-
Bitcoin
$108,228.4534
-0.75% -
Ethereum
$2,524.8743
-1.10% -
Tether USDt
$1.0003
-0.01% -
XRP
$2.2250
-0.63% -
BNB
$656.0016
-0.57% -
Solana
$148.4911
-1.36% -
USDC
$1.0000
0.00% -
TRON
$0.2846
-0.95% -
Dogecoin
$0.1646
-1.38% -
Cardano
$0.5767
-1.03% -
Hyperliquid
$39.3026
0.80% -
Sui
$2.9291
0.05% -
Bitcoin Cash
$483.6554
0.19% -
Chainlink
$13.2526
-1.01% -
UNUS SED LEO
$9.0390
-0.17% -
Avalanche
$17.9293
-1.10% -
Stellar
$0.2376
-1.15% -
Toncoin
$2.7533
-1.89% -
Shiba Inu
$0.0...01149
-0.80% -
Hedera
$0.1563
0.26% -
Litecoin
$86.7275
-1.92% -
Monero
$311.5944
-2.47% -
Polkadot
$3.3741
-1.97% -
Dai
$1.0000
0.01% -
Ethena USDe
$1.0002
0.00% -
Bitget Token
$4.4043
-1.32% -
Uniswap
$7.0131
-4.90% -
Aave
$274.3481
1.75% -
Pepe
$0.0...09808
0.11% -
Pi
$0.4677
-3.26%
How to get LINK market information with Python? API interface call tutorial
Use Python and CoinGecko API to fetch real-time LINK market data, including current price, 24-hour change, and market cap, with error handling for robust scripting.
Apr 29, 2025 at 04:28 pm
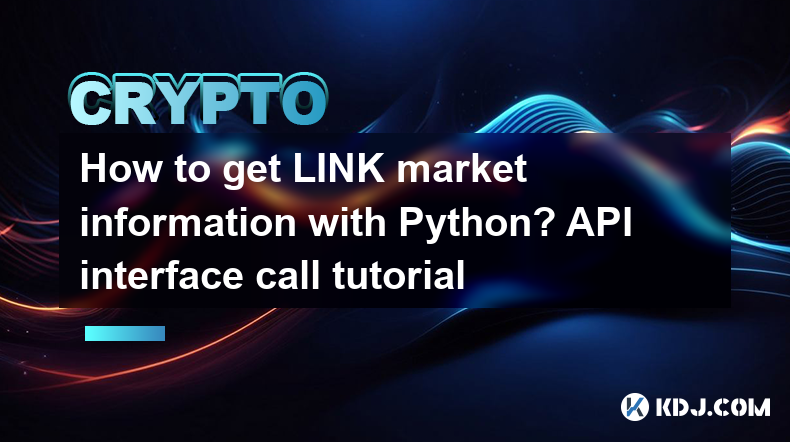
To obtain LINK market information using Python, we'll use an API interface to fetch real-time data. In this tutorial, we'll walk through the process of setting up the necessary tools, making API calls, and processing the data. We'll use the CoinGecko API, which provides free access to a wide range of cryptocurrency data, including LINK.
Setting Up the Environment
Before we can start making API calls, we need to set up our Python environment. This involves installing the necessary libraries and setting up a script to work with.
Install the
requests
library: This library will allow us to make HTTP requests to the CoinGecko API. Open your terminal or command prompt and run:pip install requests
Create a new Python file: Open your preferred text editor and create a new file named
link_market_info.py
. This file will contain our script for fetching LINK market information.
Making the API Call
Now that our environment is set up, we can proceed to make an API call to retrieve LINK market information. We'll use the CoinGecko API endpoint for fetching coin data.
Import the
requests
library: At the top of yourlink_market_info.py
file, add the following line to import therequests
library:import requests
Define the API endpoint: We'll use the
/coins/{id}
endpoint to fetch data for LINK. Theid
for LINK on CoinGecko ischainlink
. Add the following line to define the API endpoint:api_url = "https://api.coingecko.com/api/v3/coins/chainlink"
Make the API call: Use the
requests.get()
method to fetch the data from the API. Add the following lines to your script:response = requests.get(api_url)
data = response.json()Check the response: It's good practice to check if the API call was successful. Add the following lines to handle potential errors:
if response.status_code == 200:
print("Successfully fetched LINK market information.")
else:
print("Failed to fetch LINK market information. Status code:", response.status_code)
Extracting Market Information
With the data fetched, we can now extract specific market information about LINK. The CoinGecko API returns a JSON object with various fields, including market data.
Extract current price: To get the current price of LINK in USD, we can access the
market_data
field. Add the following lines to your script:current_price_usd = data['market_data']['current_price']['usd']
print(f"Current price of LINK in USD: ${current_price_usd}")
Extract 24-hour price change: To get the 24-hour price change percentage, we can access the
price_change_percentage_24h
field. Add the following lines:price_change_24h = data'market_data'
print(f"24-hour price change percentage: {price_change_24h}%")Extract market cap: To get the current market capitalization of LINK, we can access the
market_cap
field. Add the following lines:market_cap_usd = data'market_data'['usd']
print(f"Current market cap of LINK in USD: ${market_cap_usd}")
Handling Errors and Edge Cases
When working with APIs, it's important to handle potential errors and edge cases to ensure your script remains robust.
Handle JSON decoding errors: If the API response is not in the expected JSON format, we should handle this gracefully. Add the following lines to your script:
try:
data = response.json()
except ValueError:
print("Failed to decode JSON response.") exit(1)
Check for missing data: Sometimes, the API might return incomplete data. We should check for this and handle it appropriately. Add the following lines to check for missing fields:
if 'market_data' not in data: print("Market data not available in the API response.") exit(1)
if 'current_price' not in data['market_data'] or 'usd' not in data'market_data':
print("Current price data not available.") exit(1)
if 'price_change_percentage_24h' not in data['market_data']:
print("24-hour price change data not available.") exit(1)
if 'market_cap' not in data['market_data'] or 'usd' not in data'market_data':
print("Market cap data not available.") exit(1)
Putting It All Together
Now that we've covered all the necessary steps, let's put everything together into a complete script. Here's the full link_market_info.py
file:
import requests
api_url = "https://api.coingecko.com/api/v3/coins/chainlink"
response = requests.get(api_url)
if response.status_code == 200:
print("Successfully fetched LINK market information.")
else:
print("Failed to fetch LINK market information. Status code:", response.status_code)
exit(1)
try:
data = response.json()
except ValueError:
print("Failed to decode JSON response.")
exit(1)
if 'market_data' not in data:
print("Market data not available in the API response.")
exit(1)
if 'current_price' not in data['market_data'] or 'usd' not in data'market_data':
print("Current price data not available.")
exit(1)
if 'price_change_percentage_24h' not in data['market_data']:
print("24-hour price change data not available.")
exit(1)
if 'market_cap' not in data['market_data'] or 'usd' not in data'market_data':
print("Market cap data not available.")
exit(1)
current_price_usd = data'market_data'['usd']
print(f"Current price of LINK in USD: ${current_price_usd}")
price_change_24h = data'market_data'
print(f"24-hour price change percentage: {price_change_24h}%")
market_cap_usd = data'market_data'['usd']
print(f"Current market cap of LINK in USD: ${market_cap_usd}")
Frequently Asked Questions
Q: Can I use this script to fetch market information for other cryptocurrencies?
A: Yes, you can modify the api_url
to use the CoinGecko API endpoint for other cryptocurrencies. Simply replace chainlink
with the appropriate id
for the cryptocurrency you're interested in. You can find the id
for each cryptocurrency on the CoinGecko website.
Q: How often can I make API calls to CoinGecko without hitting rate limits?
A: CoinGecko has a rate limit of 50 requests per minute for unauthenticated requests. If you need to make more frequent requests, you can sign up for an API key, which allows up to 100 requests per minute.
Q: Can I use this script to fetch historical price data for LINK?
A: The script provided in this tutorial fetches current market data. To fetch historical price data, you would need to use a different CoinGecko API endpoint, such as /coins/{id}/market_chart
. You would need to modify the script to include parameters for the desired time range and interval.
Q: Is there a way to automate this script to run at regular intervals?
A: Yes, you can use Python's schedule
library to run the script at regular intervals. After installing the schedule
library with pip install schedule
, you can modify the script to include a scheduling function that calls the main script every few minutes or hours, depending on your needs.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Bitcoin, Ripple, and TPS: A New Sheriff in Town?
- 2025-07-05 19:16:07
- Bitcoin Investment: Will You 10x by 2030?
- 2025-07-05 19:16:07
- BONK on Solana: Meme Coin Mania or the Real Deal?
- 2025-07-05 19:16:08
- PEPE Coin, Neo Pepe, and Market Makers: Decoding the Latest Crypto Moves
- 2025-07-05 19:16:08
- BONK Price Rockets: ETF Buzz Sparks Rally and Breakout!
- 2025-07-05 19:16:10
- BONK Price Rockets: Rally, Breakout, and What's Next for the Meme Coin
- 2025-07-05 19:16:10
Related knowledge
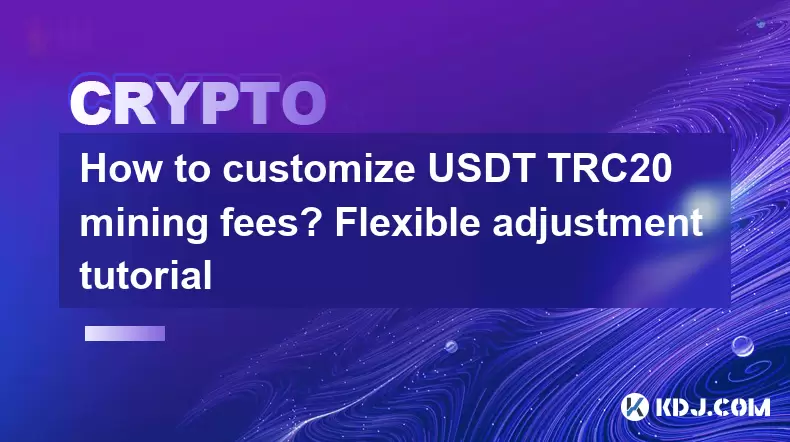
How to customize USDT TRC20 mining fees? Flexible adjustment tutorial
Jun 13,2025 at 01:42am
Understanding USDT TRC20 Mining FeesMining fees on the TRON (TRC20) network are essential for processing transactions. Unlike Bitcoin or Ethereum, where miners directly validate transactions, TRON uses a delegated proof-of-stake (DPoS) mechanism. However, users still need to pay bandwidth and energy fees, which are collectively referred to as 'mining fe...
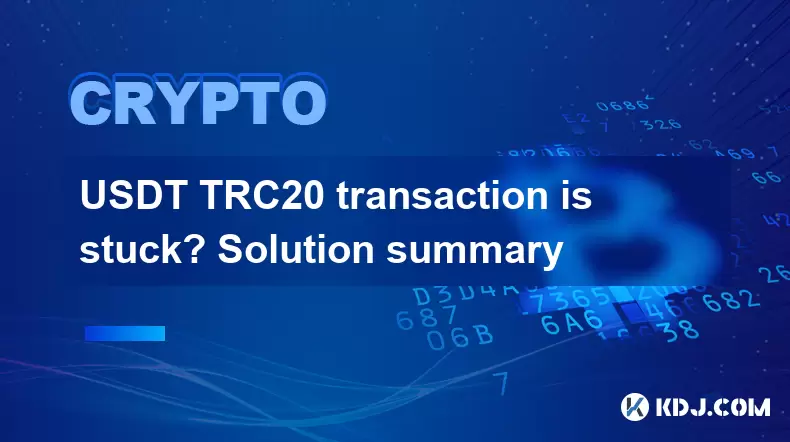
USDT TRC20 transaction is stuck? Solution summary
Jun 14,2025 at 11:15pm
Understanding USDT TRC20 TransactionsWhen users mention that a USDT TRC20 transaction is stuck, they typically refer to a situation where the transfer of Tether (USDT) on the TRON blockchain has not been confirmed for an extended period. This issue may arise due to various reasons such as network congestion, insufficient transaction fees, or wallet-rela...
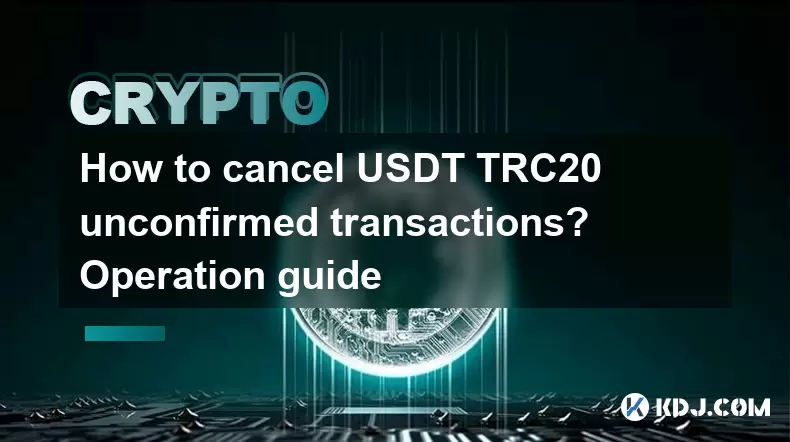
How to cancel USDT TRC20 unconfirmed transactions? Operation guide
Jun 13,2025 at 11:01pm
Understanding USDT TRC20 Unconfirmed TransactionsWhen dealing with USDT TRC20 transactions, it’s crucial to understand what an unconfirmed transaction means. An unconfirmed transaction is one that has been broadcasted to the blockchain network but hasn’t yet been included in a block. This typically occurs due to low transaction fees or network congestio...
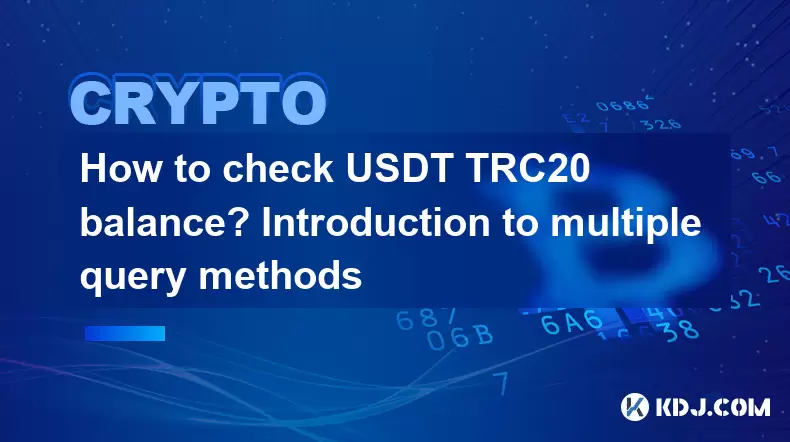
How to check USDT TRC20 balance? Introduction to multiple query methods
Jun 21,2025 at 02:42am
Understanding USDT TRC20 and Its ImportanceUSDT (Tether) is one of the most widely used stablecoins in the cryptocurrency market. It exists on multiple blockchain networks, including TRC20, which operates on the Tron (TRX) network. Checking your USDT TRC20 balance accurately is crucial for users who hold or transact with this asset. Whether you're sendi...
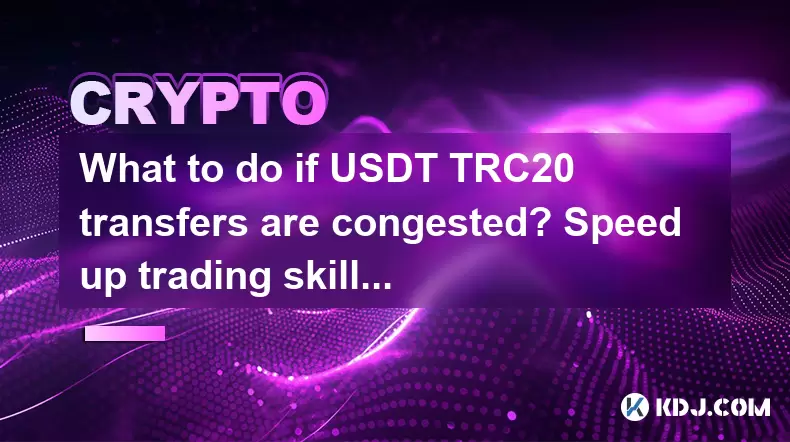
What to do if USDT TRC20 transfers are congested? Speed up trading skills
Jun 13,2025 at 09:56am
Understanding USDT TRC20 Transfer CongestionWhen transferring USDT TRC20, users may occasionally experience delays or congestion. This typically occurs due to network overload on the TRON blockchain, which hosts the TRC20 version of Tether. Unlike the ERC20 variant (which runs on Ethereum), TRC20 transactions are generally faster and cheaper, but during...
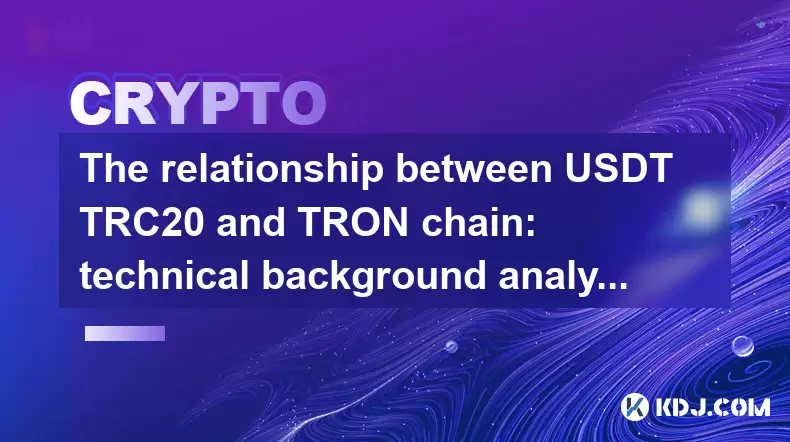
The relationship between USDT TRC20 and TRON chain: technical background analysis
Jun 12,2025 at 01:28pm
What is USDT TRC20?USDT TRC20 refers to the Tether (USDT) token issued on the TRON blockchain using the TRC-20 standard. Unlike the more commonly known ERC-20 version of USDT (which runs on Ethereum), the TRC-20 variant leverages the TRON network's infrastructure for faster and cheaper transactions. The emergence of this version came as part of Tether’s...
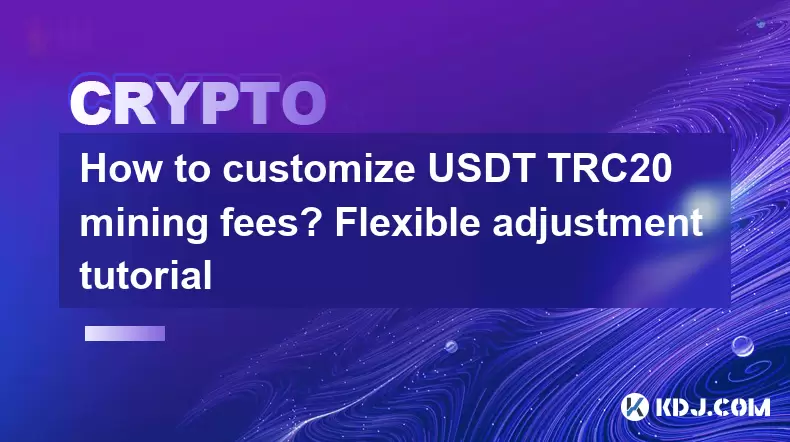
How to customize USDT TRC20 mining fees? Flexible adjustment tutorial
Jun 13,2025 at 01:42am
Understanding USDT TRC20 Mining FeesMining fees on the TRON (TRC20) network are essential for processing transactions. Unlike Bitcoin or Ethereum, where miners directly validate transactions, TRON uses a delegated proof-of-stake (DPoS) mechanism. However, users still need to pay bandwidth and energy fees, which are collectively referred to as 'mining fe...
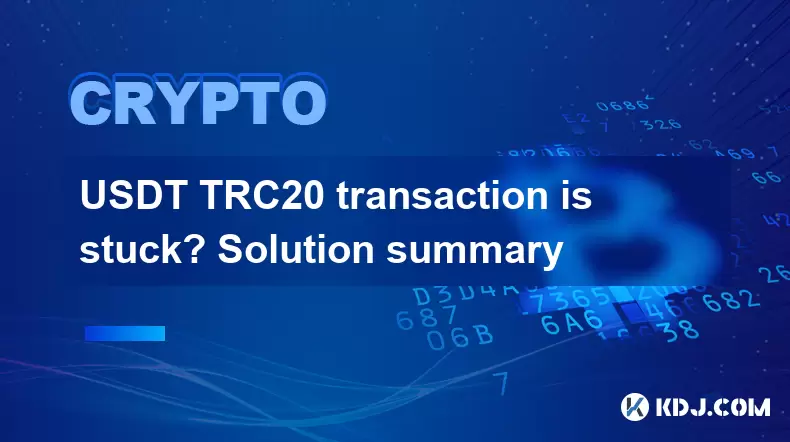
USDT TRC20 transaction is stuck? Solution summary
Jun 14,2025 at 11:15pm
Understanding USDT TRC20 TransactionsWhen users mention that a USDT TRC20 transaction is stuck, they typically refer to a situation where the transfer of Tether (USDT) on the TRON blockchain has not been confirmed for an extended period. This issue may arise due to various reasons such as network congestion, insufficient transaction fees, or wallet-rela...
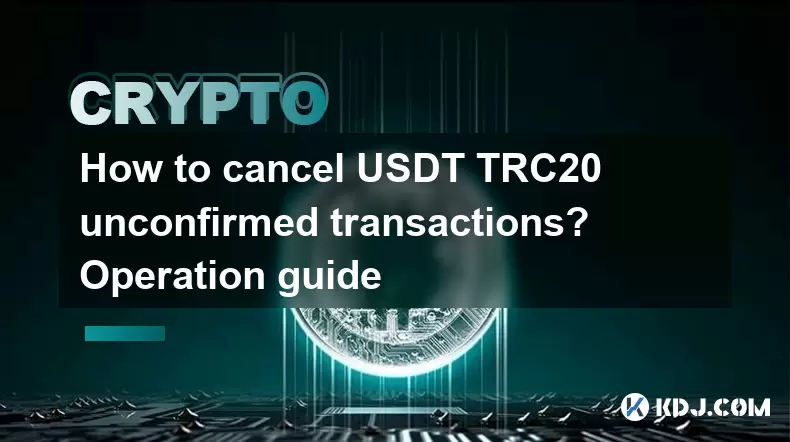
How to cancel USDT TRC20 unconfirmed transactions? Operation guide
Jun 13,2025 at 11:01pm
Understanding USDT TRC20 Unconfirmed TransactionsWhen dealing with USDT TRC20 transactions, it’s crucial to understand what an unconfirmed transaction means. An unconfirmed transaction is one that has been broadcasted to the blockchain network but hasn’t yet been included in a block. This typically occurs due to low transaction fees or network congestio...
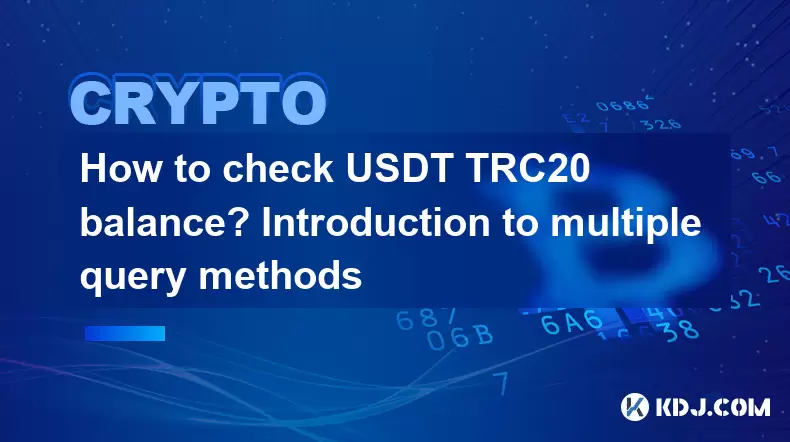
How to check USDT TRC20 balance? Introduction to multiple query methods
Jun 21,2025 at 02:42am
Understanding USDT TRC20 and Its ImportanceUSDT (Tether) is one of the most widely used stablecoins in the cryptocurrency market. It exists on multiple blockchain networks, including TRC20, which operates on the Tron (TRX) network. Checking your USDT TRC20 balance accurately is crucial for users who hold or transact with this asset. Whether you're sendi...
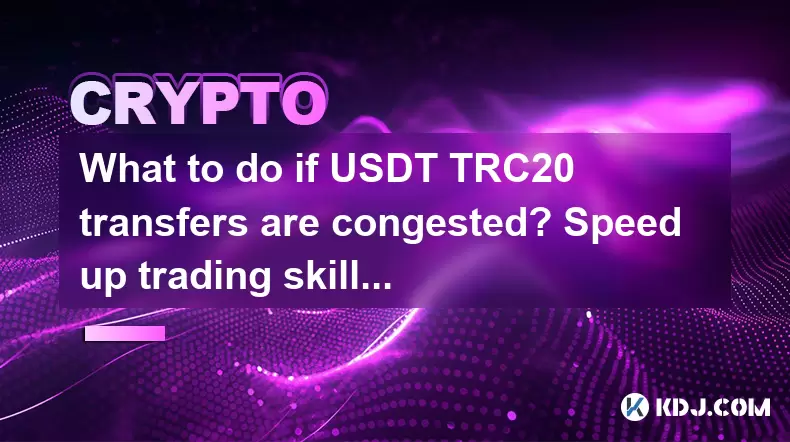
What to do if USDT TRC20 transfers are congested? Speed up trading skills
Jun 13,2025 at 09:56am
Understanding USDT TRC20 Transfer CongestionWhen transferring USDT TRC20, users may occasionally experience delays or congestion. This typically occurs due to network overload on the TRON blockchain, which hosts the TRC20 version of Tether. Unlike the ERC20 variant (which runs on Ethereum), TRC20 transactions are generally faster and cheaper, but during...
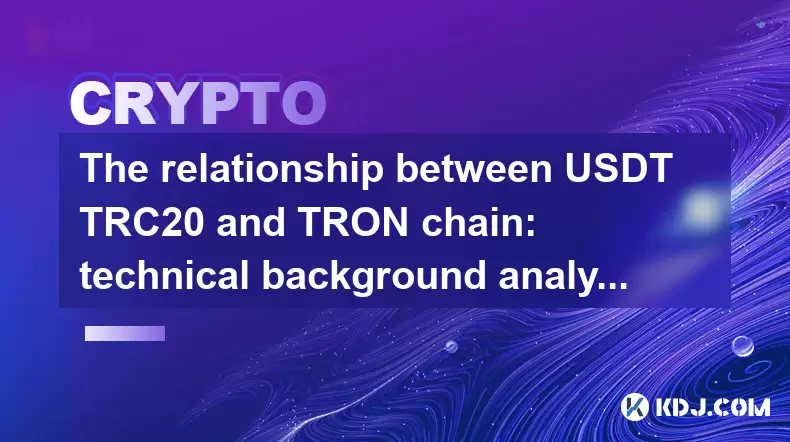
The relationship between USDT TRC20 and TRON chain: technical background analysis
Jun 12,2025 at 01:28pm
What is USDT TRC20?USDT TRC20 refers to the Tether (USDT) token issued on the TRON blockchain using the TRC-20 standard. Unlike the more commonly known ERC-20 version of USDT (which runs on Ethereum), the TRC-20 variant leverages the TRON network's infrastructure for faster and cheaper transactions. The emergence of this version came as part of Tether’s...
See all articles
