-
Bitcoin
$94,714.4389
-0.29% -
Ethereum
$1,803.4524
-0.61% -
Tether USDt
$1.0001
-0.03% -
XRP
$2.2093
-3.16% -
BNB
$599.7431
-0.28% -
Solana
$147.2526
-0.95% -
USDC
$1.0001
0.00% -
Dogecoin
$0.1742
-1.92% -
Cardano
$0.6893
-2.17% -
TRON
$0.2469
0.34% -
Sui
$3.4819
-1.65% -
Chainlink
$14.4177
-2.59% -
Avalanche
$21.1959
-2.77% -
Stellar
$0.2724
-2.29% -
UNUS SED LEO
$9.0463
0.59% -
Toncoin
$3.2007
-0.24% -
Shiba Inu
$0.0...01336
-1.65% -
Hedera
$0.1843
-2.12% -
Bitcoin Cash
$367.5524
-0.59% -
Polkadot
$4.1359
-1.61% -
Litecoin
$84.4117
-2.21% -
Hyperliquid
$18.9168
0.03% -
Dai
$1.0000
-0.01% -
Bitget Token
$4.3285
-1.35% -
Monero
$271.4103
0.27% -
Ethena USDe
$1.0003
0.07% -
Pi
$0.6159
5.36% -
Pepe
$0.0...08957
-0.84% -
Uniswap
$5.3425
0.41% -
Aptos
$5.4013
-2.47%
How to use Bithumb's API?
Using Bithumb's API can enhance trading strategies and automate processes; this guide covers registration, API key setup, and making your first API call effectively.
Apr 18, 2025 at 08:49 pm
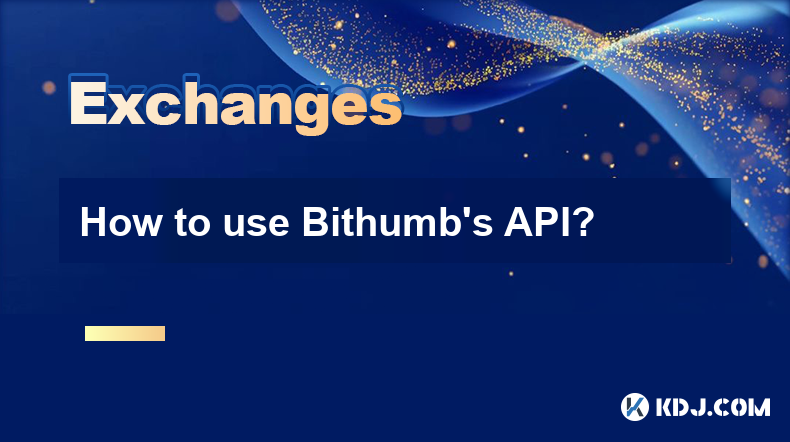
Using Bithumb's API can significantly enhance your trading strategies, automate processes, and access real-time data. In this guide, we will walk you through the steps to use Bithumb's API effectively, covering everything from registration to executing your first API call.
Registering and Setting Up an API Key
Before you can use Bithumb's API, you need to register for an account and set up an API key. Here's how you can do it:
- Visit the Bithumb website and sign up for a new account if you don't already have one. You'll need to provide your personal details and complete the verification process.
- Log into your Bithumb account. Navigate to the API Management section, which is usually found under the account settings or security settings.
- Create a new API key. You will be prompted to enter a name for your API key, which helps you manage multiple keys if needed. Make sure to enable the necessary permissions based on what you intend to do with the API.
- Generate the API key. You will receive an API Key and an API Secret. These are crucial for authenticating your API requests, so keep them secure.
Understanding Bithumb's API Endpoints
Bithumb's API is divided into several endpoints, each serving a specific purpose. Here are some key endpoints you should know:
- Public API: These endpoints do not require authentication and are used to fetch market data, such as ticker information, order books, and transaction history.
- Private API: These endpoints require authentication and allow you to perform actions like placing orders, canceling orders, and managing your account balance.
Making Your First API Call
To make your first API call, you'll need to use a programming language like Python, JavaScript, or any other language that supports HTTP requests. Here's a step-by-step guide using Python:
- Install the necessary libraries. You'll need
requests
to make HTTP requests andhmac
for signing your requests. You can install them using pip:
pip install requests
- Import the libraries in your Python script:
import requests
import hmac
import hashlib
import time
- Set up your API credentials:
api_key = 'YOUR_API_KEY'
api_secret = 'YOUR_API_SECRET'
- Construct the API call. Let's use the public API to fetch the ticker information for Bitcoin (BTC):
endpoint = 'https://api.bithumb.com/public/ticker/BTC'
response = requests.get(endpoint)
print(response.json())
- For private API calls, you need to sign your requests. Here's an example of how to place a buy order:
endpoint = 'https://api.bithumb.com/trade/place'
params = {'order_currency': 'BTC',
'payment_currency': 'KRW',
'units': '0.001',
'price': '1000000',
'type': 'bid'
}
params['endpoint'] = endpoint
nonce = str(int(time.time() * 1000))
params['nonce'] = nonce
Create the signature
query_string = '&'.join([f"{k}={v}" for k, v in sorted(params.items())])
signature = hmac.new(api_secret.encode('utf-8'), query_string.encode('utf-8'), hashlib.sha512).hexdigest()
headers = {
'Api-Key': api_key,
'Api-Sign': signature,
'Content-Type': 'application/x-www-form-urlencoded'
}
response = requests.post(endpoint, data=params, headers=headers)
print(response.json())
Handling Errors and Responses
When using Bithumb's API, it's important to handle errors and parse responses correctly. Here's how you can do it:
- Check the status code of the response. A status code of 200 indicates success, while other codes indicate errors.
- Parse the JSON response. Bithumb's API returns data in JSON format, which you can easily parse using Python's
json
module.
import jsonif response.status_code == 200:
data = response.json()
if data['status'] == '0000':
print("Success:", data)
else:
print("Error:", data['message'])
else:
print("HTTP Error:", response.status_code)
Using Bithumb's WebSocket API
For real-time data, Bithumb offers a WebSocket API. Here's how you can connect to it using Python:
- Install the
websocket-client
library:
pip install websocket-client
- Connect to the WebSocket:
import websocket
import json
def on_message(ws, message):
data = json.loads(message)
print(data)
def on_error(ws, error):
print(error)
def on_close(ws):
print("### closed ###")
def on_open(ws):
print("### opened ###")
websocket.enableTrace(True)
ws = websocket.WebSocketApp("wss://pubwss.bithumb.com/pub/ws",
on_message=on_message,
on_error=on_error,
on_close=on_close)
ws.on_open = on_open
ws.run_forever()
Best Practices for Using Bithumb's API
To ensure you use Bithumb's API effectively and securely, consider the following best practices:
- Secure your API keys. Never share your API keys or store them in version control systems. Use environment variables or secure vaults to store them.
- Rate limiting. Be mindful of Bithumb's rate limits to avoid having your API access restricted. Implement retry mechanisms with exponential backoff for handling rate limit errors.
- Error handling. Always include robust error handling in your code to deal with API failures gracefully.
- Testing. Before deploying your code to production, thoroughly test it in a sandbox or test environment to ensure it works as expected.
Frequently Asked Questions
Q: Can I use Bithumb's API for automated trading?
A: Yes, Bithumb's API supports automated trading through its private endpoints, allowing you to place orders, cancel orders, and manage your account balance programmatically.
Q: How often is the data updated on Bithumb's API?
A: The public API endpoints, such as ticker and order book data, are typically updated in real-time, while private API endpoints may have varying update frequencies based on your account's activity.
Q: Is there a limit to the number of API keys I can create on Bithumb?
A: Bithumb may have a limit on the number of API keys you can create per account. You should check the API documentation or contact Bithumb support for specific details.
Q: Can I use Bithumb's API to fetch historical data?
A: Bithumb's API primarily provides real-time data. For historical data, you might need to use third-party services or manually collect and store the data over time.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- NOIDA (CoinChapter.com) — Despite Favorable Technicals, XRP Price Underperforms BTC and ETH
- 2025-04-30 23:40:12
- Elderly US individual loses $330 million in Bitcoin to social engineering hack, now the fifth-largest crypto heist
- 2025-04-30 23:40:12
- Charles Hoskinson Takes a Jab at Bitcoin's Internal Struggles Over Controversial Code Update
- 2025-04-30 23:35:13
- How Much XRP Could Make You a Millionaire in Just a Few Years?
- 2025-04-30 23:35:13
- The U.S. Securities and Exchange Commission (SEC) has postponed decisions on the approval of ETFs for XRP and Dogecoin.
- 2025-04-30 23:30:12
- Solana (SOL) Surges Past Ethereum and Binance Smart Chain in Net Transfer Volume
- 2025-04-30 23:30:12
Related knowledge
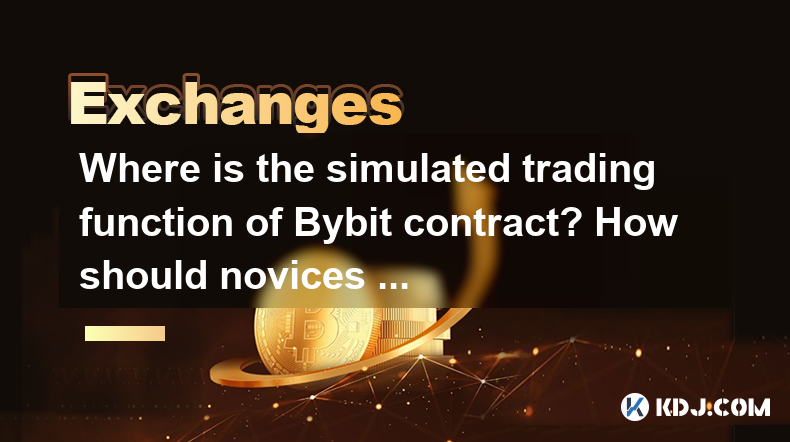
Where is the simulated trading function of Bybit contract? How should novices practice?
Apr 30,2025 at 11:14pm
Bybit, a leading cryptocurrency derivatives trading platform, offers a simulated trading function that allows users to practice trading without risking real money. This feature is particularly beneficial for novices who want to familiarize themselves with the platform and the dynamics of trading cryptocurrency contracts. In this article, we will explore...
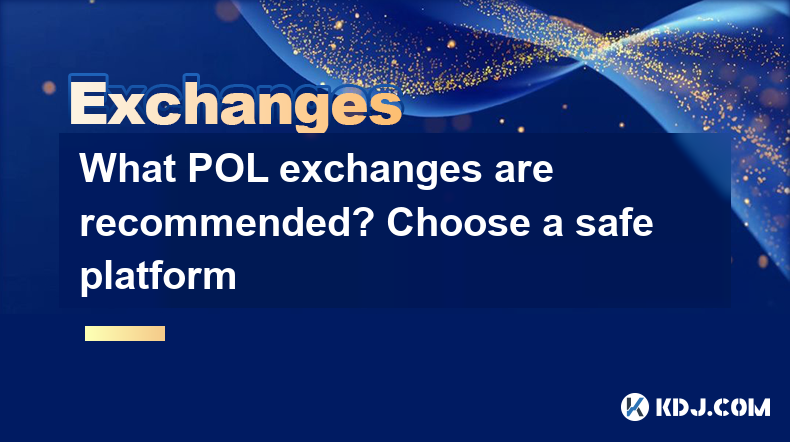
What POL exchanges are recommended? Choose a safe platform
Apr 30,2025 at 12:57pm
In the ever-evolving world of cryptocurrencies, selecting a safe and reliable exchange to trade POL (Polkadot) is crucial. This article will guide you through some of the recommended POL exchanges and help you choose a platform that prioritizes safety and security. We will delve into the features, benefits, and step-by-step processes for using these exc...
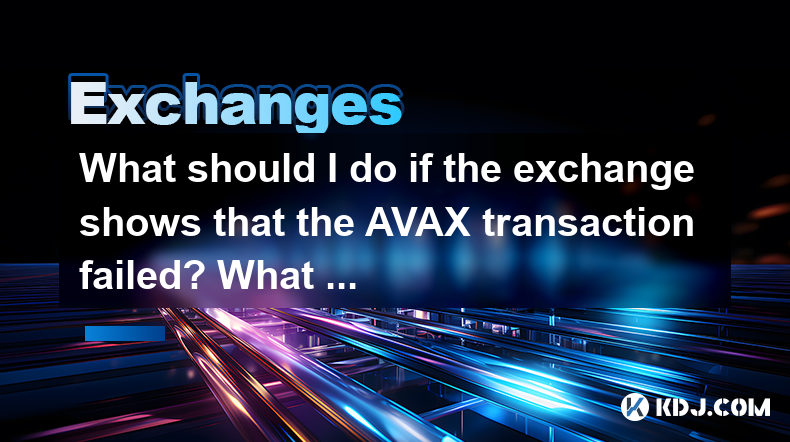
What should I do if the exchange shows that the AVAX transaction failed? What are the common reasons?
Apr 29,2025 at 03:42pm
If you encounter a situation where the AVAX transaction on an exchange shows as failed, it can be frustrating and confusing. Understanding the common reasons behind this issue and knowing the steps to take can help you resolve the problem more effectively. In this article, we will explore the common reasons for AVAX transaction failures on exchanges and...
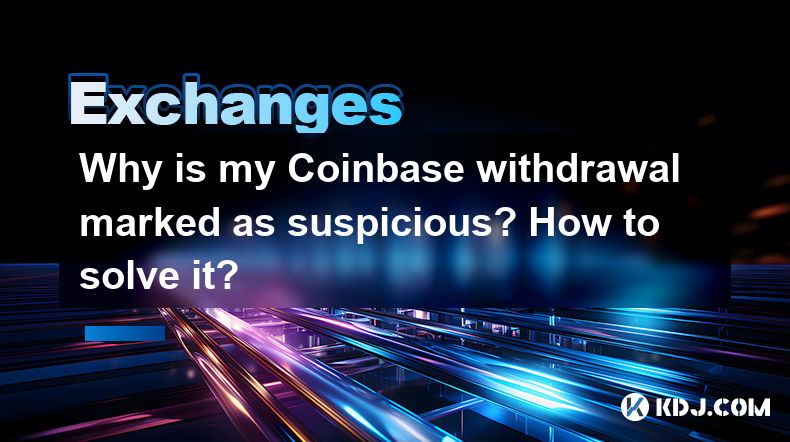
Why is my Coinbase withdrawal marked as suspicious? How to solve it?
Apr 29,2025 at 02:49am
If you've encountered a situation where your Coinbase withdrawal is marked as suspicious, it can be both frustrating and confusing. Understanding why this happens and how to resolve it is crucial for a smooth experience with your cryptocurrency transactions. This article will delve into the reasons behind Coinbase marking withdrawals as suspicious and p...
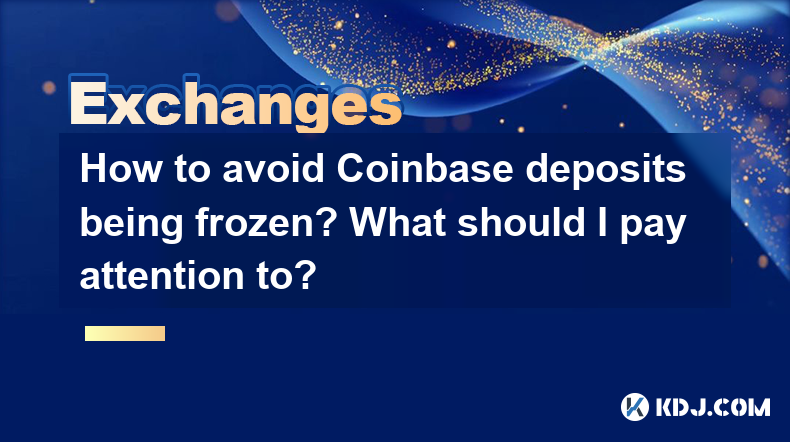
How to avoid Coinbase deposits being frozen? What should I pay attention to?
Apr 27,2025 at 11:57pm
Understanding Coinbase Deposit FreezingCoinbase, one of the largest cryptocurrency exchanges, occasionally freezes deposits for various reasons. Understanding why your deposits might be frozen is crucial for preventing such occurrences. Common reasons include suspicious activity, account verification issues, or failure to comply with regulatory requirem...
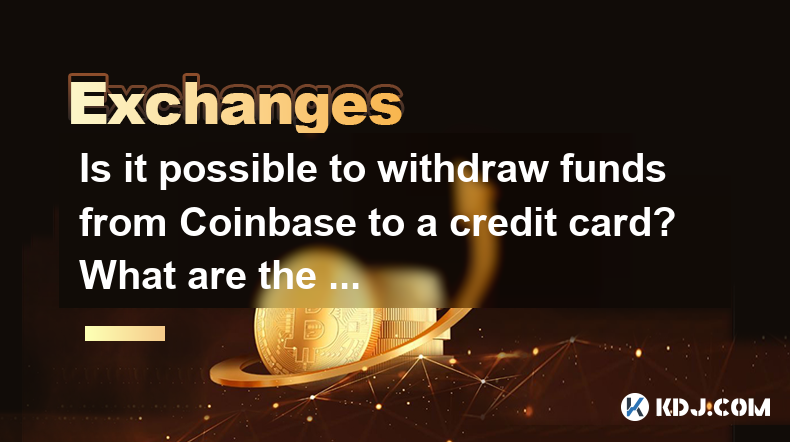
Is it possible to withdraw funds from Coinbase to a credit card? What are the restrictions?
Apr 28,2025 at 05:57pm
Is it possible to withdraw funds from Coinbase to a credit card? What are the restrictions? When it comes to managing your cryptocurrency, understanding the various methods of moving funds in and out of your accounts is crucial. One common question many users have is whether it's possible to withdraw funds from Coinbase directly to a credit card. In thi...
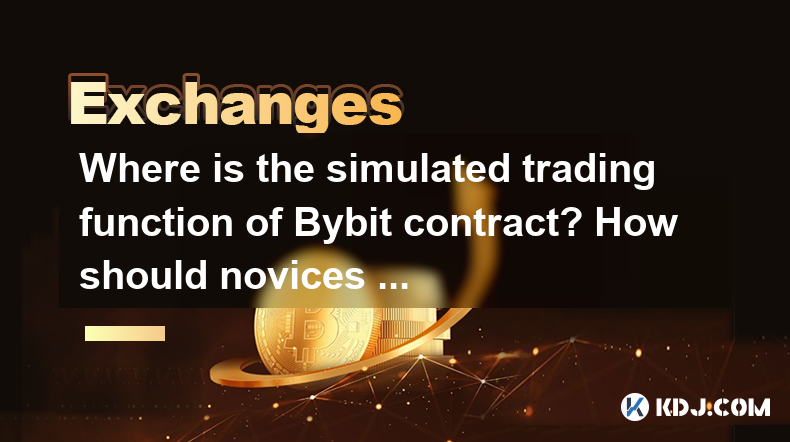
Where is the simulated trading function of Bybit contract? How should novices practice?
Apr 30,2025 at 11:14pm
Bybit, a leading cryptocurrency derivatives trading platform, offers a simulated trading function that allows users to practice trading without risking real money. This feature is particularly beneficial for novices who want to familiarize themselves with the platform and the dynamics of trading cryptocurrency contracts. In this article, we will explore...
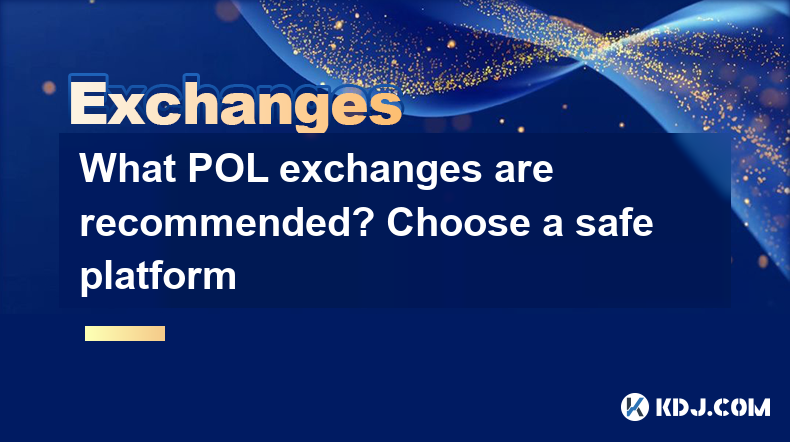
What POL exchanges are recommended? Choose a safe platform
Apr 30,2025 at 12:57pm
In the ever-evolving world of cryptocurrencies, selecting a safe and reliable exchange to trade POL (Polkadot) is crucial. This article will guide you through some of the recommended POL exchanges and help you choose a platform that prioritizes safety and security. We will delve into the features, benefits, and step-by-step processes for using these exc...
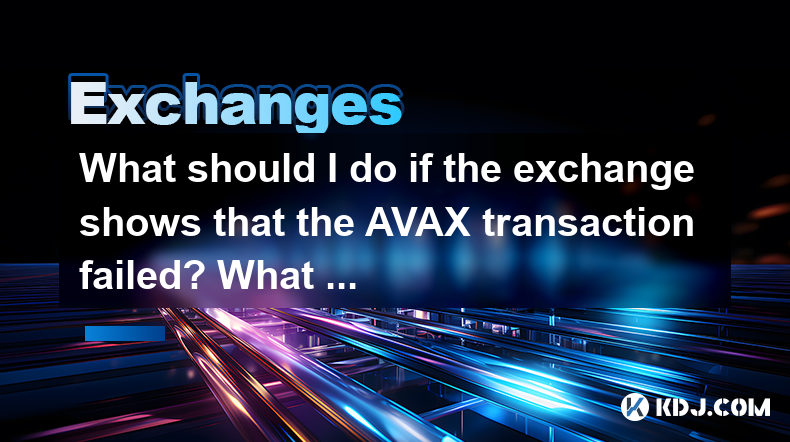
What should I do if the exchange shows that the AVAX transaction failed? What are the common reasons?
Apr 29,2025 at 03:42pm
If you encounter a situation where the AVAX transaction on an exchange shows as failed, it can be frustrating and confusing. Understanding the common reasons behind this issue and knowing the steps to take can help you resolve the problem more effectively. In this article, we will explore the common reasons for AVAX transaction failures on exchanges and...
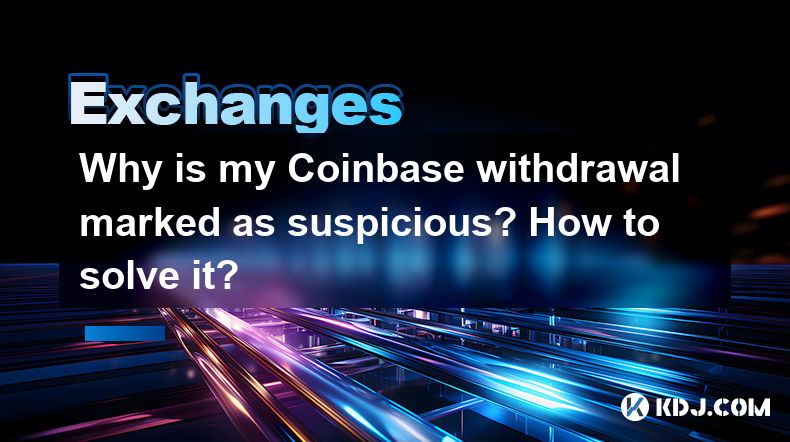
Why is my Coinbase withdrawal marked as suspicious? How to solve it?
Apr 29,2025 at 02:49am
If you've encountered a situation where your Coinbase withdrawal is marked as suspicious, it can be both frustrating and confusing. Understanding why this happens and how to resolve it is crucial for a smooth experience with your cryptocurrency transactions. This article will delve into the reasons behind Coinbase marking withdrawals as suspicious and p...
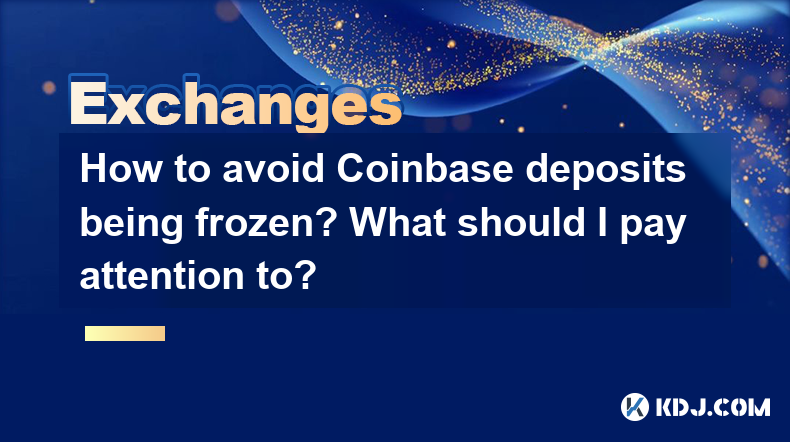
How to avoid Coinbase deposits being frozen? What should I pay attention to?
Apr 27,2025 at 11:57pm
Understanding Coinbase Deposit FreezingCoinbase, one of the largest cryptocurrency exchanges, occasionally freezes deposits for various reasons. Understanding why your deposits might be frozen is crucial for preventing such occurrences. Common reasons include suspicious activity, account verification issues, or failure to comply with regulatory requirem...
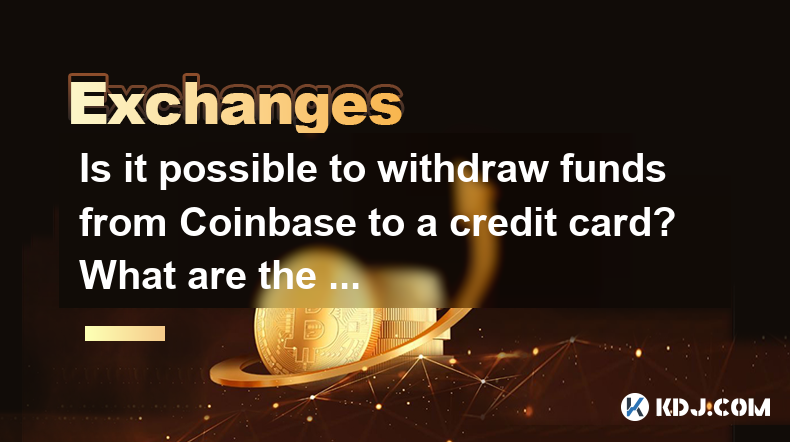
Is it possible to withdraw funds from Coinbase to a credit card? What are the restrictions?
Apr 28,2025 at 05:57pm
Is it possible to withdraw funds from Coinbase to a credit card? What are the restrictions? When it comes to managing your cryptocurrency, understanding the various methods of moving funds in and out of your accounts is crucial. One common question many users have is whether it's possible to withdraw funds from Coinbase directly to a credit card. In thi...
See all articles
