-
Bitcoin
$103,838.1932
1.42% -
Ethereum
$2,672.1709
9.17% -
XRP
$2.5784
4.18% -
Tether USDt
$0.9998
-0.03% -
Solana
$181.9841
7.49% -
BNB
$662.9837
2.49% -
USDC
$0.9997
-0.04% -
Dogecoin
$0.2447
10.14% -
Cardano
$0.8270
5.29% -
TRON
$0.2743
4.89% -
Sui
$4.0119
2.82% -
Chainlink
$17.3332
6.45% -
Avalanche
$26.3756
9.64% -
Stellar
$0.3123
3.11% -
Shiba Inu
$0.0...01642
8.36% -
Pi
$1.2793
17.94% -
Hedera
$0.2139
4.58% -
Hyperliquid
$25.8599
8.65% -
Toncoin
$3.4142
4.99% -
Polkadot
$5.2208
6.17% -
Bitcoin Cash
$409.8938
3.43% -
UNUS SED LEO
$8.7926
2.27% -
Litecoin
$104.3510
2.69% -
Monero
$348.8959
4.21% -
Pepe
$0.0...01435
9.17% -
Bitget Token
$4.8554
3.83% -
Dai
$0.9999
-0.02% -
Ethena USDe
$1.0006
0.00% -
Uniswap
$6.9351
4.35% -
Bittensor
$468.0684
4.27%
How to use the Crypto.com contract API? What programming languages are supported?
The Crypto.com Contract API enables developers to interact with smart contracts and manage tokens on the Crypto.com blockchain using languages like JavaScript, Python, Java, and Go.
May 05, 2025 at 08:43 pm
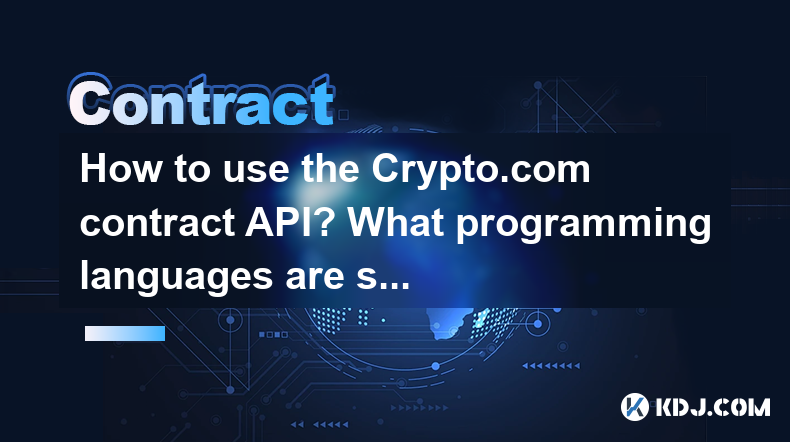
Introduction to Crypto.com Contract API
The Crypto.com Contract API is a powerful tool designed for developers looking to interact with the Crypto.com ecosystem programmatically. This API allows users to execute smart contracts, manage tokens, and perform various other blockchain operations directly from their applications. Understanding how to use this API effectively can greatly enhance the functionality of any crypto-related project. In this article, we will explore the steps needed to utilize the Crypto.com Contract API, as well as the programming languages that are supported for its use.
Getting Started with the Crypto.com Contract API
To begin using the Crypto.com Contract API, you first need to set up an account on the Crypto.com platform and obtain the necessary API keys. These keys are crucial for authenticating your requests and ensuring that only authorized users can interact with the smart contracts.
- Sign up for a Crypto.com account if you haven't already.
- Navigate to the API section within your account settings and generate your API keys. You will receive both a public key and a private key, which should be kept secure.
- Familiarize yourself with the API documentation provided by Crypto.com. This documentation includes detailed instructions on how to structure your API requests, the endpoints available, and the expected responses.
Supported Programming Languages
The Crypto.com Contract API supports a variety of programming languages to cater to different developer preferences. Some of the most commonly used languages include:
- JavaScript: Ideal for web-based applications and Node.js environments.
- Python: Popular among data scientists and developers who prefer a more readable syntax.
- Java: Suitable for enterprise-level applications and Android development.
- Go: Known for its efficiency and concurrency support, making it perfect for high-performance applications.
Each of these languages has its own set of libraries and SDKs provided by Crypto.com, which can simplify the process of integrating the Contract API into your project.
Setting Up Your Development Environment
Before you can start making API calls, you need to set up your development environment with the appropriate tools and libraries. Here’s how you can do it for some of the supported languages:
For JavaScript:
- Install Node.js if you haven't already.
- Use npm to install the Crypto.com SDK:
npm install @crypto-com/chain-sdk
. - Import the SDK in your project:
const { CryptoComChain } = require('@crypto-com/chain-sdk');
.
For Python:
- Ensure you have Python installed on your system.
- Install the Crypto.com SDK using pip:
pip install cryptocom-chain-sdk
. - Import the SDK in your script:
from cryptocom_chain_sdk import CryptoComChain
.
For Java:
- Set up your Java development environment with an IDE like IntelliJ IDEA or Eclipse.
- Add the Crypto.com SDK to your project's dependencies. If you're using Maven, you can add the following to your
pom.xml
:<groupId>com.crypto.com</groupId> <artifactId>chain-sdk</artifactId> <version>latest-version</version>
- Import the necessary classes in your Java code:
import com.cryptocom.chain.sdk.CryptoComChain;
.
For Go:
- Install Go on your system if you haven't already.
- Use Go's package manager to install the Crypto.com SDK:
go get github.com/crypto-com/chain-sdk-go
. - Import the SDK in your Go project:
import "github.com/crypto-com/chain-sdk-go/chain"
.
Making API Calls with the Crypto.com Contract API
Once your environment is set up, you can start making API calls to interact with smart contracts on the Crypto.com blockchain. Here’s a general process for making an API call:
- Initialize the SDK: Use the API keys you generated earlier to initialize the SDK.
- Connect to the Network: Establish a connection to the Crypto.com blockchain network.
- Prepare the Request: Construct your API request according to the documentation. This may involve specifying the contract address, function to call, and any necessary arguments.
- Send the Request: Execute the API call and handle the response accordingly.
Here is a simple example of how to call a smart contract function using JavaScript:
const { CryptoComChain } = require('@crypto-com/chain-sdk');// Initialize the SDK with your API keys
const chain = new CryptoComChain({
apiKey: 'your_api_key',
apiSecret: 'your_api_secret'
});
// Connect to the Crypto.com blockchain network
chain.connect();
// Define the smart contract address and function to call
const contractAddress = '0xYourContractAddress';
const functionName = 'yourFunctionName';
const args = ['arg1', 'arg2'];
// Call the smart contract function
chain.callSmartContract(contractAddress, functionName, args)
.then(response => {
console.log('Smart contract call successful:', response);
})
.catch(error => {
console.error('Error calling smart contract:', error);
});
Handling Responses and Errors
When working with the Crypto.com Contract API, it’s important to handle responses and errors appropriately. The API will return responses in JSON format, which can be parsed and processed within your application. Common response fields include status
, message
, and data
, which you should check to determine the success or failure of your API call.
- Check the Status: The
status
field will indicate whether the call was successful or not. A status of 200
typically means success. - Parse the Data: If the call was successful, the
data
field will contain the results of your smart contract execution. - Handle Errors: If an error occurs, the
message
field will provide details about what went wrong. Make sure to log these errors and handle them gracefully in your application.
Security Considerations
When using the Crypto.com Contract API, security should be a top priority. Here are some best practices to keep in mind:
- Secure Your API Keys: Never expose your API keys in your source code or share them with anyone. Use environment variables or a secure vault to store them.
- Validate Inputs: Always validate and sanitize any user inputs before passing them to the API to prevent injection attacks.
- Use HTTPS: Ensure that all communications with the Crypto.com API are done over HTTPS to prevent man-in-the-middle attacks.
- Rate Limiting: Be aware of the rate limits imposed by the Crypto.com API and implement appropriate throttling in your application to avoid being blocked.
Frequently Asked Questions
Q: Can I use the Crypto.com Contract API to create new smart contracts?
A: Yes, the Crypto.com Contract API allows you to deploy new smart contracts onto the Crypto.com blockchain. You will need to prepare the contract bytecode and use the appropriate API endpoint to deploy it.
Q: Are there any costs associated with using the Crypto.com Contract API?
A: Yes, interacting with the Crypto.com blockchain, including executing smart contract functions, may incur transaction fees. These fees are typically paid in the native cryptocurrency of the Crypto.com chain.
Q: How can I test my smart contract interactions before going live?
A: Crypto.com provides a testnet environment where you can deploy and interact with smart contracts without risking real funds. Use the testnet API endpoints to test your application before deploying to the mainnet.
Q: Is there a community or support forum for developers using the Crypto.com Contract API?
A: Yes, Crypto.com has an active developer community and support forums where you can ask questions, share experiences, and get help with any issues you encounter while using the Contract API.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- Bitcoin price is regaining new bullish momentum after filling a key CME gap
- 2025-05-14 14:05:13
- Investors Jumped on Shares of Coinbase Global (COIN) After the Monday Night News That the Crypto Exchange Will Join the S&P 500 Next Week.
- 2025-05-14 14:05:13
- Tether's USDT market cap has surpassed $150 billion
- 2025-05-14 14:00:24
- Meme Coin FloppyPepe (FPPE) Promises Life-Changing Returns, ChatGPT Picks It to Turn $1500 into $2M
- 2025-05-14 14:00:24
- It's Time To Build Some Star Wars Partners This Week In Monopoly Go
- 2025-05-14 13:55:13
- Arizona Rejects Bitcoin Reserve Proposal, Marking a Key Decision in the State's Digital Asset Policy
- 2025-05-14 13:55:13
Related knowledge
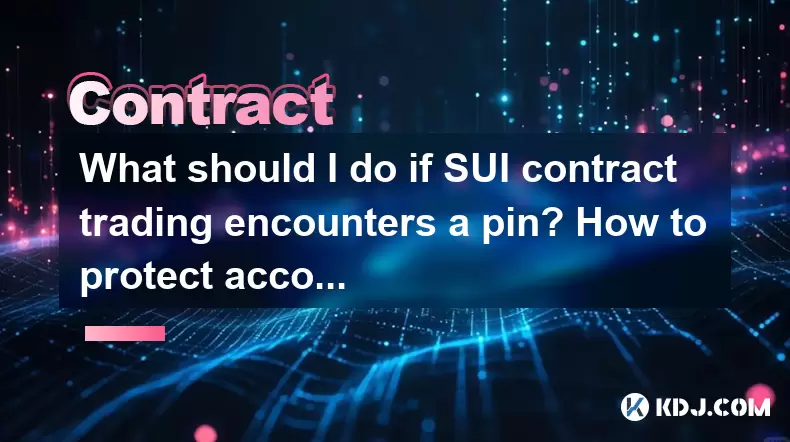
What should I do if SUI contract trading encounters a pin? How to protect account security?
May 09,2025 at 01:56am
When trading SUI contracts, encountering a pin can be a frustrating experience, but there are effective strategies to handle such situations and ensure your account remains secure. This article will guide you through the steps to manage a pin in SUI contract trading and provide detailed advice on protecting your account security. Understanding What a Pi...
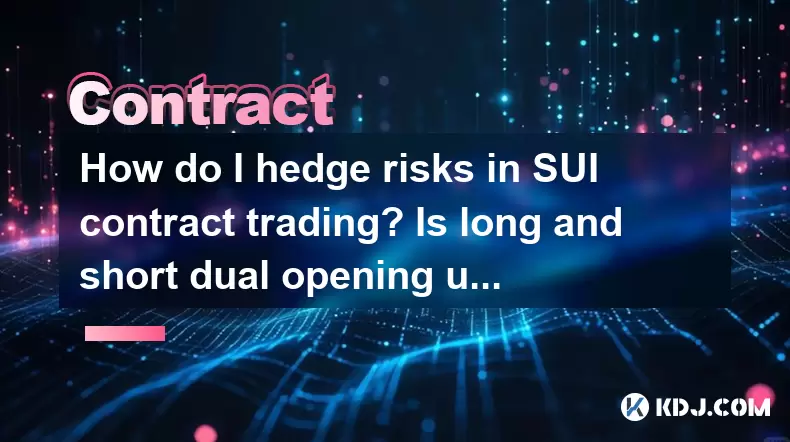
How do I hedge risks in SUI contract trading? Is long and short dual opening useful?
May 08,2025 at 09:14pm
Trading SUI contracts, like any other cryptocurrency derivative, involves significant risk due to the volatile nature of the market. Hedging is a strategy traders use to mitigate potential losses. In this article, we will explore various methods to hedge risks in SUI contract trading and evaluate the effectiveness of long and short dual opening as a hed...
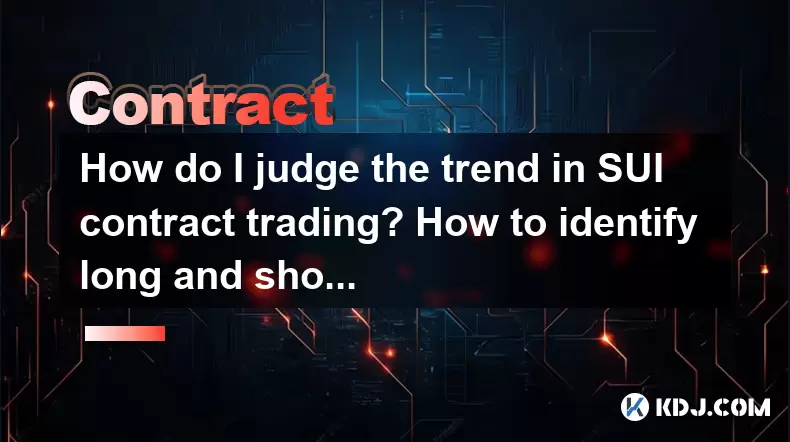
How do I judge the trend in SUI contract trading? How to identify long and short signals?
May 09,2025 at 01:49pm
Understanding SUI Contract TradingSUI contract trading involves trading contracts based on the SUI cryptocurrency, which is a relatively new entrant in the crypto market. To effectively trade these contracts, it's crucial to understand how to judge the market trend and identify long and short signals. This article will guide you through the process of a...
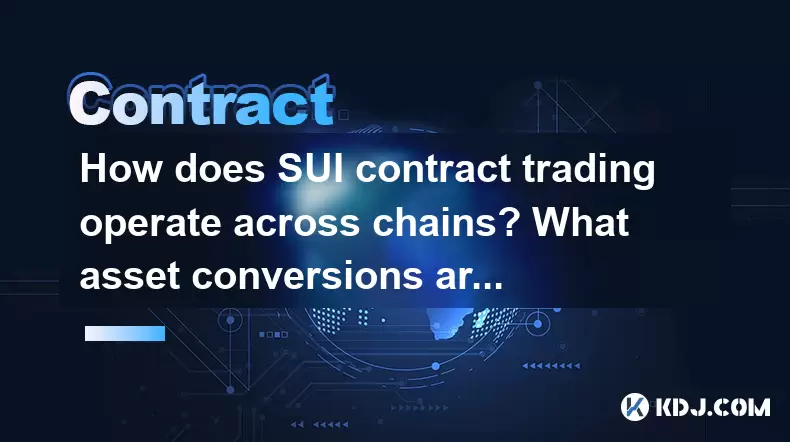
How does SUI contract trading operate across chains? What asset conversions are supported?
May 12,2025 at 02:56am
Introduction to SUI Contract Trading Across ChainsSUI contract trading is a sophisticated mechanism that allows users to engage in decentralized trading across multiple blockchain networks. This process involves the use of smart contracts to facilitate trades, ensuring that transactions are secure, transparent, and efficient. The ability to trade across...
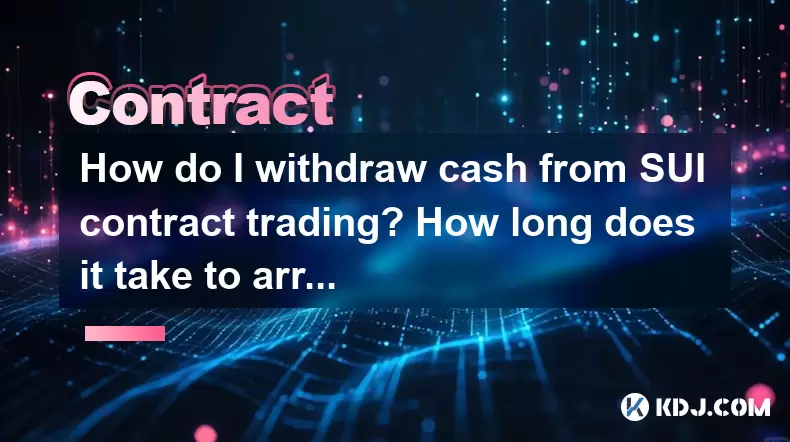
How do I withdraw cash from SUI contract trading? How long does it take to arrive?
May 09,2025 at 02:00am
Introduction to SUI Contract TradingSUI is a popular cryptocurrency that has gained traction in the decentralized finance (DeFi) space due to its efficient transaction processing and smart contract capabilities. Contract trading on the SUI network involves engaging in futures or options contracts based on the SUI token's price movements. When it comes t...
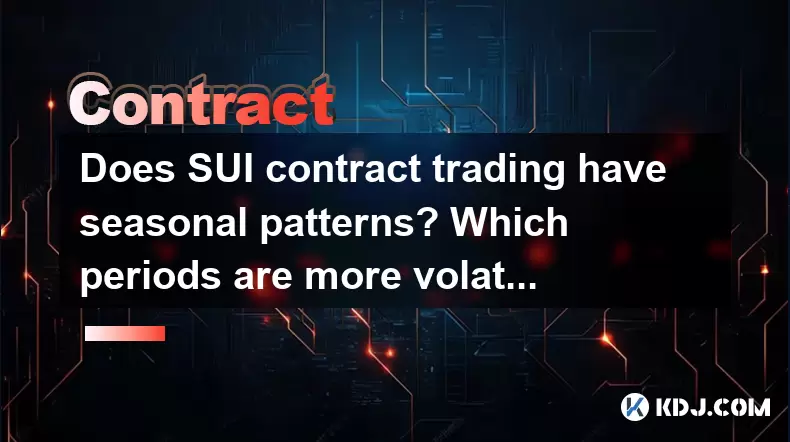
Does SUI contract trading have seasonal patterns? Which periods are more volatile?
May 10,2025 at 11:50pm
The SUI cryptocurrency, like many other digital assets, exhibits certain seasonal patterns in its trading activity. Understanding these patterns can be crucial for traders looking to capitalize on volatility and potential price movements. In this article, we will explore whether SUI contract trading has seasonal patterns, identify the periods that are m...
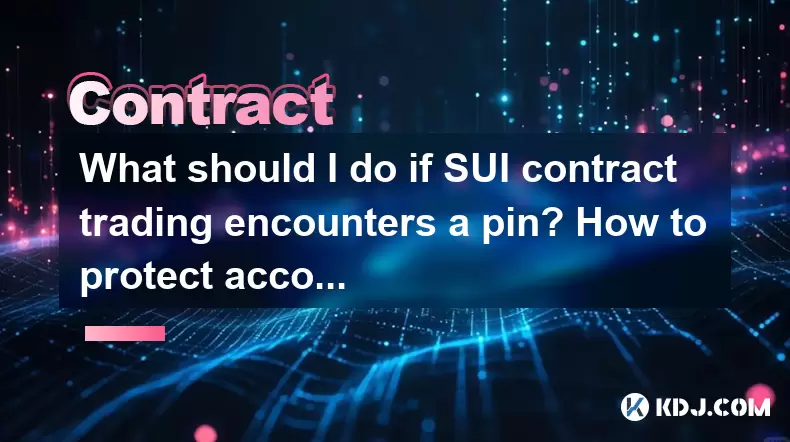
What should I do if SUI contract trading encounters a pin? How to protect account security?
May 09,2025 at 01:56am
When trading SUI contracts, encountering a pin can be a frustrating experience, but there are effective strategies to handle such situations and ensure your account remains secure. This article will guide you through the steps to manage a pin in SUI contract trading and provide detailed advice on protecting your account security. Understanding What a Pi...
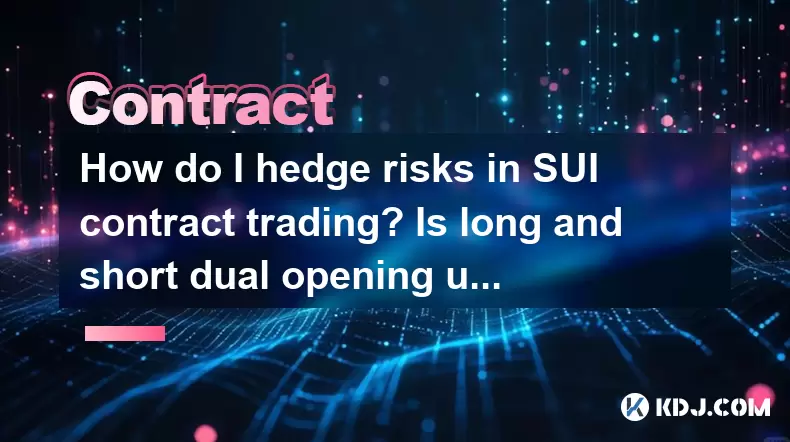
How do I hedge risks in SUI contract trading? Is long and short dual opening useful?
May 08,2025 at 09:14pm
Trading SUI contracts, like any other cryptocurrency derivative, involves significant risk due to the volatile nature of the market. Hedging is a strategy traders use to mitigate potential losses. In this article, we will explore various methods to hedge risks in SUI contract trading and evaluate the effectiveness of long and short dual opening as a hed...
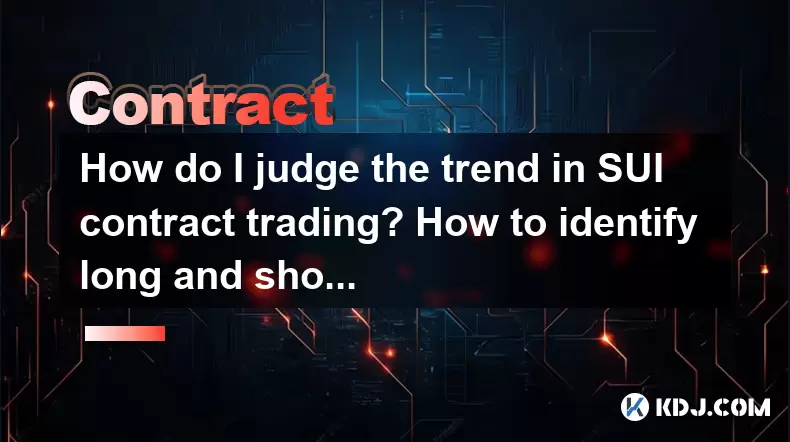
How do I judge the trend in SUI contract trading? How to identify long and short signals?
May 09,2025 at 01:49pm
Understanding SUI Contract TradingSUI contract trading involves trading contracts based on the SUI cryptocurrency, which is a relatively new entrant in the crypto market. To effectively trade these contracts, it's crucial to understand how to judge the market trend and identify long and short signals. This article will guide you through the process of a...
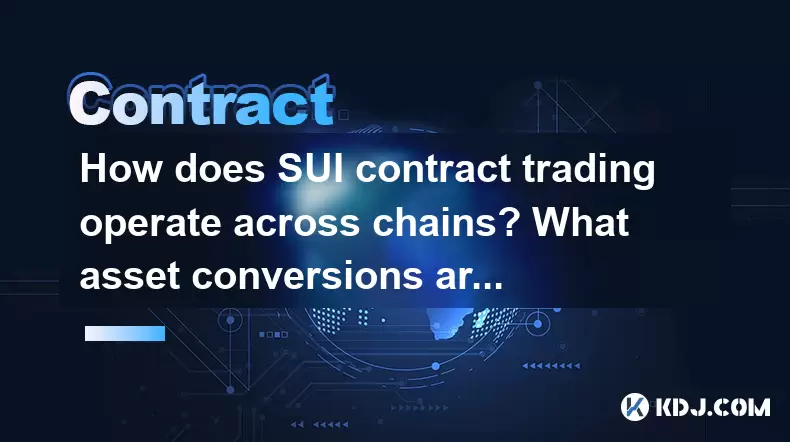
How does SUI contract trading operate across chains? What asset conversions are supported?
May 12,2025 at 02:56am
Introduction to SUI Contract Trading Across ChainsSUI contract trading is a sophisticated mechanism that allows users to engage in decentralized trading across multiple blockchain networks. This process involves the use of smart contracts to facilitate trades, ensuring that transactions are secure, transparent, and efficient. The ability to trade across...
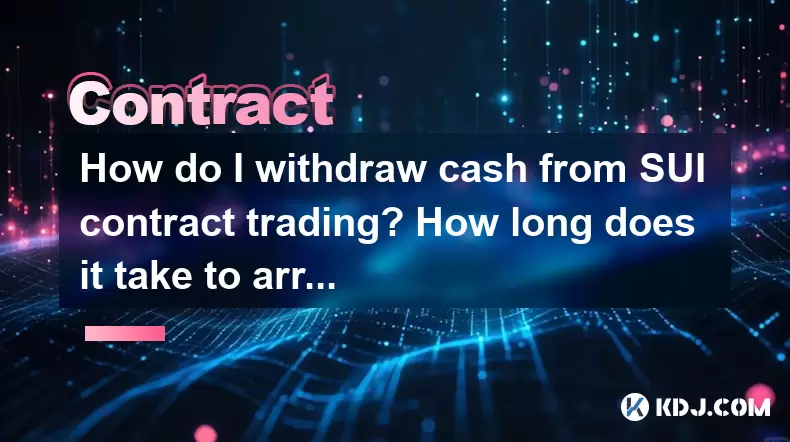
How do I withdraw cash from SUI contract trading? How long does it take to arrive?
May 09,2025 at 02:00am
Introduction to SUI Contract TradingSUI is a popular cryptocurrency that has gained traction in the decentralized finance (DeFi) space due to its efficient transaction processing and smart contract capabilities. Contract trading on the SUI network involves engaging in futures or options contracts based on the SUI token's price movements. When it comes t...
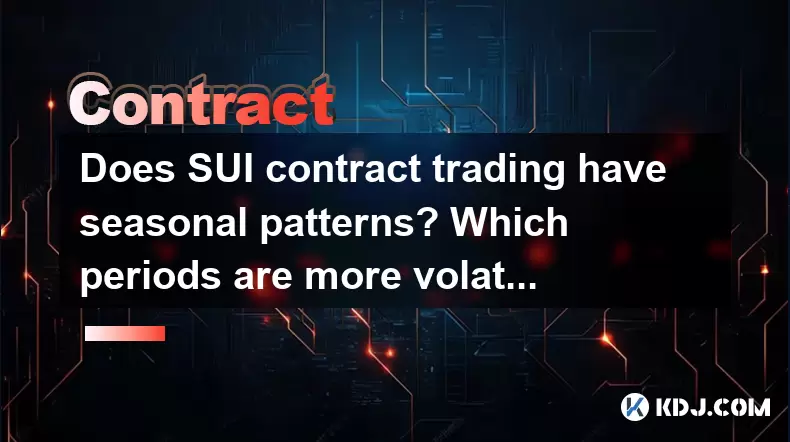
Does SUI contract trading have seasonal patterns? Which periods are more volatile?
May 10,2025 at 11:50pm
The SUI cryptocurrency, like many other digital assets, exhibits certain seasonal patterns in its trading activity. Understanding these patterns can be crucial for traders looking to capitalize on volatility and potential price movements. In this article, we will explore whether SUI contract trading has seasonal patterns, identify the periods that are m...
See all articles
