-
Bitcoin
$94,312.0993
-0.62% -
Ethereum
$1,805.7103
0.53% -
Tether USDt
$1.0005
0.02% -
XRP
$2.1998
0.24% -
BNB
$608.1016
0.72% -
Solana
$148.9611
-1.74% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1813
-0.43% -
Cardano
$0.7074
-1.68% -
TRON
$0.2529
4.08% -
Sui
$3.4699
-3.15% -
Chainlink
$14.8586
-1.44% -
Avalanche
$22.0449
-1.95% -
Stellar
$0.2902
1.58% -
Toncoin
$3.3348
3.29% -
UNUS SED LEO
$9.0795
1.97% -
Shiba Inu
$0.0...01417
1.79% -
Hedera
$0.1915
-3.07% -
Bitcoin Cash
$358.7537
-5.08% -
Polkadot
$4.2816
-0.16% -
Litecoin
$87.2835
0.52% -
Hyperliquid
$17.8206
-2.83% -
Dai
$1.0000
0.01% -
Bitget Token
$4.4047
-0.78% -
Ethena USDe
$0.9996
0.00% -
Pi
$0.6482
0.54% -
Monero
$230.0411
0.66% -
Pepe
$0.0...09137
4.23% -
Uniswap
$5.8009
-1.27% -
Aptos
$5.6368
1.59%
How to use Bitfinex's WebSocket API?
To use Bitfinex's WebSocket API for crypto trading, establish a connection, subscribe to channels like ticker data, and handle incoming data for real-time updates.
Apr 23, 2025 at 06:43 am
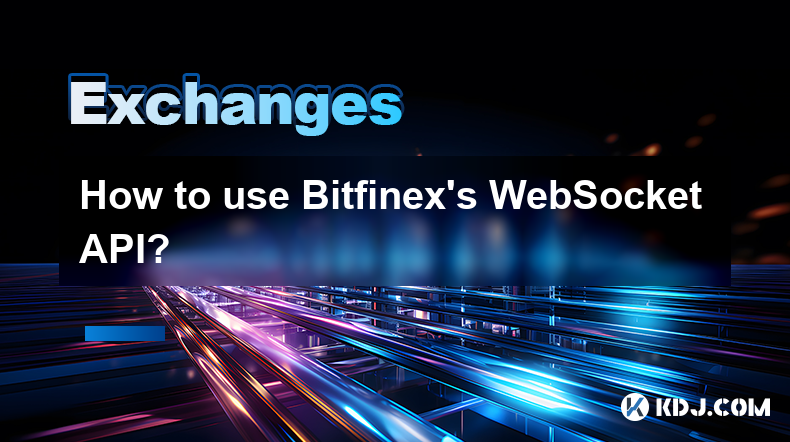
Using Bitfinex's WebSocket API involves several steps and considerations to effectively manage real-time data streams for cryptocurrency trading. This guide will walk you through the process, from establishing a connection to handling and interpreting the data you receive.
Establishing a Connection
To start using Bitfinex's WebSocket API, you first need to establish a connection to their WebSocket server. The server address for Bitfinex's WebSocket API is wss://api-pub.bitfinex.com/ws/2
.
Open a WebSocket connection to
wss://api-pub.bitfinex.com/ws/2
using your preferred programming language or library. For example, in JavaScript, you might use the WebSocket object:const ws = new WebSocket('wss://api-pub.bitfinex.com/ws/2');
Set up event listeners for the WebSocket connection to handle incoming messages, errors, and connection status changes. For instance:
ws.onmessage = (event) => {
console.log('Received:', event.data);
};ws.onerror = (error) => {
console.log('WebSocket Error:', error);
};ws.onclose = (event) => {
console.log('WebSocket Closed:', event);
};
Subscribing to Channels
Once the connection is established, you can subscribe to various channels to receive real-time data. Bitfinex offers several channels, including ticker, trades, and order book data.
Send a subscription message to the WebSocket server to start receiving data from a specific channel. For example, to subscribe to the ticker channel for the BTC/USD pair, you would send:
ws.send(JSON.stringify({
event: 'subscribe',
channel: 'ticker',
symbol: 'tBTCUSD'
}));Handle the subscription confirmation message from the server, which will include a channel ID that you can use to identify the data stream.
Receiving and Interpreting Data
After subscribing to a channel, you will start receiving data in real-time. It's important to understand the format of the data and how to interpret it.
Parse the incoming data to extract relevant information. For example, ticker data for the BTC/USD pair might look like this:
[12345, [35000.0, 0.1, 35001.0, 0.2, 10, 35000.0, 35001.0, '123456789']]
Here,
12345
is the channel ID, and the array contains the latest ticker information such as bid price, bid size, ask price, ask size, etc.Store and process the data according to your application's needs. You might want to update a user interface, trigger trading algorithms, or log the data for analysis.
Managing Subscriptions
You can manage your subscriptions by unsubscribing from channels when they are no longer needed or by subscribing to additional channels as required.
Unsubscribe from a channel by sending an unsubscribe message. For example, to unsubscribe from the ticker channel for BTC/USD:
ws.send(JSON.stringify({
event: 'unsubscribe',
chanId: 12345
}));Subscribe to multiple channels by sending multiple subscription messages. Each channel will have its own channel ID, which you need to keep track of.
Handling Errors and Disconnections
It's crucial to handle errors and disconnections gracefully to maintain a robust application.
Implement error handling to catch and respond to any errors that occur during the WebSocket connection. This might involve logging the error, notifying the user, or attempting to reconnect.
Set up a reconnection mechanism to automatically reconnect to the WebSocket server if the connection is lost. For example:
function reconnect() {
setTimeout(() => {ws = new WebSocket('wss://api-pub.bitfinex.com/ws/2'); // Set up event listeners again
}, 1000);
}ws.onclose = (event) => {
console.log('WebSocket Closed:', event);
reconnect();
};
Authenticating for Private Data
To access private data such as your account balances and orders, you need to authenticate your WebSocket connection.
Generate an authentication payload using your API key and secret. The payload should include a nonce, which is a unique number to prevent replay attacks.
Send the authentication message to the WebSocket server. For example:
const apiKey = 'your_api_key';
const apiSecret = 'your_api_secret';
const nonce = Date.now() * 1000;
const payload =AUTH${nonce}
;
const signature = crypto.createHmac('sha384', apiSecret).update(payload).digest('hex');ws.send(JSON.stringify({
event: 'auth',
apiKey: apiKey,
authSig: signature,
authPayload: payload,
authNonce: nonce
}));Handle the authentication response from the server, which will indicate whether the authentication was successful. If successful, you can then subscribe to private channels like account balances or order updates.
FAQs
Q: Can I use Bitfinex's WebSocket API for automated trading?
A: Yes, you can use Bitfinex's WebSocket API for automated trading by subscribing to real-time market data and sending trading commands through the authenticated WebSocket connection. Ensure you handle the data processing and trading logic in your application.
Q: How do I handle rate limits with Bitfinex's WebSocket API?
A: Bitfinex has rate limits in place to prevent abuse. You should monitor the rate at which you send requests and ensure you stay within the limits. If you exceed the rate limits, you might receive error messages, and you should implement a backoff strategy to reduce the frequency of your requests.
Q: What should I do if I encounter a WebSocket connection error?
A: If you encounter a WebSocket connection error, log the error for debugging purposes, notify the user if necessary, and attempt to reconnect to the WebSocket server. Implementing a robust reconnection mechanism can help maintain a stable connection.
Q: Is it possible to subscribe to multiple cryptocurrency pairs simultaneously?
A: Yes, you can subscribe to multiple cryptocurrency pairs by sending multiple subscription messages. Each subscription will have its own channel ID, and you can manage these subscriptions independently.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- SUI Price Surge Hits 60% as Solana Price Prediction Eyes New Highs, Unstaked at $0.0065 Joins Top Layer 1 Blockchain Leaders
- 2025-04-27 02:25:12
- Altcoin season is expected to kick off earlier than anticipated
- 2025-04-27 02:25:12
- XY Miners: The Ultimate Guide to Bitcoin Cloud Mining
- 2025-04-27 02:20:11
- Today's Focus Is on These Prospective Altcoins: Movement, eCash, Snek, and Arweave
- 2025-04-27 02:20:11
- Brazil Now Has the World's First Spot XRP ETF (XRPH11)
- 2025-04-27 02:15:13
- Best AI Crypto Coins to Buy Now as the Sector's Market Cap Reaches a New All-Time High
- 2025-04-27 02:15:13
Related knowledge
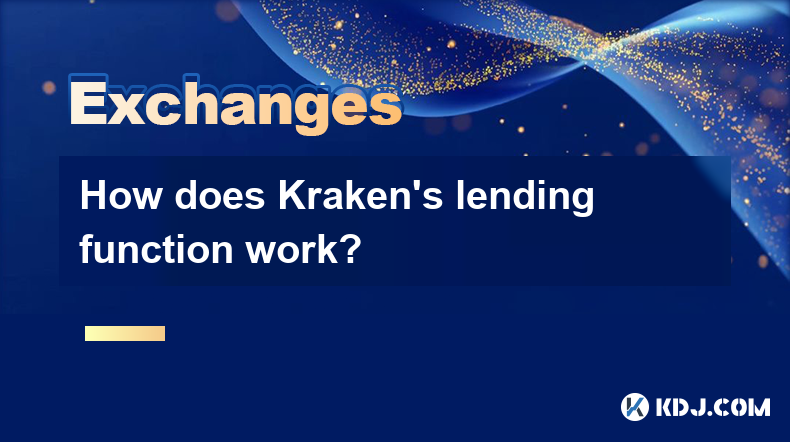
How does Kraken's lending function work?
Apr 25,2025 at 07:28pm
Kraken's lending function provides users with the opportunity to earn interest on their cryptocurrency holdings by lending them out to other users on the platform. This feature is designed to be user-friendly and secure, allowing both novice and experienced crypto enthusiasts to participate in the lending market. In this article, we will explore how Kra...
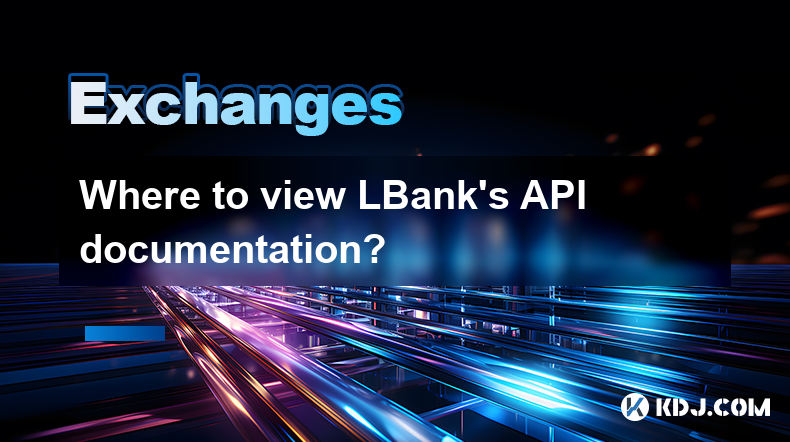
Where to view LBank's API documentation?
Apr 24,2025 at 06:21am
LBank is a popular cryptocurrency exchange that provides various services to its users, including trading, staking, and more. One of the essential resources for developers and advanced users is the API documentation, which allows them to interact with the platform programmatically. In this article, we will explore where to view LBank's API documentation...
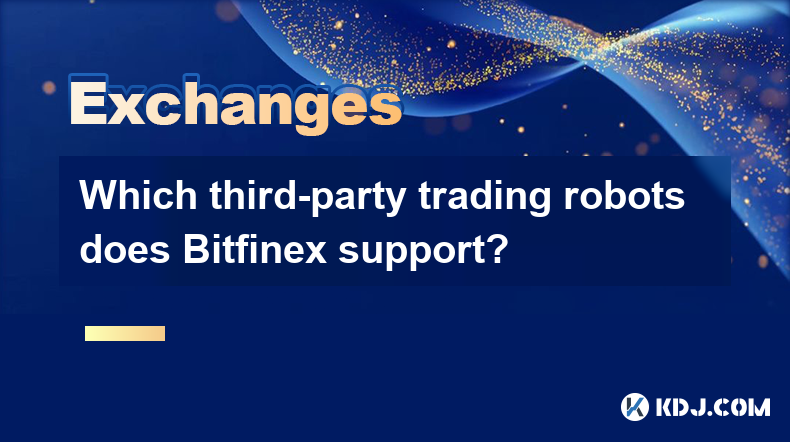
Which third-party trading robots does Bitfinex support?
Apr 24,2025 at 03:08am
Bitfinex, one of the leading cryptocurrency exchanges, supports a variety of third-party trading robots to enhance the trading experience of its users. These robots automate trading strategies, allowing traders to execute trades more efficiently and potentially increase their profits. In this article, we will explore the different third-party trading ro...
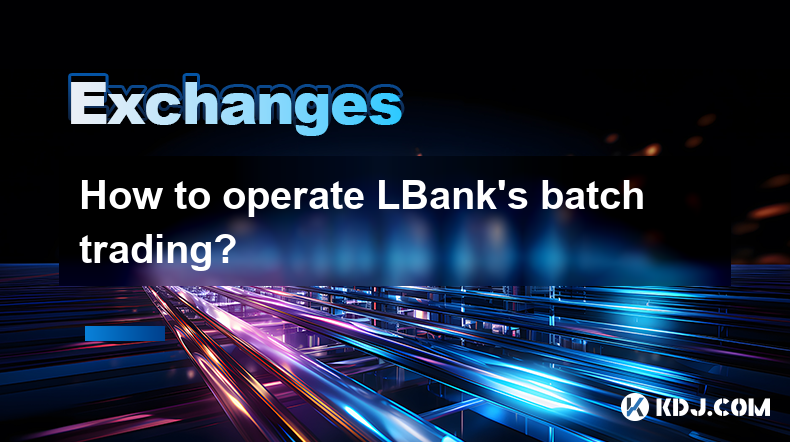
How to operate LBank's batch trading?
Apr 23,2025 at 01:15pm
LBank is a well-known cryptocurrency exchange that offers a variety of trading features to its users, including the option for batch trading. Batch trading allows users to execute multiple trades simultaneously, which can be particularly useful for those looking to manage a diverse portfolio or engage in arbitrage opportunities. In this article, we will...
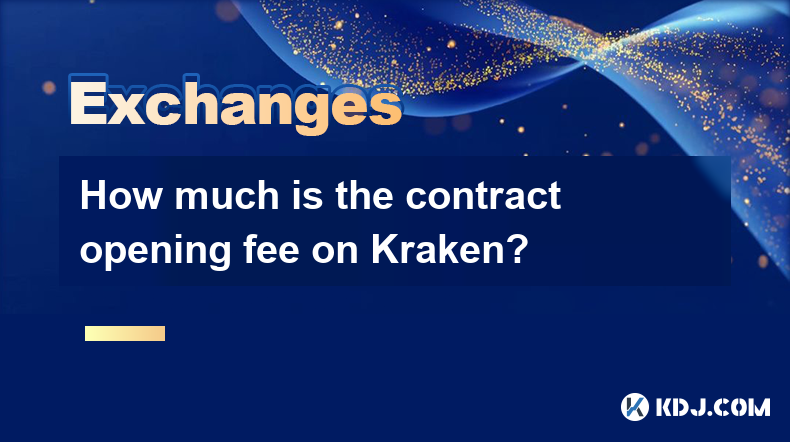
How much is the contract opening fee on Kraken?
Apr 23,2025 at 03:00pm
When engaging with cryptocurrency exchanges like Kraken, understanding the fee structure is crucial for managing trading costs effectively. One specific fee that traders often inquire about is the contract opening fee. On Kraken, this fee is associated with futures trading, which allows users to speculate on the future price of cryptocurrencies. Let's d...
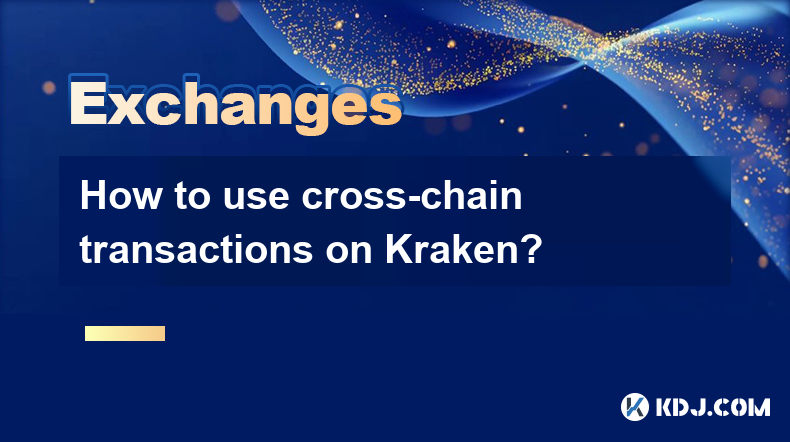
How to use cross-chain transactions on Kraken?
Apr 23,2025 at 12:50pm
Cross-chain transactions on Kraken allow users to transfer cryptocurrencies between different blockchain networks seamlessly. This feature is particularly useful for traders and investors looking to diversify their portfolios across various blockchains or to take advantage of specific opportunities on different networks. In this article, we will explore...
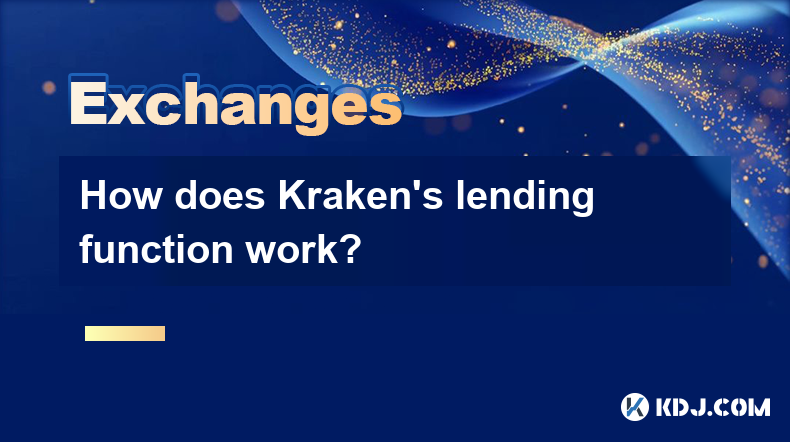
How does Kraken's lending function work?
Apr 25,2025 at 07:28pm
Kraken's lending function provides users with the opportunity to earn interest on their cryptocurrency holdings by lending them out to other users on the platform. This feature is designed to be user-friendly and secure, allowing both novice and experienced crypto enthusiasts to participate in the lending market. In this article, we will explore how Kra...
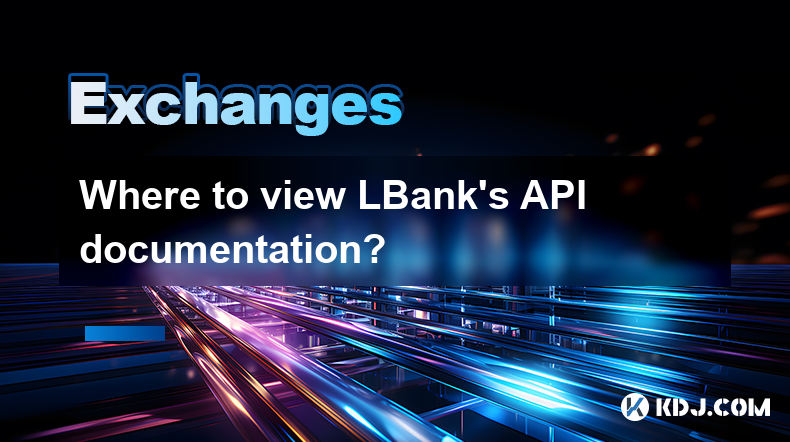
Where to view LBank's API documentation?
Apr 24,2025 at 06:21am
LBank is a popular cryptocurrency exchange that provides various services to its users, including trading, staking, and more. One of the essential resources for developers and advanced users is the API documentation, which allows them to interact with the platform programmatically. In this article, we will explore where to view LBank's API documentation...
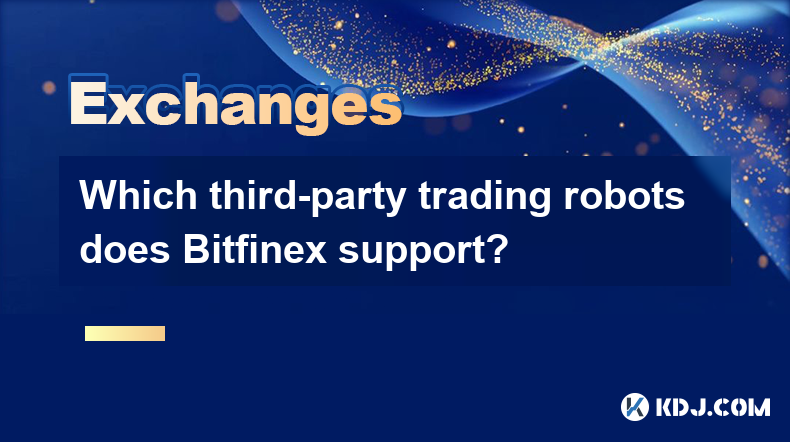
Which third-party trading robots does Bitfinex support?
Apr 24,2025 at 03:08am
Bitfinex, one of the leading cryptocurrency exchanges, supports a variety of third-party trading robots to enhance the trading experience of its users. These robots automate trading strategies, allowing traders to execute trades more efficiently and potentially increase their profits. In this article, we will explore the different third-party trading ro...
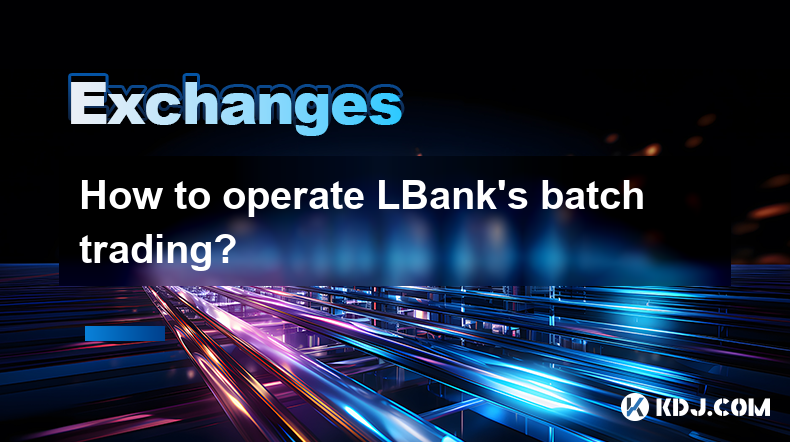
How to operate LBank's batch trading?
Apr 23,2025 at 01:15pm
LBank is a well-known cryptocurrency exchange that offers a variety of trading features to its users, including the option for batch trading. Batch trading allows users to execute multiple trades simultaneously, which can be particularly useful for those looking to manage a diverse portfolio or engage in arbitrage opportunities. In this article, we will...
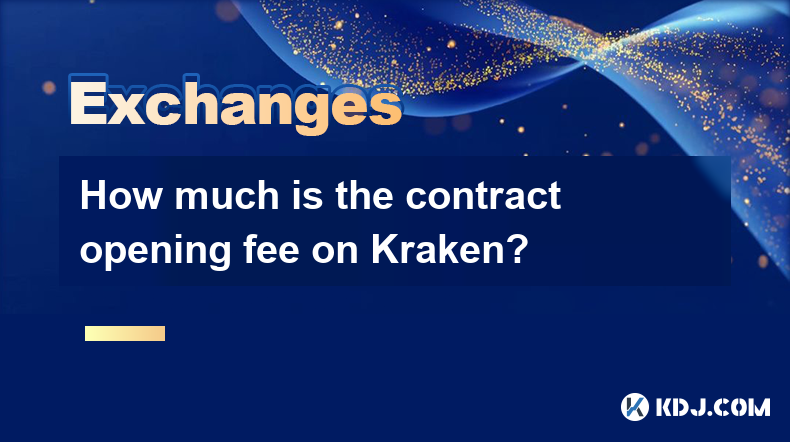
How much is the contract opening fee on Kraken?
Apr 23,2025 at 03:00pm
When engaging with cryptocurrency exchanges like Kraken, understanding the fee structure is crucial for managing trading costs effectively. One specific fee that traders often inquire about is the contract opening fee. On Kraken, this fee is associated with futures trading, which allows users to speculate on the future price of cryptocurrencies. Let's d...
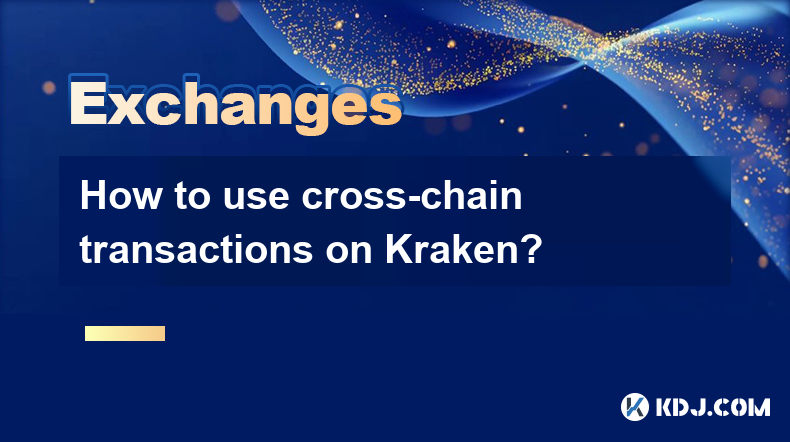
How to use cross-chain transactions on Kraken?
Apr 23,2025 at 12:50pm
Cross-chain transactions on Kraken allow users to transfer cryptocurrencies between different blockchain networks seamlessly. This feature is particularly useful for traders and investors looking to diversify their portfolios across various blockchains or to take advantage of specific opportunities on different networks. In this article, we will explore...
See all articles
