-
Bitcoin
$108,262.4325
-1.40% -
Ethereum
$2,518.2882
-2.94% -
Tether USDt
$1.0003
-0.01% -
XRP
$2.2262
-1.71% -
BNB
$653.9254
-1.55% -
Solana
$148.1036
-3.11% -
USDC
$1.0000
0.01% -
TRON
$0.2829
-1.45% -
Dogecoin
$0.1639
-4.82% -
Cardano
$0.5742
-4.43% -
Hyperliquid
$38.9506
-3.95% -
Sui
$2.9040
-4.34% -
Bitcoin Cash
$484.8307
-2.62% -
Chainlink
$13.1971
-3.73% -
UNUS SED LEO
$9.0822
0.51% -
Avalanche
$17.8613
-4.01% -
Stellar
$0.2385
-2.26% -
Toncoin
$2.7570
-3.88% -
Shiba Inu
$0.0...01145
-3.99% -
Litecoin
$86.9999
-2.43% -
Hedera
$0.1538
-3.90% -
Monero
$313.7554
-2.03% -
Polkadot
$3.3681
-5.08% -
Dai
$1.0000
0.00% -
Ethena USDe
$1.0001
-0.01% -
Bitget Token
$4.4401
-2.97% -
Uniswap
$6.9644
-8.41% -
Pepe
$0.0...09666
-4.79% -
Aave
$266.5686
-5.04% -
Pi
$0.4713
-4.95%
How to use the Maker (MKR) trading API? Does it support automated strategies?
The Maker trading API empowers traders to automate strategies, offering real-time data access and trade execution on the decentralized lending platform.
May 01, 2025 at 08:28 am
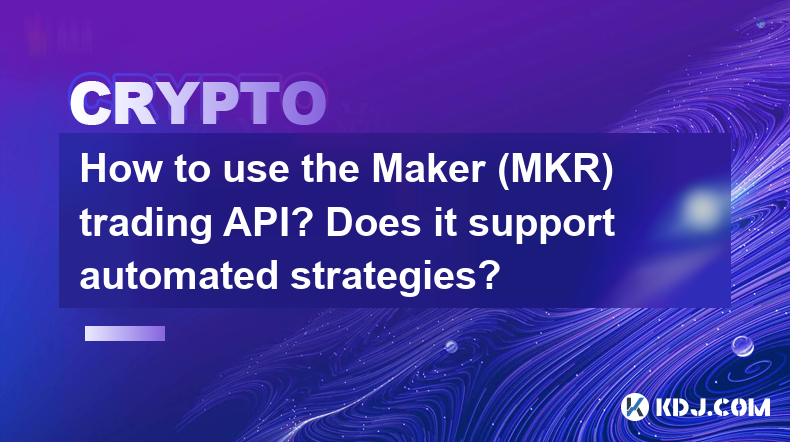
Using the Maker (MKR) trading API can be a powerful tool for traders looking to automate their trading strategies. The Maker platform, known for its decentralized lending and borrowing system, also provides an API that allows users to interact with the platform programmatically. In this article, we will explore how to use the Maker trading API and whether it supports automated trading strategies.
Understanding the Maker Trading API
The Maker trading API is designed to allow developers and traders to interact with the Maker protocol directly. This API enables users to access real-time data, execute trades, manage positions, and more. The API is typically used by those who want to build custom applications or automate their trading activities on the Maker platform.
To start using the Maker trading API, you first need to obtain an API key. This key serves as your authentication token, allowing you to make requests to the Maker server. Obtaining an API key involves creating an account on the Maker platform, navigating to the API section, and generating a new key. Once you have your key, you can begin making API calls.
Setting Up the API Environment
Before you can start making API calls, you need to set up your development environment. This involves choosing a programming language and setting up the necessary libraries. Popular choices for interacting with the Maker API include Python and JavaScript, both of which have well-maintained libraries for blockchain interactions.
- Install the necessary libraries: For Python, you might use
web3.py
oreth-brownie
. For JavaScript,ethers.js
orweb3.js
are common choices. - Set up your development environment: Ensure you have a code editor, a terminal, and any other tools you typically use for development.
- Configure your API key: Store your API key securely, preferably as an environment variable to avoid hardcoding it into your scripts.
Making API Calls
Once your environment is set up, you can start making API calls to the Maker platform. API calls can be used to fetch data, execute trades, or manage positions. Here's a step-by-step guide on how to make a simple API call using Python and web3.py
:
Import the necessary libraries:
from web3 import Web3
import jsonConnect to the Ethereum network:
w3 = Web3(Web3.HTTPProvider('https://mainnet.infura.io/v3/YOUR_PROJECT_ID'))
Load your private key and account:
with open('private_key.txt', 'r') as file:
private_key = file.read().strip()
account = w3.eth.account.from_key(private_key)
Prepare the transaction:
contract_address = '0x9f8F72aA9304c8B593d555F12eF6589cC3A579A2' # Example contract address
abi = json.loads('ABI_JSON_STRING') # Load the ABI for the contract
contract = w3.eth.contract(address=contract_address, abi=abi)Execute the transaction:
nonce = w3.eth.get_transaction_count(account.address)
transaction = contract.functions.someFunction().buildTransaction({'from': account.address, 'nonce': nonce, 'gasPrice': w3.toWei('20', 'gwei'), 'gas': 200000,
})
signed_txn = w3.eth.account.sign_transaction(transaction, private_key)
tx_hash = w3.eth.send_raw_transaction(signed_txn.rawTransaction)
This example demonstrates how to interact with a smart contract on the Maker platform. Each API call will have different parameters and requirements, so be sure to refer to the Maker API documentation for specific details.
Automating Trading Strategies with the Maker API
The Maker trading API does support automated trading strategies. By leveraging the API, traders can create scripts that automatically execute trades based on predefined conditions. This can be particularly useful for implementing strategies that require constant monitoring and quick execution, such as arbitrage or algorithmic trading.
To automate trading strategies, you'll need to write a script that continuously monitors market conditions and executes trades when certain criteria are met. Here's a basic example of how you might automate a simple trading strategy using Python:
Set up your script to monitor market conditions:
import time
while True:
# Fetch current market data using the Maker API current_price = fetch_current_price() # Define your trading criteria if current_price < target_price: # Execute a buy order execute_buy_order() elif current_price > sell_price: # Execute a sell order execute_sell_order() # Wait for a short period before checking again time.sleep(60) # Check every minute
Implement the
fetch_current_price
function:def fetch_current_price(): # Use the Maker API to fetch the current price of MKR # This is a placeholder; you'll need to implement the actual API call return 1000 # Example price
Implement the
execute_buy_order
andexecute_sell_order
functions:def execute_buy_order(): # Use the Maker API to execute a buy order # This is a placeholder; you'll need to implement the actual API call print("Executing buy order")
def execute_sell_order():
# Use the Maker API to execute a sell order # This is a placeholder; you'll need to implement the actual API call print("Executing sell order")
This example demonstrates how you might structure an automated trading strategy. The actual implementation will depend on your specific strategy and the Maker API endpoints you use.
Handling Errors and Security
When using the Maker trading API, it's important to handle errors and ensure the security of your operations. Error handling involves catching and responding to API errors, which can occur due to network issues, invalid parameters, or other reasons. Here's how you might handle errors in your Python script:
Wrap your API calls in try-except blocks:
try: # API call here response = api_call()
except Exception as e:
print(f"An error occurred: {e}") # Implement error recovery logic here
Implement retries for transient errors:
import time
max_retries = 3
for attempt in range(max_retries):try: # API call here response = api_call() break except Exception as e: if attempt < max_retries - 1: time.sleep(2 ** attempt) # Exponential backoff else: raise e # Re-raise the exception if all retries fail
Security is also crucial when using the Maker trading API. Ensure that your API key is stored securely and never shared. Use HTTPS for all API communications, and consider implementing additional security measures such as rate limiting and IP whitelisting.
Testing and Deployment
Before deploying your automated trading strategy to the live Maker platform, it's essential to test your script thoroughly. You can use testnets or simulated environments to ensure your strategy works as expected without risking real funds.
- Test on a testnet: Use a testnet like Goerli to simulate real-world conditions without using real MKR.
- Simulate market conditions: Create a simulation environment to test how your strategy performs under various market scenarios.
- Monitor and log: Implement logging to track the performance of your strategy and identify any issues.
Once you're confident in your strategy, you can deploy it to the live Maker platform. Ensure you have robust monitoring and alerting in place to quickly respond to any issues that arise.
Frequently Asked Questions
Q: Can I use the Maker trading API with other cryptocurrencies besides MKR?
A: The Maker trading API is primarily designed for interacting with the Maker protocol, which is focused on MKR and DAI. However, you can use the Ethereum network's capabilities to interact with other cryptocurrencies if you're using a compatible wallet or exchange that supports them.
Q: Are there any limitations on the number of API calls I can make per day?
A: The Maker API may have rate limits in place to prevent abuse. These limits can vary, so it's important to check the Maker API documentation for the most current information on rate limits and how to handle them.
Q: How do I ensure my automated trading strategy complies with regulatory requirements?
A: Compliance with regulatory requirements depends on your jurisdiction and the specifics of your trading activities. It's important to consult with a legal professional to ensure your automated trading strategy adheres to all relevant laws and regulations.
Q: Can I use the Maker trading API to manage my DAI savings and borrowing positions?
A: Yes, the Maker trading API can be used to manage DAI savings and borrowing positions. You can interact with the Maker protocol to deposit collateral, borrow DAI, or manage your savings rate through API calls.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Bitcoin's Pattern Break: Are HODLers the Key to the Next Surge?
- 2025-07-04 18:50:12
- Bitcoin Price, Trump's Bill, and the $150K Dream: A NYC Take
- 2025-07-04 19:50:12
- Ethereum, LILPEPE, and the July Bounce: Will Pepe Steal ETH's Thunder?
- 2025-07-04 19:10:12
- Binance Institutional Loans: Unlocking 4x Leverage and Zero Interest for Whales
- 2025-07-04 19:15:12
- Bitcoin Bull Run: Analysts Eye Peak in Late 2025?
- 2025-07-04 19:20:13
- Pepe Indicators, Bullish Forecast: Can the Meme Coin Rally?
- 2025-07-04 19:25:12
Related knowledge
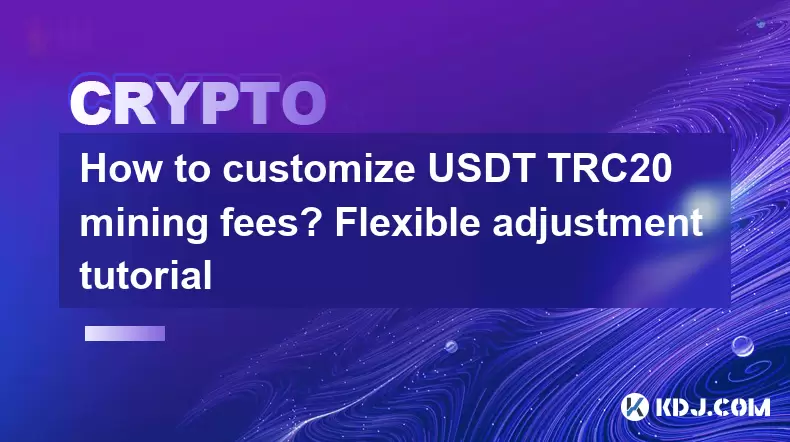
How to customize USDT TRC20 mining fees? Flexible adjustment tutorial
Jun 13,2025 at 01:42am
Understanding USDT TRC20 Mining FeesMining fees on the TRON (TRC20) network are essential for processing transactions. Unlike Bitcoin or Ethereum, where miners directly validate transactions, TRON uses a delegated proof-of-stake (DPoS) mechanism. However, users still need to pay bandwidth and energy fees, which are collectively referred to as 'mining fe...
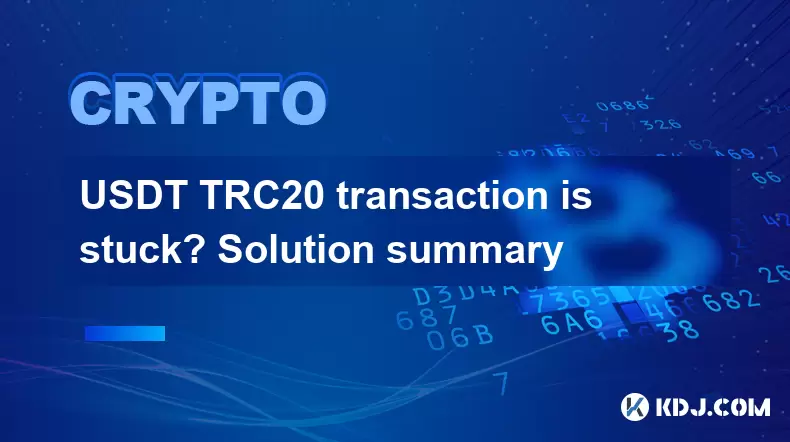
USDT TRC20 transaction is stuck? Solution summary
Jun 14,2025 at 11:15pm
Understanding USDT TRC20 TransactionsWhen users mention that a USDT TRC20 transaction is stuck, they typically refer to a situation where the transfer of Tether (USDT) on the TRON blockchain has not been confirmed for an extended period. This issue may arise due to various reasons such as network congestion, insufficient transaction fees, or wallet-rela...
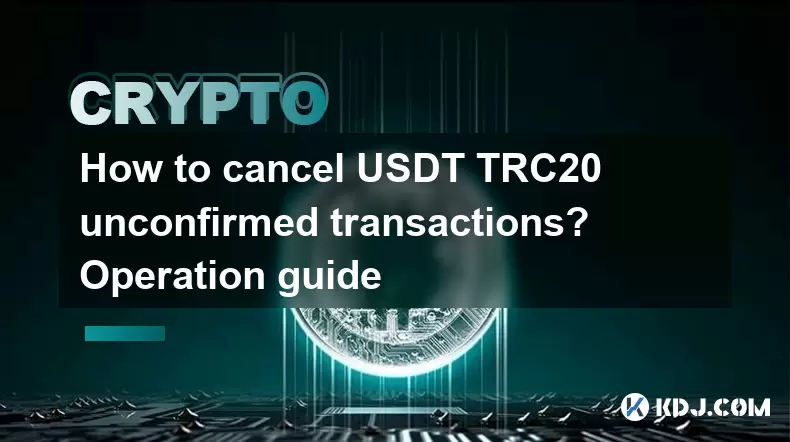
How to cancel USDT TRC20 unconfirmed transactions? Operation guide
Jun 13,2025 at 11:01pm
Understanding USDT TRC20 Unconfirmed TransactionsWhen dealing with USDT TRC20 transactions, it’s crucial to understand what an unconfirmed transaction means. An unconfirmed transaction is one that has been broadcasted to the blockchain network but hasn’t yet been included in a block. This typically occurs due to low transaction fees or network congestio...
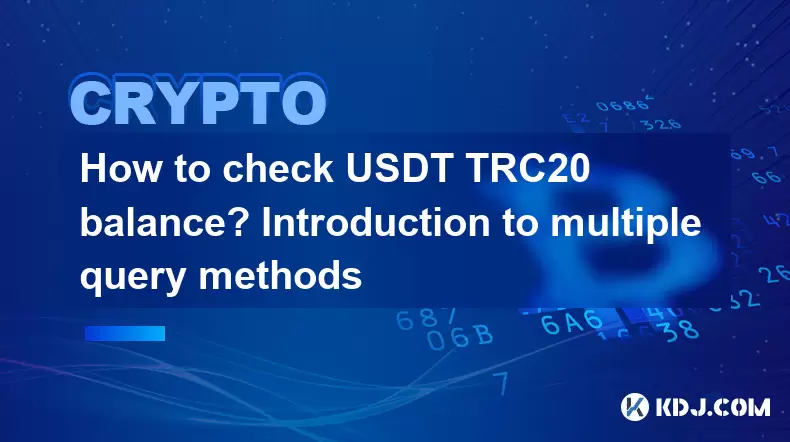
How to check USDT TRC20 balance? Introduction to multiple query methods
Jun 21,2025 at 02:42am
Understanding USDT TRC20 and Its ImportanceUSDT (Tether) is one of the most widely used stablecoins in the cryptocurrency market. It exists on multiple blockchain networks, including TRC20, which operates on the Tron (TRX) network. Checking your USDT TRC20 balance accurately is crucial for users who hold or transact with this asset. Whether you're sendi...
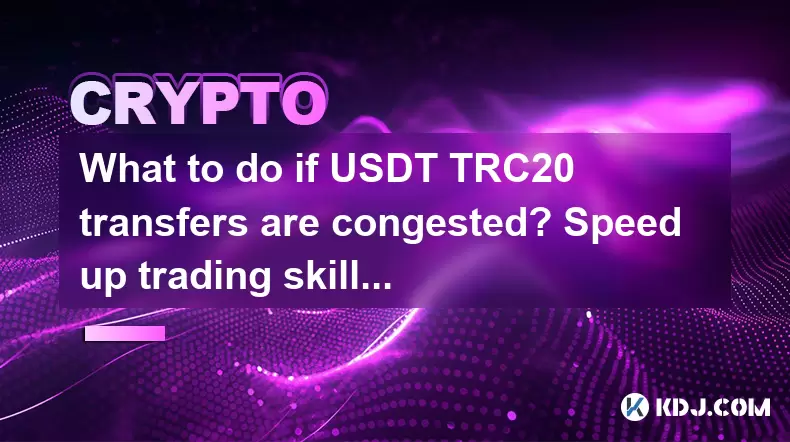
What to do if USDT TRC20 transfers are congested? Speed up trading skills
Jun 13,2025 at 09:56am
Understanding USDT TRC20 Transfer CongestionWhen transferring USDT TRC20, users may occasionally experience delays or congestion. This typically occurs due to network overload on the TRON blockchain, which hosts the TRC20 version of Tether. Unlike the ERC20 variant (which runs on Ethereum), TRC20 transactions are generally faster and cheaper, but during...
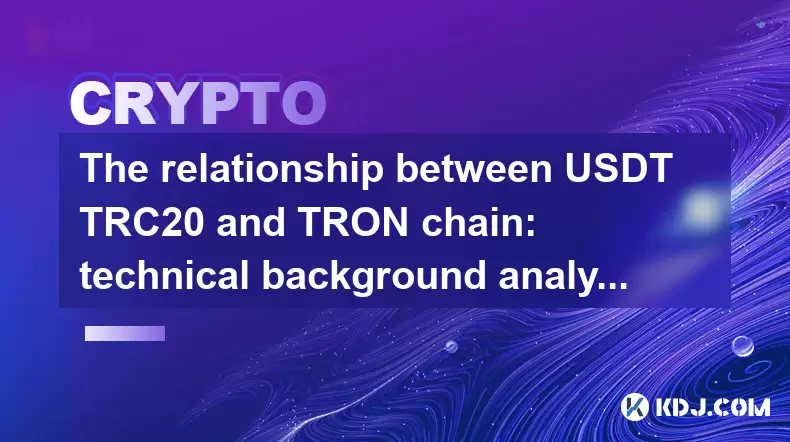
The relationship between USDT TRC20 and TRON chain: technical background analysis
Jun 12,2025 at 01:28pm
What is USDT TRC20?USDT TRC20 refers to the Tether (USDT) token issued on the TRON blockchain using the TRC-20 standard. Unlike the more commonly known ERC-20 version of USDT (which runs on Ethereum), the TRC-20 variant leverages the TRON network's infrastructure for faster and cheaper transactions. The emergence of this version came as part of Tether’s...
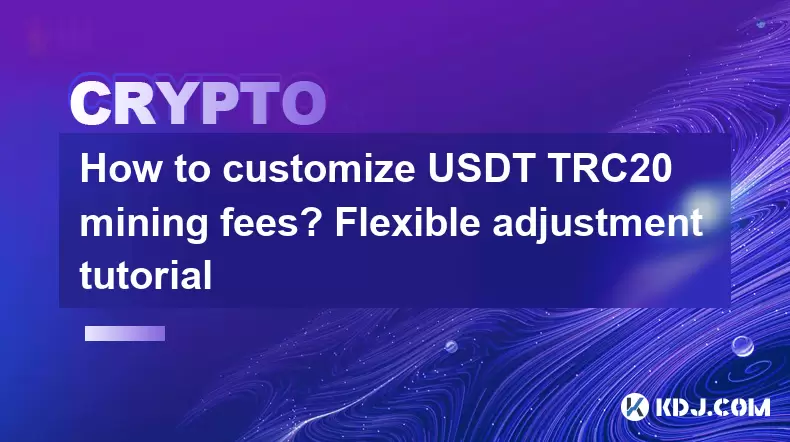
How to customize USDT TRC20 mining fees? Flexible adjustment tutorial
Jun 13,2025 at 01:42am
Understanding USDT TRC20 Mining FeesMining fees on the TRON (TRC20) network are essential for processing transactions. Unlike Bitcoin or Ethereum, where miners directly validate transactions, TRON uses a delegated proof-of-stake (DPoS) mechanism. However, users still need to pay bandwidth and energy fees, which are collectively referred to as 'mining fe...
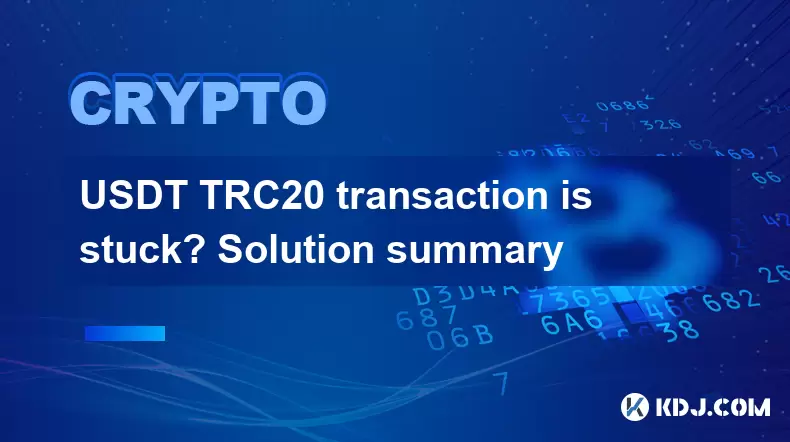
USDT TRC20 transaction is stuck? Solution summary
Jun 14,2025 at 11:15pm
Understanding USDT TRC20 TransactionsWhen users mention that a USDT TRC20 transaction is stuck, they typically refer to a situation where the transfer of Tether (USDT) on the TRON blockchain has not been confirmed for an extended period. This issue may arise due to various reasons such as network congestion, insufficient transaction fees, or wallet-rela...
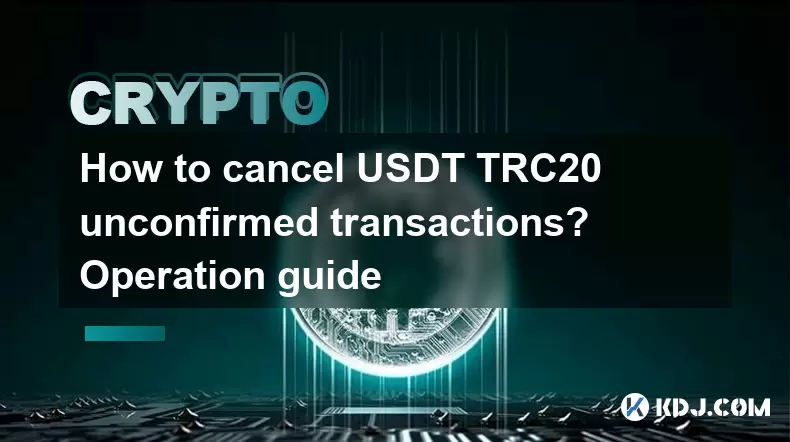
How to cancel USDT TRC20 unconfirmed transactions? Operation guide
Jun 13,2025 at 11:01pm
Understanding USDT TRC20 Unconfirmed TransactionsWhen dealing with USDT TRC20 transactions, it’s crucial to understand what an unconfirmed transaction means. An unconfirmed transaction is one that has been broadcasted to the blockchain network but hasn’t yet been included in a block. This typically occurs due to low transaction fees or network congestio...
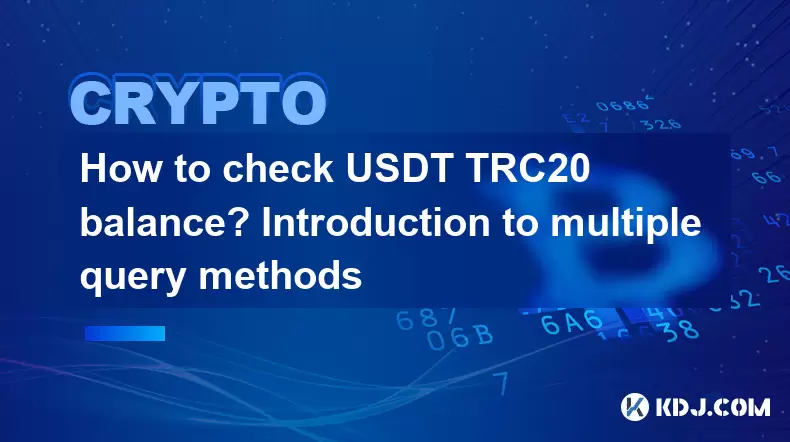
How to check USDT TRC20 balance? Introduction to multiple query methods
Jun 21,2025 at 02:42am
Understanding USDT TRC20 and Its ImportanceUSDT (Tether) is one of the most widely used stablecoins in the cryptocurrency market. It exists on multiple blockchain networks, including TRC20, which operates on the Tron (TRX) network. Checking your USDT TRC20 balance accurately is crucial for users who hold or transact with this asset. Whether you're sendi...
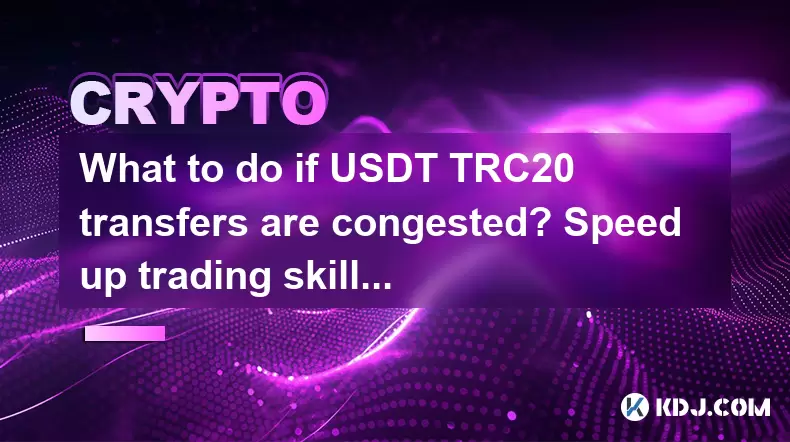
What to do if USDT TRC20 transfers are congested? Speed up trading skills
Jun 13,2025 at 09:56am
Understanding USDT TRC20 Transfer CongestionWhen transferring USDT TRC20, users may occasionally experience delays or congestion. This typically occurs due to network overload on the TRON blockchain, which hosts the TRC20 version of Tether. Unlike the ERC20 variant (which runs on Ethereum), TRC20 transactions are generally faster and cheaper, but during...
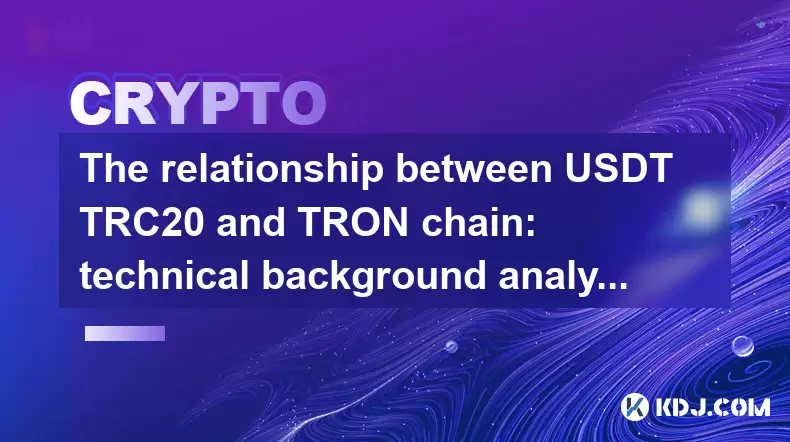
The relationship between USDT TRC20 and TRON chain: technical background analysis
Jun 12,2025 at 01:28pm
What is USDT TRC20?USDT TRC20 refers to the Tether (USDT) token issued on the TRON blockchain using the TRC-20 standard. Unlike the more commonly known ERC-20 version of USDT (which runs on Ethereum), the TRC-20 variant leverages the TRON network's infrastructure for faster and cheaper transactions. The emergence of this version came as part of Tether’s...
See all articles
