trading. Batch trading allows users to execute multiple trades simultaneously, which can be particularly useful for those looking to manage a diverse portfolio or engage in arbitrage opportunities. In this article, we will...
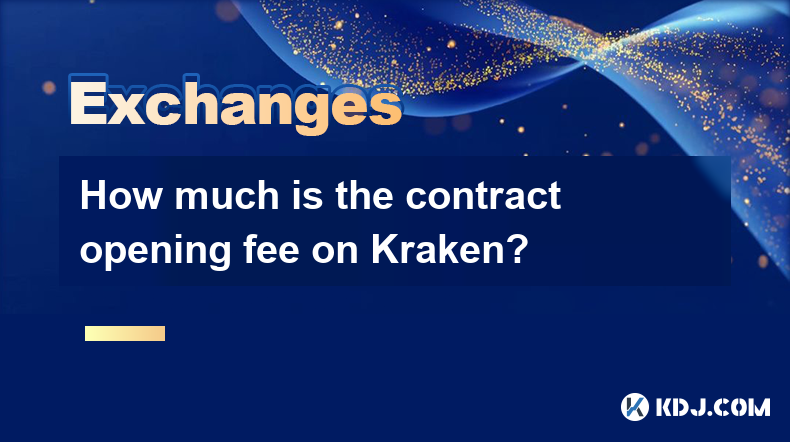
How much is the contract opening fee on Kraken?
Apr 23,2025 at 03:00pm
When engaging with cryptocurrency exchanges like Kraken, understanding the fee structure is crucial for managing trading costs effectively. One specific fee that traders often inquire about is the contract opening fee. On Kraken, this fee is associated with futures trading, which allows users to speculate on the future price of cryptocurrencies. Let's d...
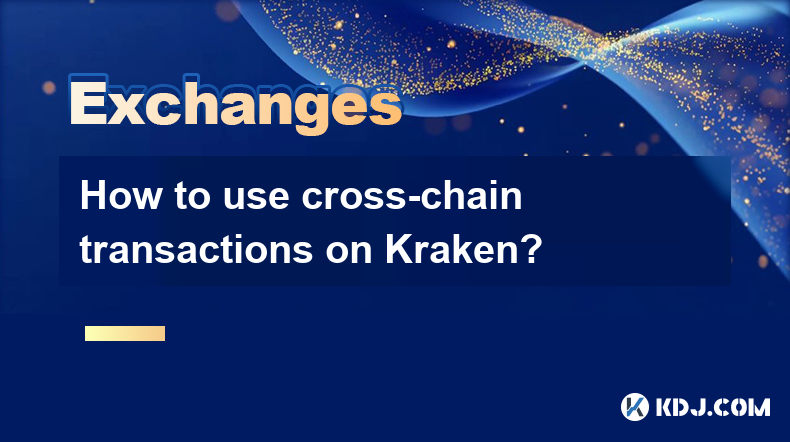
How to use cross-chain transactions on Kraken?
Apr 23,2025 at 12:50pm
Cross-chain transactions on Kraken allow users to transfer cryptocurrencies between different blockchain networks seamlessly. This feature is particularly useful for traders and investors looking to diversify their portfolios across various blockchains or to take advantage of specific opportunities on different networks. In this article, we will explore...
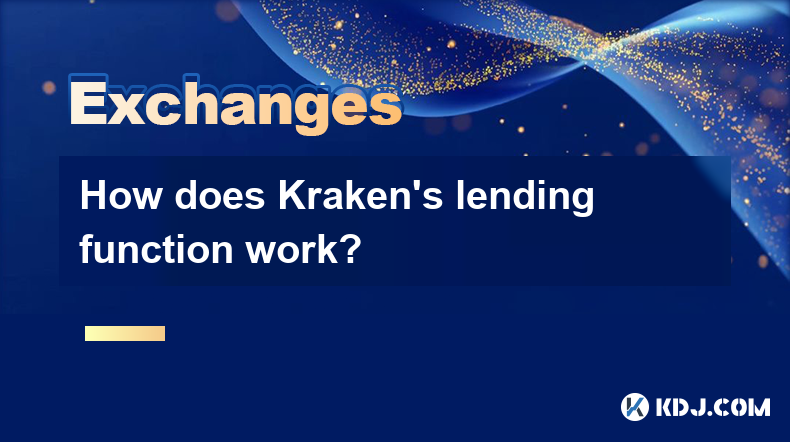
How does Kraken's lending function work?
Apr 25,2025 at 07:28pm
Kraken's lending function provides users with the opportunity to earn interest on their cryptocurrency holdings by lending them out to other users on the platform. This feature is designed to be user-friendly and secure, allowing both novice and experienced crypto enthusiasts to participate in the lending market. In this article, we will explore how Kra...
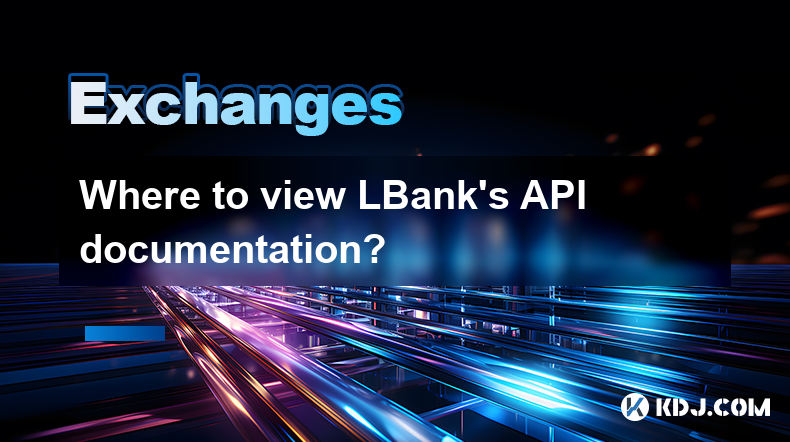
Where to view LBank's API documentation?
Apr 24,2025 at 06:21am
LBank is a popular cryptocurrency exchange that provides various services to its users, including trading, staking, and more. One of the essential resources for developers and advanced users is the API documentation, which allows them to interact with the platform programmatically. In this article, we will explore where to view LBank's API documentation...
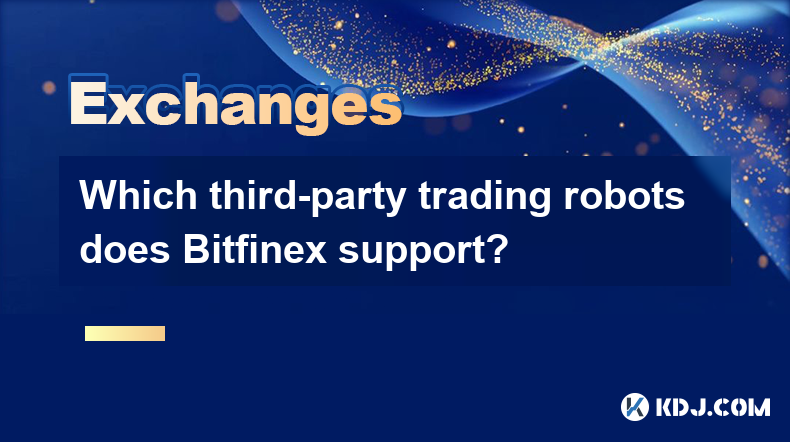
Which third-party trading robots does Bitfinex support?
Apr 24,2025 at 03:08am
Bitfinex, one of the leading cryptocurrency exchanges, supports a variety of third-party trading robots to enhance the trading experience of its users. These robots automate trading strategies, allowing traders to execute trades more efficiently and potentially increase their profits. In this article, we will explore the different third-party trading ro...
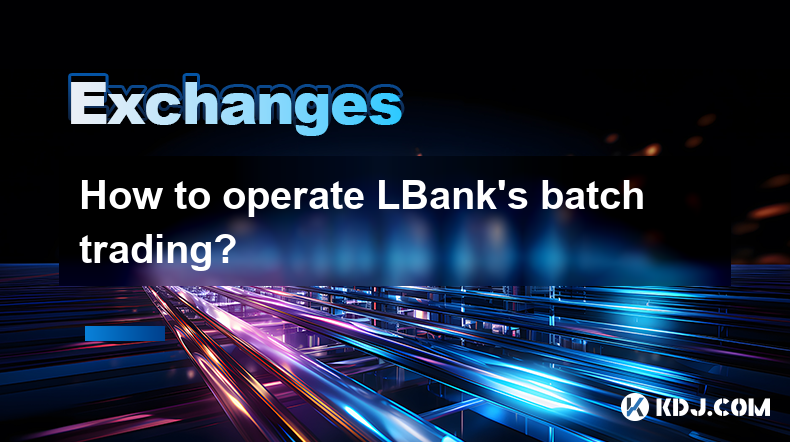
How to operate LBank's batch trading?
Apr 23,2025 at 01:15pm
LBank is a well-known cryptocurrency exchange that offers a variety of trading features to its users, including the option for batch trading. Batch trading allows users to execute multiple trades simultaneously, which can be particularly useful for those looking to manage a diverse portfolio or engage in arbitrage opportunities. In this article, we will...
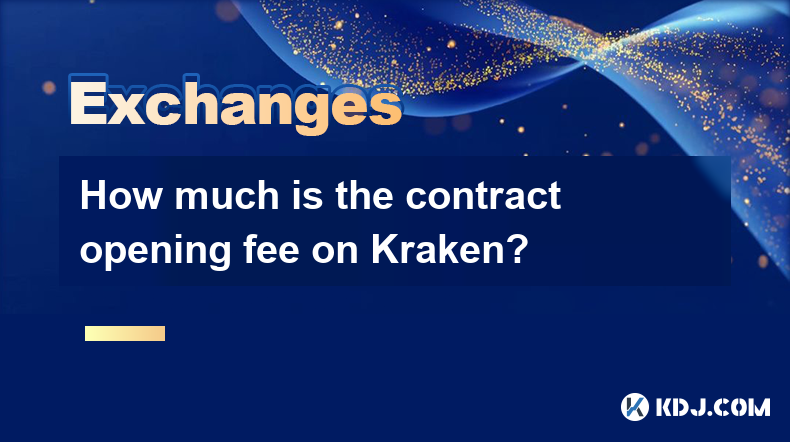
How much is the contract opening fee on Kraken?
Apr 23,2025 at 03:00pm
When engaging with cryptocurrency exchanges like Kraken, understanding the fee structure is crucial for managing trading costs effectively. One specific fee that traders often inquire about is the contract opening fee. On Kraken, this fee is associated with futures trading, which allows users to speculate on the future price of cryptocurrencies. Let's d...
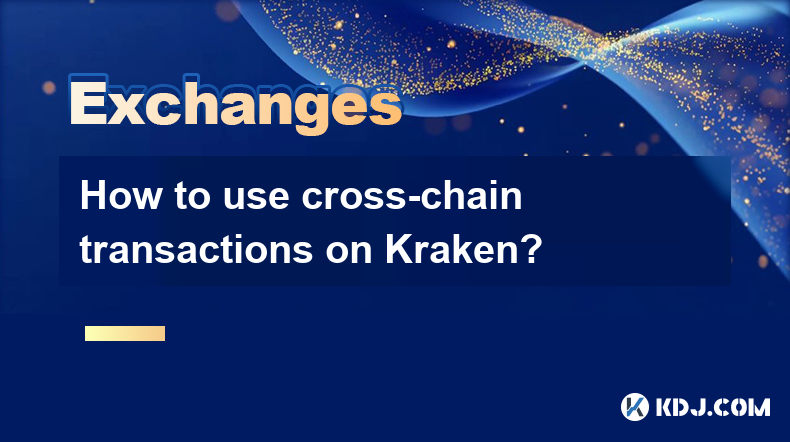
How to use cross-chain transactions on Kraken?
Apr 23,2025 at 12:50pm
Cross-chain transactions on Kraken allow users to transfer cryptocurrencies between different blockchain networks seamlessly. This feature is particularly useful for traders and investors looking to diversify their portfolios across various blockchains or to take advantage of specific opportunities on different networks. In this article, we will explore...
See all articles
