-
Bitcoin
$93,943.7603
-0.41% -
Ethereum
$1,773.2137
-1.80% -
Tether USDt
$0.9998
-0.01% -
XRP
$2.0961
-2.64% -
BNB
$594.6411
-0.06% -
Solana
$142.7596
-1.39% -
USDC
$1.0000
0.00% -
Dogecoin
$0.1659
-3.07% -
TRON
$0.2441
-1.84% -
Cardano
$0.6506
-2.54% -
Sui
$3.2012
-7.51% -
Chainlink
$13.3057
-3.56% -
Avalanche
$19.4416
-0.68% -
UNUS SED LEO
$8.7236
1.19% -
Stellar
$0.2548
-3.44% -
Toncoin
$2.9787
-0.37% -
Shiba Inu
$0.0...01244
-1.94% -
Hedera
$0.1702
-2.20% -
Bitcoin Cash
$352.4717
-0.56% -
Hyperliquid
$19.6286
-2.65% -
Litecoin
$81.9195
-7.52% -
Polkadot
$3.8566
-1.91% -
Dai
$1.0000
0.00% -
Monero
$287.3544
4.00% -
Bitget Token
$4.3075
0.19% -
Ethena USDe
$1.0001
-0.02% -
Pi
$0.5789
-2.25% -
Pepe
$0.0...07661
-4.14% -
Bittensor
$358.9078
-0.29% -
Uniswap
$4.8671
-2.68%
How to place orders using Bitfinex's API?
Bitfinex's API allows placing market, limit, and stop orders; set up Python environment, authenticate with API key, and manage orders effectively.
Apr 13, 2025 at 07:56 pm
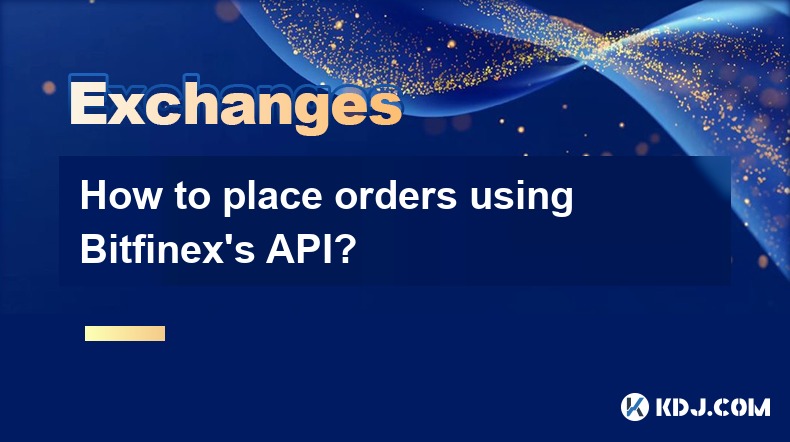
Using Bitfinex's API to place orders involves understanding the API's structure, setting up your environment, and executing the appropriate commands. This article will guide you through the process step-by-step, ensuring you can successfully place orders using Bitfinex's API.
Understanding Bitfinex's API
Bitfinex's API is a powerful tool that allows traders to interact with the exchange programmatically. The API supports various order types, including market orders, limit orders, and stop orders. It also provides endpoints for account management, trading, and retrieving market data. To use the API, you need to have an API key and secret, which you can generate from your Bitfinex account settings.
Setting Up Your Environment
Before you can start placing orders, you need to set up your development environment. Here's how you can do it:
- Install Python: Bitfinex's API can be used with various programming languages, but for this tutorial, we'll use Python. Download and install Python from the official website if you haven't already.
- Install the Bitfinex API library: Open a terminal or command prompt and run the following command to install the Bitfinex API library:
pip install bitfinex
- Generate API Key and Secret: Log into your Bitfinex account, navigate to the API section, and generate a new API key and secret. Make sure to save these securely, as you'll need them to authenticate your requests.
Authenticating with the API
To interact with Bitfinex's API, you need to authenticate your requests using your API key and secret. Here's how to do it in Python:
Import the necessary libraries:
from bitfinex import ClientV2
import timeInitialize the client:
api_key = 'your_api_key'
api_secret = 'your_api_secret'
client = ClientV2(api_key, api_secret)Test the connection:
try:
wallets = client.wallets()
print(wallets)
except Exception as e:
print(f"An error occurred: {e}")
Placing a Market Order
A market order is an order to buy or sell a cryptocurrency at the current market price. Here's how to place a market order using Bitfinex's API:
Define the order parameters:
symbol = 'tBTCUSD' # The trading pair
amount = '0.01' # The amount of BTC to buy
side = 'buy' # 'buy' or 'sell'Place the order:
try:
order = client.new_order(symbol=symbol, amount=amount, side=side, type='MARKET'
)
print(order)
except Exception as e:
print(f"An error occurred: {e}")
Placing a Limit Order
A limit order allows you to specify the price at which you want to buy or sell a cryptocurrency. Here's how to place a limit order:
Define the order parameters:
symbol = 'tBTCUSD'
amount = '0.01'
side = 'buy'
price = '30000' # The price at which you want to buyPlace the order:
try:
order = client.new_order(symbol=symbol, amount=amount, side=side, price=price, type='LIMIT'
)
print(order)
except Exception as e:
print(f"An error occurred: {e}")
Placing a Stop Order
A stop order is used to buy or sell a cryptocurrency when it reaches a specified price. Here's how to place a stop order:
Define the order parameters:
symbol = 'tBTCUSD'
amount = '0.01'
side = 'sell'
price = '35000' # The price at which you want to sellPlace the order:
try:
order = client.new_order(symbol=symbol, amount=amount, side=side, price=price, type='STOP'
)
print(order)
except Exception as e:
print(f"An error occurred: {e}")
Managing Orders
Once you've placed an order, you may need to manage it, such as canceling or modifying it. Here's how to do that:
Retrieve active orders:
try:
active_orders = client.active_orders()
print(active_orders)
except Exception as e:
print(f"An error occurred: {e}")Cancel an order:
order_id = 'your_order_id' # Replace with the actual order ID
try:
result = client.cancel_order(order_id)
print(result)
except Exception as e:
print(f"An error occurred: {e}")Modify an order:
order_id = 'your_order_id'
new_price = '31000' # New price for the order
try:
result = client.update_order(order_id=order_id, price=new_price
)
print(result)
except Exception as e:
print(f"An error occurred: {e}")
Retrieving Order History
To keep track of your trading activity, you can retrieve your order history using the following code:
- Retrieve order history:
try:
order_history = client.order_history()
print(order_history)
except Exception as e:
print(f"An error occurred: {e}")
Frequently Asked Questions
Q: Can I use Bitfinex's API with languages other than Python?
A: Yes, Bitfinex's API can be used with various programming languages, including JavaScript, Java, and C#. You'll need to use the appropriate library or SDK for your chosen language.
Q: How do I handle errors when using the API?
A: Bitfinex's API returns error codes and messages that you can use to handle errors. You should wrap your API calls in try-except blocks to catch and handle exceptions gracefully.
Q: Is there a limit to the number of orders I can place using the API?
A: Yes, Bitfinex has rate limits on API requests. You should check the official Bitfinex API documentation for the most current information on rate limits and how to manage them.
Q: Can I use the API to trade on margin?
A: Yes, Bitfinex's API supports margin trading. You can place margin orders by specifying the appropriate parameters in your order requests.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Bitcoin (BTC) Holds Above $94,000 as the Market Awaits the Fed's Decision
- 2025-05-06 20:15:12
- The Rise of Crypto Launchpads: How Pumpfun Dominates the Market and New Players Emerge
- 2025-05-06 20:15:12
- Hedera (HBAR) Holders Brace for Impact – Trillions Are Flooding On-Chain!
- 2025-05-06 20:10:14
- The internet has long promised freedom—freedom to connect, to create, and to share without boundaries.
- 2025-05-06 20:10:14
- VanEck Files to Launch the First-Ever Spot BNB ETF
- 2025-05-06 20:05:12
- The 4 Best Long-Term Crypto Opportunities in 2025: Web3 ai, Avalanche, Cardano, and Toncoin
- 2025-05-06 20:05:12
Related knowledge
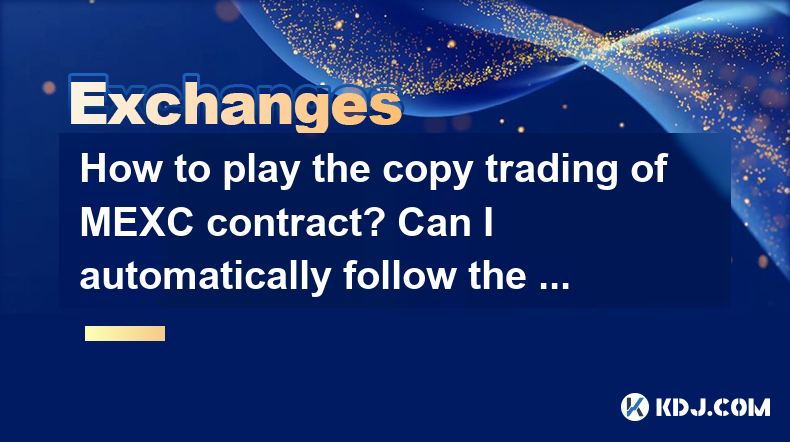
How to play the copy trading of MEXC contract? Can I automatically follow the operation of experts?
May 06,2025 at 06:29pm
Introduction to MEXC Copy TradingMEXC is a popular cryptocurrency exchange that offers a variety of trading options, including contract trading. One of the most appealing features of MEXC is its copy trading functionality, which allows users to automatically replicate the trades of experienced traders. This feature is particularly beneficial for beginne...
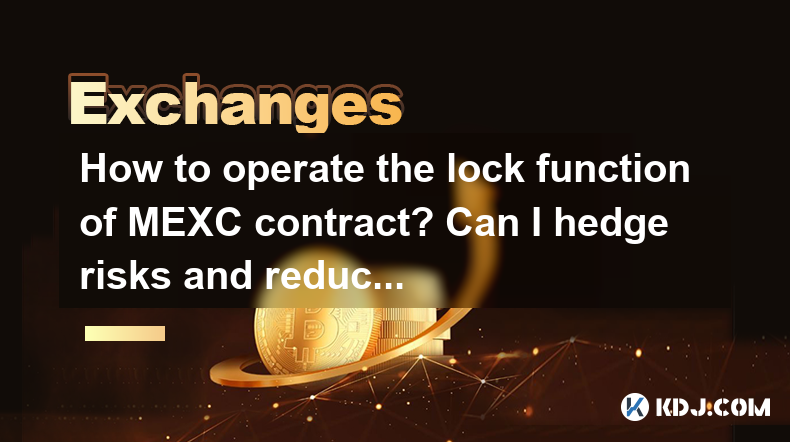
How to operate the lock function of MEXC contract? Can I hedge risks and reduce losses?
May 06,2025 at 07:28pm
Understanding the Lock Function on MEXC ContractThe lock function on MEXC, a prominent cryptocurrency exchange, is a feature designed to help traders manage their positions more effectively. Locking a position means that you temporarily prevent any changes to your current position, which can be particularly useful in volatile markets. This feature is cr...
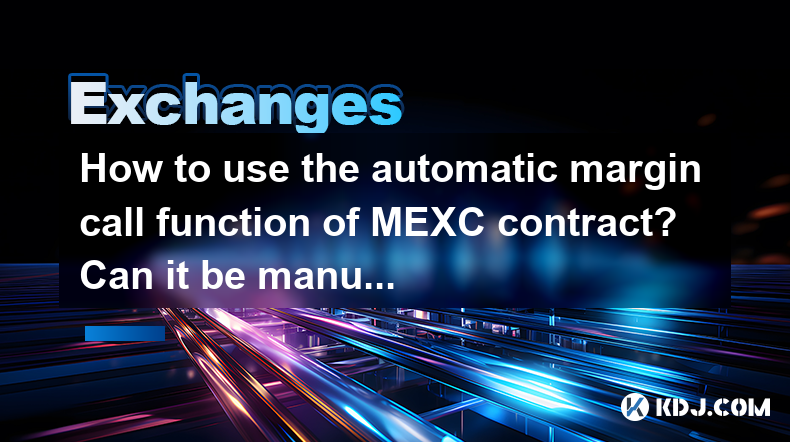
How to use the automatic margin call function of MEXC contract? Can it be manually canceled after triggering?
May 06,2025 at 06:15pm
Using the automatic margin call function of MEXC contract is an essential feature for traders looking to manage their risk effectively. This function helps maintain your position by automatically adding margin when your position is at risk of liquidation. In this article, we will delve into how to use this feature and whether it can be manually canceled...
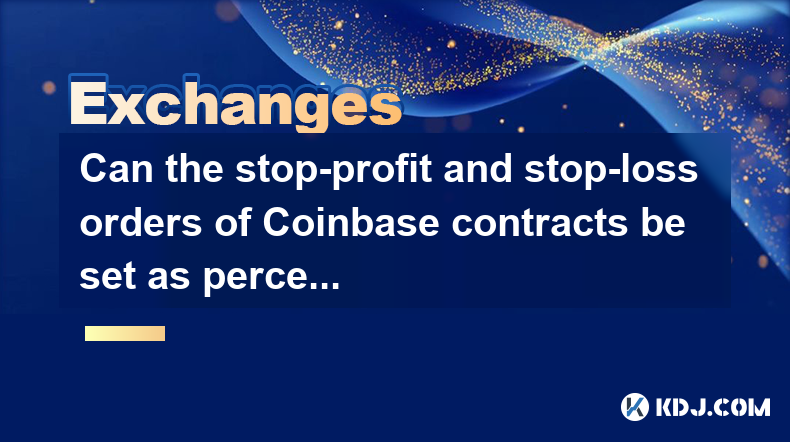
Can the stop-profit and stop-loss orders of Coinbase contracts be set as percentages? Or can only fixed prices be used?
May 06,2025 at 08:01pm
Understanding Stop-Profit and Stop-Loss Orders on CoinbaseStop-profit and stop-loss orders are essential tools for traders looking to manage risk and secure profits in the volatile cryptocurrency market. These orders allow traders to automatically sell or buy an asset when it reaches a specific price level, helping to mitigate losses and lock in gains. ...
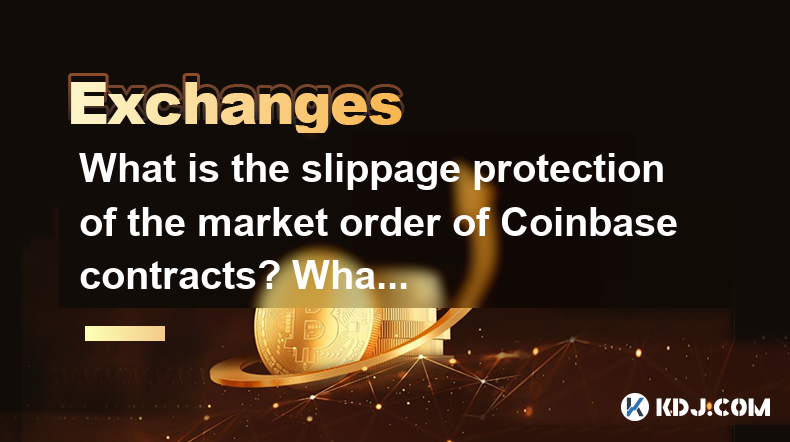
What is the slippage protection of the market order of Coinbase contracts? What are the triggering conditions?
May 06,2025 at 06:49pm
In the world of cryptocurrency trading, understanding the mechanics of market orders and their associated features like slippage protection is crucial for traders. Coinbase, a leading cryptocurrency exchange, offers various trading options, including market orders for its contracts. This article delves into the specifics of the slippage protection featu...
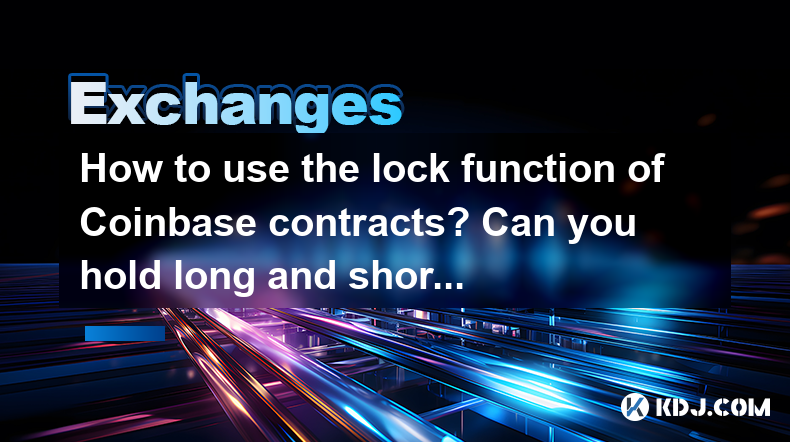
How to use the lock function of Coinbase contracts? Can you hold long and short bidirectional positions at the same time?
May 06,2025 at 05:14pm
Introduction to Coinbase ContractsCoinbase, one of the leading cryptocurrency exchanges, offers a variety of financial instruments to its users, including futures contracts. These contracts allow traders to speculate on the future price of cryptocurrencies. One of the key features of Coinbase's futures contracts is the lock function, which can be used t...
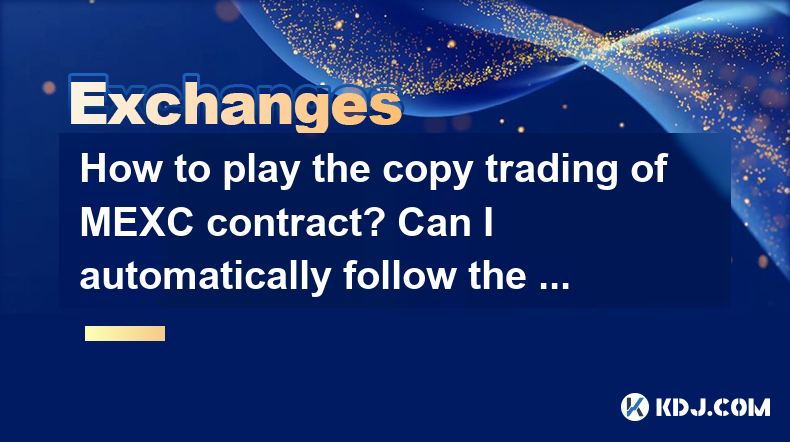
How to play the copy trading of MEXC contract? Can I automatically follow the operation of experts?
May 06,2025 at 06:29pm
Introduction to MEXC Copy TradingMEXC is a popular cryptocurrency exchange that offers a variety of trading options, including contract trading. One of the most appealing features of MEXC is its copy trading functionality, which allows users to automatically replicate the trades of experienced traders. This feature is particularly beneficial for beginne...
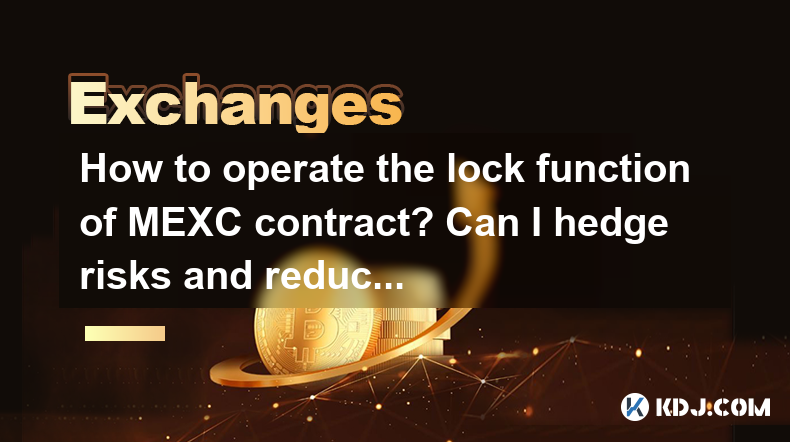
How to operate the lock function of MEXC contract? Can I hedge risks and reduce losses?
May 06,2025 at 07:28pm
Understanding the Lock Function on MEXC ContractThe lock function on MEXC, a prominent cryptocurrency exchange, is a feature designed to help traders manage their positions more effectively. Locking a position means that you temporarily prevent any changes to your current position, which can be particularly useful in volatile markets. This feature is cr...
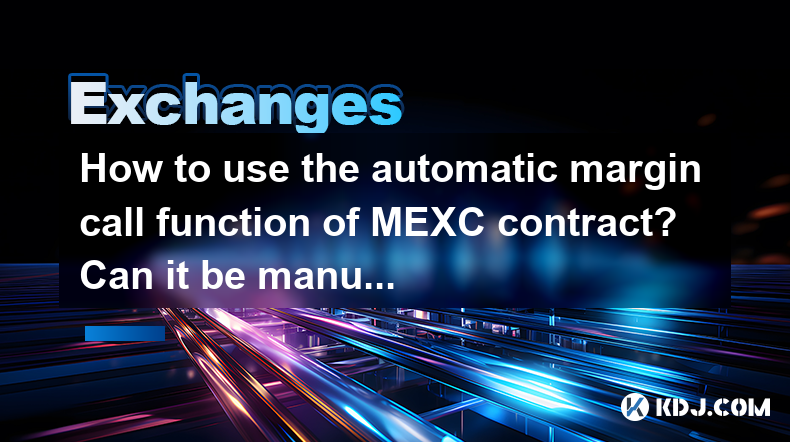
How to use the automatic margin call function of MEXC contract? Can it be manually canceled after triggering?
May 06,2025 at 06:15pm
Using the automatic margin call function of MEXC contract is an essential feature for traders looking to manage their risk effectively. This function helps maintain your position by automatically adding margin when your position is at risk of liquidation. In this article, we will delve into how to use this feature and whether it can be manually canceled...
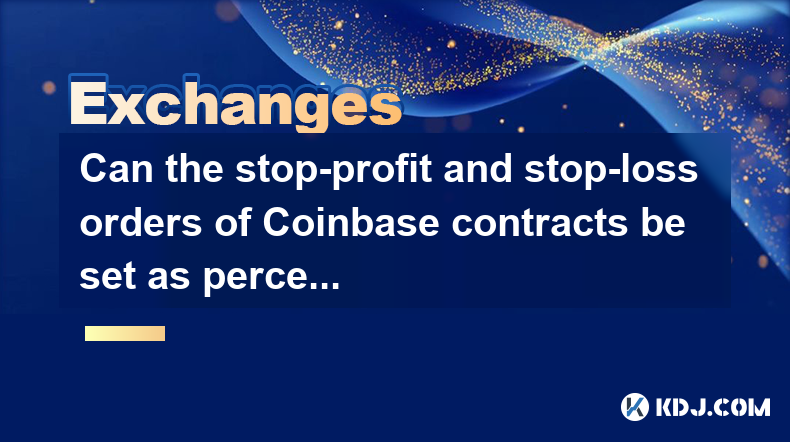
Can the stop-profit and stop-loss orders of Coinbase contracts be set as percentages? Or can only fixed prices be used?
May 06,2025 at 08:01pm
Understanding Stop-Profit and Stop-Loss Orders on CoinbaseStop-profit and stop-loss orders are essential tools for traders looking to manage risk and secure profits in the volatile cryptocurrency market. These orders allow traders to automatically sell or buy an asset when it reaches a specific price level, helping to mitigate losses and lock in gains. ...
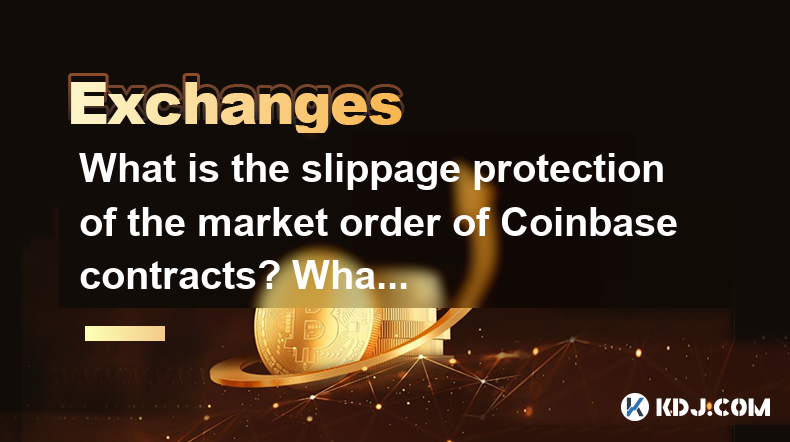
What is the slippage protection of the market order of Coinbase contracts? What are the triggering conditions?
May 06,2025 at 06:49pm
In the world of cryptocurrency trading, understanding the mechanics of market orders and their associated features like slippage protection is crucial for traders. Coinbase, a leading cryptocurrency exchange, offers various trading options, including market orders for its contracts. This article delves into the specifics of the slippage protection featu...
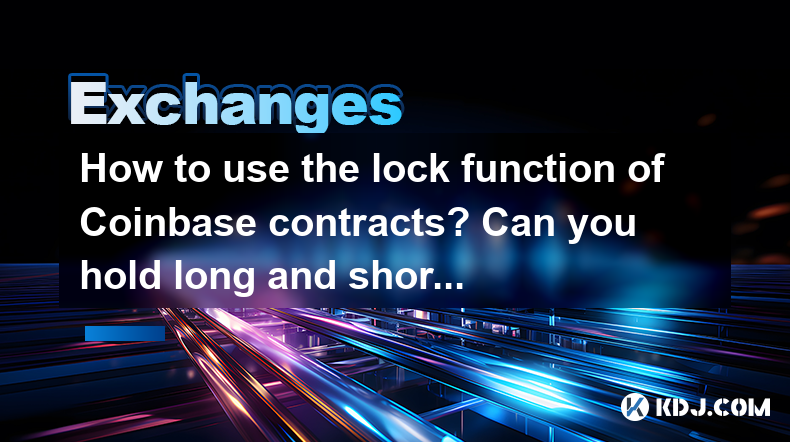
How to use the lock function of Coinbase contracts? Can you hold long and short bidirectional positions at the same time?
May 06,2025 at 05:14pm
Introduction to Coinbase ContractsCoinbase, one of the leading cryptocurrency exchanges, offers a variety of financial instruments to its users, including futures contracts. These contracts allow traders to speculate on the future price of cryptocurrencies. One of the key features of Coinbase's futures contracts is the lock function, which can be used t...
See all articles
