-
Bitcoin
$106,754.6083
1.33% -
Ethereum
$2,625.8249
3.80% -
Tether USDt
$1.0001
-0.03% -
XRP
$2.1891
1.67% -
BNB
$654.5220
0.66% -
Solana
$156.9428
7.28% -
USDC
$0.9998
0.00% -
Dogecoin
$0.1780
1.14% -
TRON
$0.2706
-0.16% -
Cardano
$0.6470
2.77% -
Hyperliquid
$44.6467
10.24% -
Sui
$3.1128
3.86% -
Bitcoin Cash
$455.7646
3.00% -
Chainlink
$13.6858
4.08% -
UNUS SED LEO
$9.2682
0.21% -
Avalanche
$19.7433
3.79% -
Stellar
$0.2616
1.64% -
Toncoin
$3.0222
2.19% -
Shiba Inu
$0.0...01220
1.49% -
Hedera
$0.1580
2.75% -
Litecoin
$87.4964
2.29% -
Polkadot
$3.8958
3.05% -
Ethena USDe
$1.0000
-0.04% -
Monero
$317.2263
0.26% -
Bitget Token
$4.5985
1.68% -
Dai
$0.9999
0.00% -
Pepe
$0.0...01140
2.44% -
Uniswap
$7.6065
5.29% -
Pi
$0.6042
-2.00% -
Aave
$289.6343
6.02%
What is Solidity and its smart contract development?
Solidity, designed for Ethereum, enables smart contracts that run on the EVM, supporting features like inheritance and libraries for decentralized app development.
Apr 12, 2025 at 02:22 pm
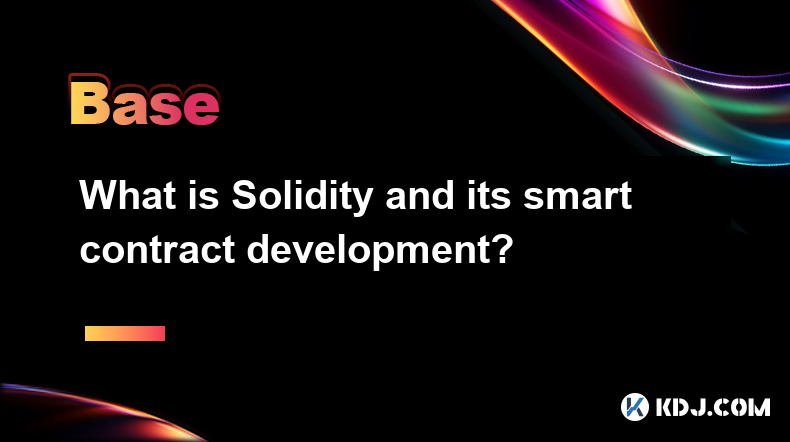
Solidity is a high-level, contract-oriented programming language specifically designed for writing smart contracts on blockchain platforms, most notably Ethereum. It is statically typed and supports inheritance, libraries, and complex user-defined types, among other features. Solidity is used to implement smart contracts that run on the Ethereum Virtual Machine (EVM), enabling developers to create decentralized applications (DApps) and other blockchain-based solutions.
What is a Smart Contract?
A smart contract is a self-executing contract with the terms of the agreement directly written into code. It automatically enforces and executes the terms of a contract when predefined conditions are met. Smart contracts run on blockchain networks, ensuring transparency, immutability, and security. They eliminate the need for intermediaries, reducing costs and increasing efficiency.
Key Features of Solidity
Solidity offers several key features that make it a preferred choice for smart contract development:
- Statically Typed: Solidity is a statically typed language, which means that variable types are known at compile time. This helps catch errors early in the development process.
- Inheritance: Solidity supports inheritance, allowing developers to create complex contract structures by reusing code.
- Libraries: Developers can use libraries to share code across multiple contracts, enhancing modularity and reducing redundancy.
- Complex User-Defined Types: Solidity allows the creation of complex data structures, which are essential for building sophisticated smart contracts.
Writing a Smart Contract in Solidity
To write a smart contract in Solidity, you need to follow a series of steps. Here's a detailed guide on how to create a simple smart contract:
- Install the Solidity Compiler: First, you need to install the Solidity compiler, known as
solc
. You can do this using npm by running the commandnpm install -g solc
. - Set Up a Development Environment: Choose a development environment like Remix, Truffle, or Hardhat. For beginners, Remix is recommended as it's a web-based IDE that doesn't require local setup.
- Write the Contract: Open your chosen development environment and create a new file with a
.sol
extension. Here's an example of a simple smart contract:
// SPDX-License-Identifier: MIT
pragma solidity ^0.8.0;contract SimpleStorage {
uint256 storedData;
function set(uint256 x) public {
storedData = x;
}
function get() public view returns (uint256) {
return storedData;
}
}
- Compile the Contract: Use the
solc
compiler to compile your Solidity code. In Remix, you can do this by clicking the "Compile" button. - Deploy the Contract: After compilation, deploy the contract to a blockchain network. In Remix, you can choose a network like Ethereum Mainnet, Ropsten Testnet, or a local development network.
- Interact with the Contract: Once deployed, you can interact with the contract using its functions. For example, you can call the
set
function to store a value and theget
function to retrieve it.
Testing and Debugging Solidity Contracts
Testing and debugging are crucial steps in smart contract development. Here's how you can test and debug your Solidity contracts:
- Unit Testing: Use frameworks like Truffle or Hardhat to write and run unit tests. These frameworks provide tools to simulate blockchain environments and test contract behavior.
- Debugging: Remix offers a built-in debugger that allows you to step through your code and inspect variables. For more advanced debugging, you can use tools like Truffle's
truffle debug
command. - Static Analysis: Tools like Slither and Mythril can perform static analysis on your Solidity code to identify potential security vulnerabilities.
Best Practices for Solidity Development
Following best practices can significantly improve the quality and security of your smart contracts:
- Use the Latest Version of Solidity: Always use the latest stable version of Solidity to benefit from the latest features and security enhancements.
- Avoid Using
tx.origin
:tx.origin
can be a security risk in certain scenarios. Instead, usemsg.sender
for authentication. - Implement Access Control: Use modifiers like
onlyOwner
to restrict access to certain functions. - Handle Reentrancy: Use the checks-effects-interactions pattern to prevent reentrancy attacks.
- Optimize Gas Usage: Minimize gas costs by optimizing your code and using efficient data structures.
Common Use Cases for Solidity Smart Contracts
Solidity smart contracts are used in various applications within the cryptocurrency ecosystem:
- Decentralized Finance (DeFi): Smart contracts power DeFi platforms, enabling lending, borrowing, and trading without intermediaries.
- Non-Fungible Tokens (NFTs): Solidity is used to create and manage NFTs, which represent unique digital assets.
- Token Creation: Many cryptocurrencies and tokens are created using Solidity smart contracts, such as ERC-20 and ERC-721 tokens.
- Supply Chain Management: Smart contracts can automate and secure supply chain processes, ensuring transparency and traceability.
Frequently Asked Questions
Q: Can Solidity be used on blockchains other than Ethereum?
A: While Solidity is primarily designed for Ethereum, it can be used on other blockchain platforms that support the Ethereum Virtual Machine (EVM), such as Binance Smart Chain and Polygon.
Q: What are the main security concerns when developing Solidity smart contracts?
A: Common security concerns include reentrancy attacks, integer overflow/underflow, and improper access control. It's essential to follow best practices and use security tools to mitigate these risks.
Q: How can I learn Solidity if I'm new to programming?
A: If you're new to programming, start with basic programming concepts using languages like JavaScript or Python. Once you have a solid foundation, you can move to Solidity-specific resources like online courses, tutorials, and the official Solidity documentation.
Q: Are there any alternatives to Solidity for smart contract development?
A: Yes, there are alternatives like Vyper, which is also designed for the EVM but focuses on simplicity and security. For non-EVM blockchains, languages like Rust (for Solana) and Go (for Hyperledger Fabric) are used for smart contract development.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- 2025-W Uncirculated American Gold Eagle and Dr. Vera Rubin Quarter Mark New Products
- 2025-06-13 06:25:13
- Ruvi AI (RVU) Leverages Blockchain and Artificial Intelligence to Disrupt Marketing, Entertainment, and Finance
- 2025-06-13 07:05:12
- H100 Group AB Raises 101 Million SEK (Approximately $10.6 Million) to Bolster Bitcoin Reserves
- 2025-06-13 06:25:13
- Galaxy Digital CEO Mike Novogratz Says Bitcoin Will Replace Gold and Go to $1,000,000
- 2025-06-13 06:45:13
- Trust Wallet Token (TWT) Price Drops 5.7% as RWA Integration Plans Ignite Excitement
- 2025-06-13 06:45:13
- Ethereum (ETH) Is in the Second Phase of a Three-Stage Market Cycle
- 2025-06-13 07:25:13
Related knowledge
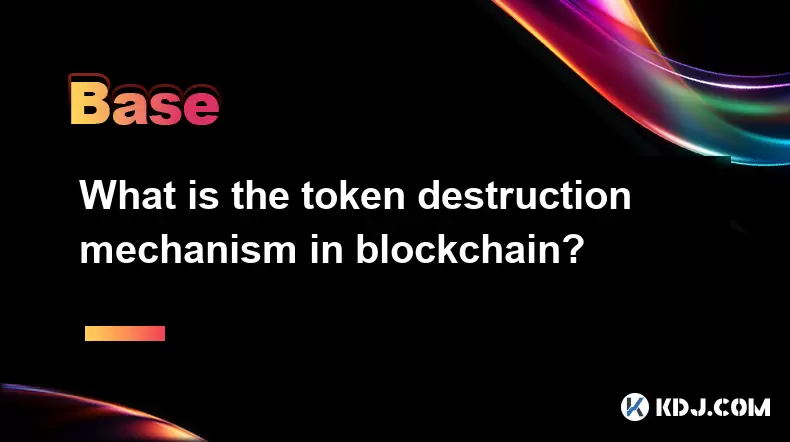
What is the token destruction mechanism in blockchain?
Jun 15,2025 at 12:14pm
Understanding Token Destruction in BlockchainToken destruction, often referred to as token burning, is a mechanism used within blockchain ecosystems to permanently remove a certain number of tokens from circulation. This process typically involves sending tokens to an irretrievable wallet address — commonly known as a burn address or eater address — whi...
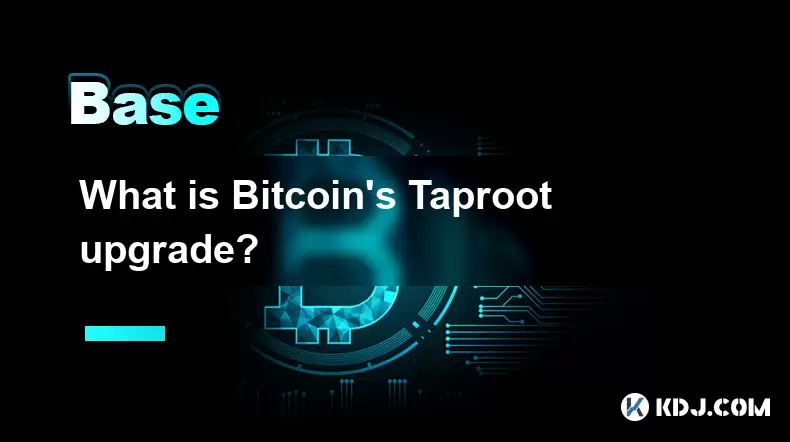
What is Bitcoin's Taproot upgrade?
Jun 14,2025 at 06:21am
Understanding the Basics of Bitcoin's Taproot UpgradeBitcoin's Taproot upgrade is a significant soft fork improvement introduced to enhance privacy, scalability, and smart contract functionality on the Bitcoin network. Activated in November 2021, Taproot represents one of the most notable upgrades since SegWit (Segregated Witness) in 2017. At its core, ...
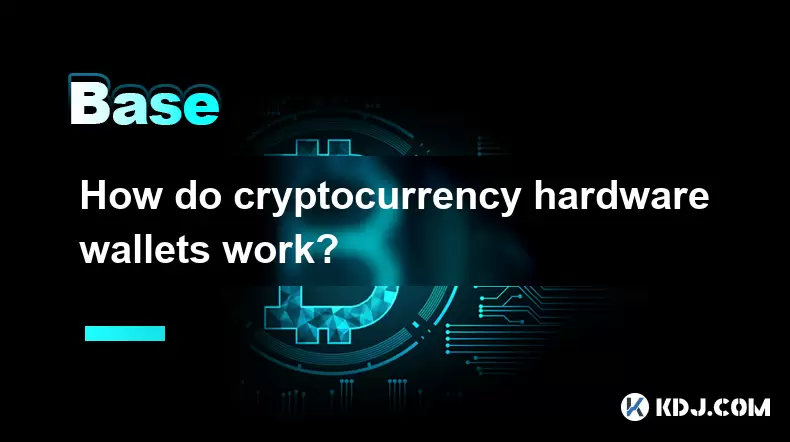
How do cryptocurrency hardware wallets work?
Jun 14,2025 at 11:28am
Understanding the Basics of Cryptocurrency Hardware WalletsCryptocurrency hardware wallets are physical devices designed to securely store users' private keys offline, offering a high level of protection against online threats. Unlike software wallets that remain connected to the internet, hardware wallets keep private keys isolated from potentially com...
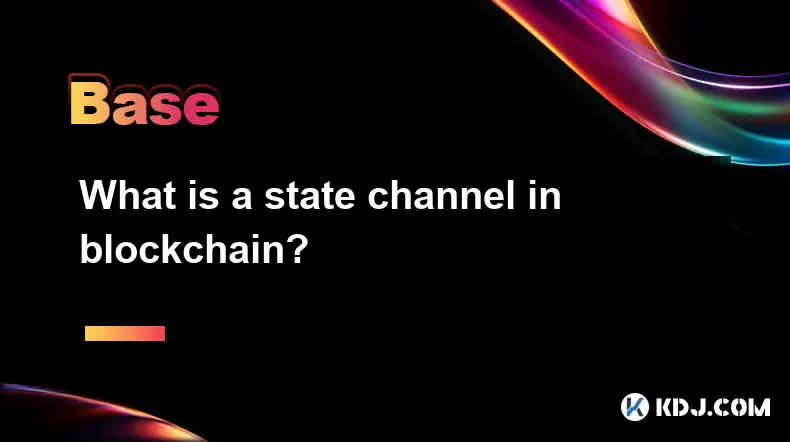
What is a state channel in blockchain?
Jun 18,2025 at 02:42am
Understanding the Concept of a State ChannelA state channel is a mechanism in blockchain technology that enables participants to conduct multiple transactions off-chain while only interacting with the blockchain for opening and closing the channel. This technique enhances scalability by reducing congestion on the main chain, allowing faster and cheaper ...
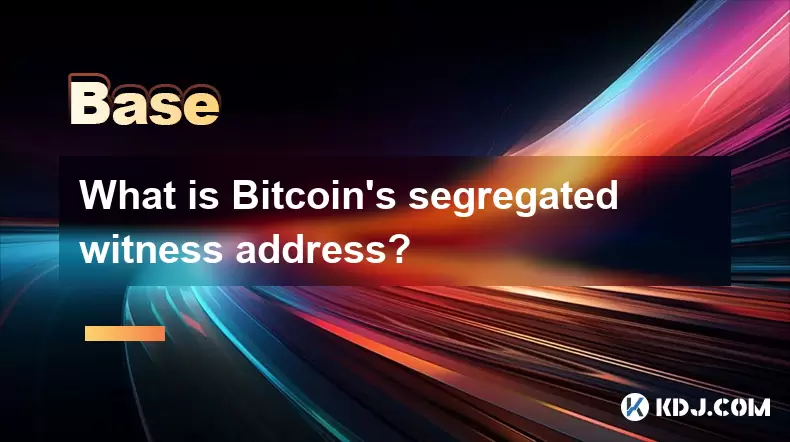
What is Bitcoin's segregated witness address?
Jun 16,2025 at 04:14pm
Understanding the Concept of Segregated Witness (SegWit)Bitcoin's Segregated Witness (SegWit) is a protocol upgrade implemented in 2017 to improve the scalability and efficiency of Bitcoin transactions. SegWit addresses were introduced as part of this upgrade, designed to separate (or 'segregate') signature data from transaction data. This separation al...
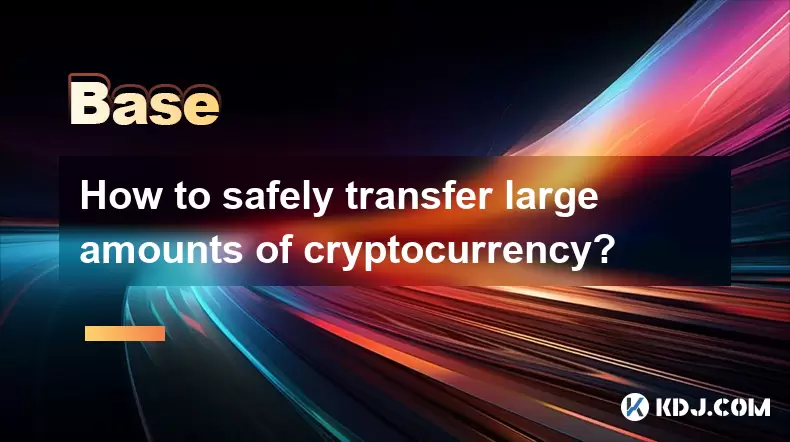
How to safely transfer large amounts of cryptocurrency?
Jun 17,2025 at 03:35pm
Understanding the Risks Involved in Transferring Large AmountsTransferring large amounts of cryptocurrency involves a unique set of risks that differ from regular transactions. The most critical risk is exposure to theft via compromised private keys or phishing attacks. Additionally, network congestion can lead to delayed confirmations, and incorrect wa...
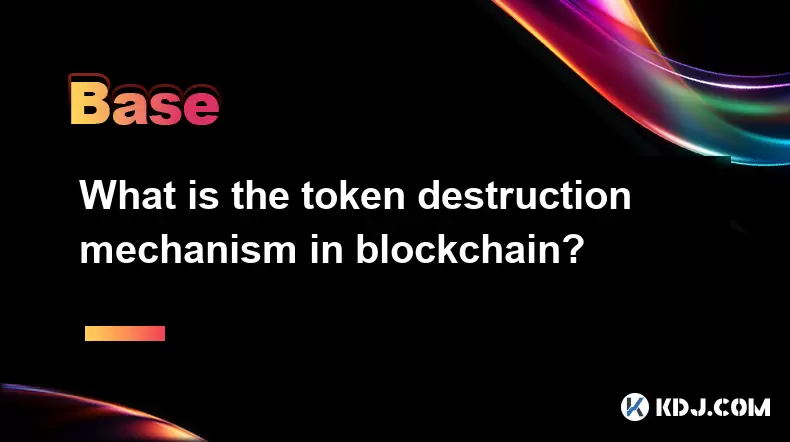
What is the token destruction mechanism in blockchain?
Jun 15,2025 at 12:14pm
Understanding Token Destruction in BlockchainToken destruction, often referred to as token burning, is a mechanism used within blockchain ecosystems to permanently remove a certain number of tokens from circulation. This process typically involves sending tokens to an irretrievable wallet address — commonly known as a burn address or eater address — whi...
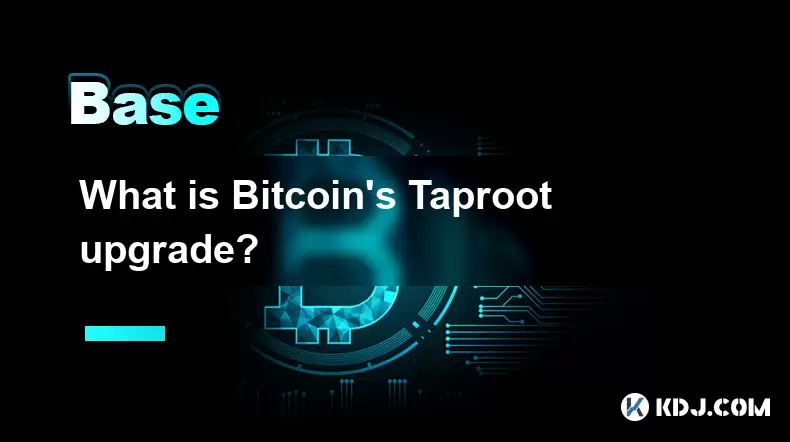
What is Bitcoin's Taproot upgrade?
Jun 14,2025 at 06:21am
Understanding the Basics of Bitcoin's Taproot UpgradeBitcoin's Taproot upgrade is a significant soft fork improvement introduced to enhance privacy, scalability, and smart contract functionality on the Bitcoin network. Activated in November 2021, Taproot represents one of the most notable upgrades since SegWit (Segregated Witness) in 2017. At its core, ...
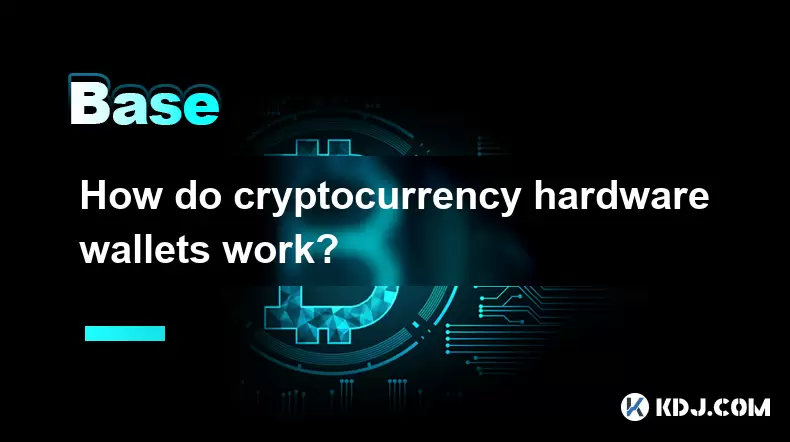
How do cryptocurrency hardware wallets work?
Jun 14,2025 at 11:28am
Understanding the Basics of Cryptocurrency Hardware WalletsCryptocurrency hardware wallets are physical devices designed to securely store users' private keys offline, offering a high level of protection against online threats. Unlike software wallets that remain connected to the internet, hardware wallets keep private keys isolated from potentially com...
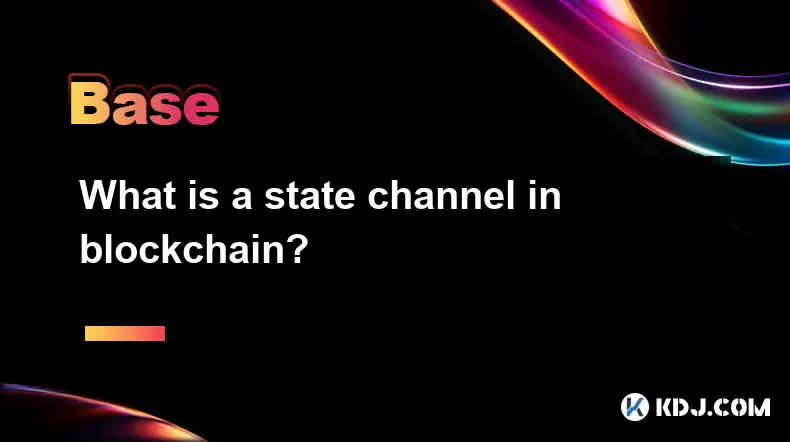
What is a state channel in blockchain?
Jun 18,2025 at 02:42am
Understanding the Concept of a State ChannelA state channel is a mechanism in blockchain technology that enables participants to conduct multiple transactions off-chain while only interacting with the blockchain for opening and closing the channel. This technique enhances scalability by reducing congestion on the main chain, allowing faster and cheaper ...
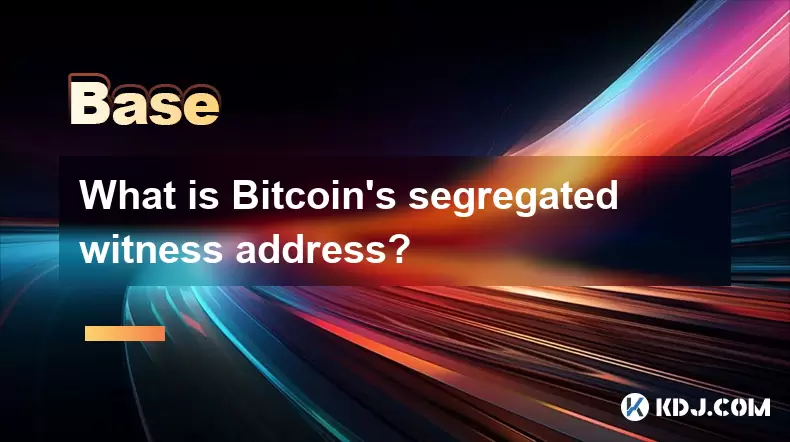
What is Bitcoin's segregated witness address?
Jun 16,2025 at 04:14pm
Understanding the Concept of Segregated Witness (SegWit)Bitcoin's Segregated Witness (SegWit) is a protocol upgrade implemented in 2017 to improve the scalability and efficiency of Bitcoin transactions. SegWit addresses were introduced as part of this upgrade, designed to separate (or 'segregate') signature data from transaction data. This separation al...
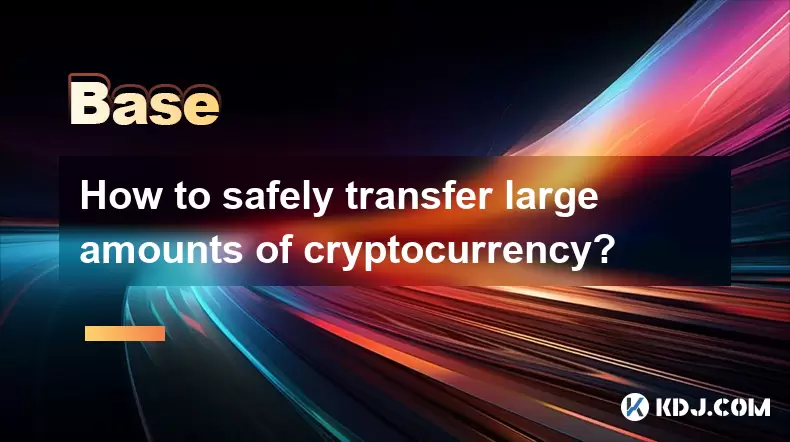
How to safely transfer large amounts of cryptocurrency?
Jun 17,2025 at 03:35pm
Understanding the Risks Involved in Transferring Large AmountsTransferring large amounts of cryptocurrency involves a unique set of risks that differ from regular transactions. The most critical risk is exposure to theft via compromised private keys or phishing attacks. Additionally, network congestion can lead to delayed confirmations, and incorrect wa...
See all articles
