-
Bitcoin
$94,092.2703
-0.29% -
Ethereum
$1,799.1701
-0.39% -
Tether USDt
$1.0003
-0.02% -
XRP
$2.2315
1.42% -
BNB
$601.0919
-1.03% -
Solana
$149.1813
-0.03% -
USDC
$1.0000
0.01% -
Dogecoin
$0.1796
-1.12% -
Cardano
$0.6979
-1.47% -
TRON
$0.2491
-1.02% -
Sui
$3.5910
3.48% -
Chainlink
$14.5359
-2.27% -
Avalanche
$21.7970
-0.90% -
Stellar
$0.2830
-2.31% -
UNUS SED LEO
$9.0082
-0.81% -
Toncoin
$3.2314
-2.12% -
Shiba Inu
$0.0...01348
-5.09% -
Hedera
$0.1871
-2.69% -
Bitcoin Cash
$351.4456
-2.28% -
Litecoin
$86.3202
-1.04% -
Polkadot
$4.0892
-4.60% -
Hyperliquid
$17.8780
0.04% -
Dai
$1.0001
0.00% -
Bitget Token
$4.3692
-0.76% -
Ethena USDe
$0.9995
-0.01% -
Pi
$0.6305
-2.50% -
Monero
$228.7745
-0.04% -
Pepe
$0.0...08790
-3.92% -
Uniswap
$5.5526
-4.49% -
Aptos
$5.3721
-4.12%
What is a reentrancy attack? How to prevent this vulnerability?
Reentrancy attacks exploit smart contract flaws, allowing repeated function calls before state resolution, leading to unauthorized actions; prevent with checks-effects-interactions pattern.
Apr 12, 2025 at 12:35 am
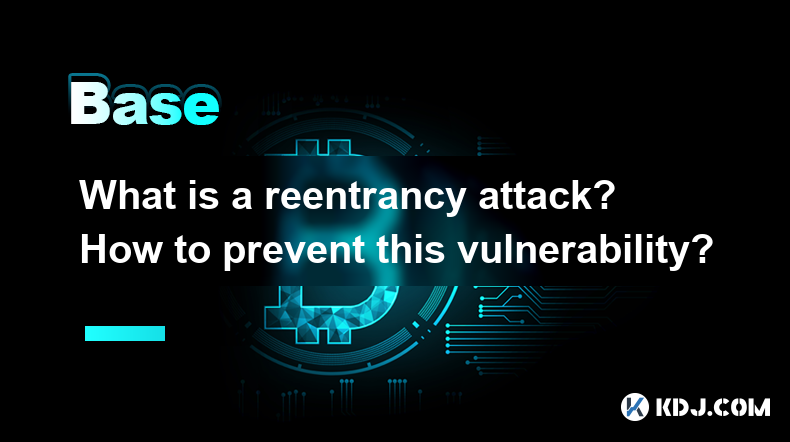
A reentrancy attack is a type of security vulnerability that can occur in smart contracts, particularly those on the Ethereum blockchain. This attack exploits a flaw in the contract's logic that allows an attacker to repeatedly call a function before the initial call is fully resolved. This can lead to unauthorized withdrawals or other malicious actions. In this article, we will explore the mechanics of a reentrancy attack, examine real-world examples, and provide detailed guidance on how to prevent this vulnerability in your smart contracts.
Understanding Reentrancy Attacks
A reentrancy attack occurs when a smart contract calls an external contract before resolving its own state changes. This can create a window of opportunity for the external contract to reenter the original contract and manipulate its state. The attack typically involves a malicious contract that drains funds from the victim contract by repeatedly calling a function such as withdraw()
before the victim contract can update its balance.
To illustrate, consider a simple example of a contract that allows users to deposit and withdraw funds:
contract Vulnerable {mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Transfer failed");
balances[msg.sender] -= amount;
}
}
In this example, the withdraw
function first checks if the user has sufficient balance, then attempts to send the funds to the user, and finally updates the user's balance. The vulnerability lies in the fact that the balances[msg.sender]
is not updated until after the external call to msg.sender.call
. If the msg.sender
is a malicious contract, it can reenter the withdraw
function before the balance is updated, allowing multiple withdrawals before the balance is set to zero.
Real-World Examples of Reentrancy Attacks
One of the most infamous reentrancy attacks occurred during the DAO hack in 2016. The DAO (Decentralized Autonomous Organization) was a smart contract on the Ethereum blockchain that allowed users to invest in projects. The contract had a vulnerability similar to the one described above, which allowed an attacker to drain approximately 3.6 million ETH from the DAO.
Another example is the Parity Wallet hack in 2017. The Parity Wallet, a popular multi-signature wallet on Ethereum, was exploited due to a reentrancy vulnerability. The attacker was able to drain funds from multiple wallets, resulting in significant losses for users.
How to Prevent Reentrancy Attacks
Preventing reentrancy attacks requires careful design and implementation of smart contracts. Here are some strategies to mitigate this vulnerability:
Use the Checks-Effects-Interactions Pattern
The Checks-Effects-Interactions pattern is a best practice for writing secure smart contracts. This pattern ensures that all state changes are made before any external calls are executed. In the context of the withdraw
function, this means updating the user's balance before sending the funds:
contract Secure {mapping(address => uint) public balances;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function withdraw(uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Transfer failed");
}
}
By updating the balance before making the external call, the contract ensures that the user's balance is correctly set to zero before any reentrancy can occur.
Use the Withdrawal Pattern
Another effective way to prevent reentrancy attacks is to use the withdrawal pattern. Instead of directly sending funds to users, the contract stores the withdrawal amount and allows users to pull their funds at a later time. This approach eliminates the need for external calls during the withdrawal process:
contract WithdrawalPattern {mapping(address => uint) public balances;
mapping(address => uint) public withdrawalPending;
function deposit() public payable {
balances[msg.sender] += msg.value;
}
function requestWithdrawal(uint amount) public {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
withdrawalPending[msg.sender] += amount;
}
function withdraw() public {
uint amount = withdrawalPending[msg.sender];
require(amount > 0, "No pending withdrawal");
withdrawalPending[msg.sender] = 0;
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Transfer failed");
}
}
In this example, the requestWithdrawal
function updates the user's balance and stores the withdrawal amount in withdrawalPending
. The withdraw
function then sends the funds to the user without any risk of reentrancy.
Implement Reentrancy Guards
Reentrancy guards are another technique to prevent reentrancy attacks. These guards use a state variable to track whether a function is currently being executed. If a function is reentered, the guard will prevent further execution:
contract ReentrancyGuard {bool private _notEntered;
constructor() {
_notEntered = true;
}
modifier nonReentrant() {
require(_notEntered, "ReentrancyGuard: reentrant call");
_notEntered = false;
_;
_notEntered = true;
}
function withdraw(uint amount) public nonReentrant {
require(balances[msg.sender] >= amount, "Insufficient balance");
balances[msg.sender] -= amount;
(bool success, ) = msg.sender.call{value: amount}("");
require(success, "Transfer failed");
}
}
The nonReentrant
modifier ensures that the withdraw
function cannot be reentered while it is still executing.
Testing and Auditing for Reentrancy Vulnerabilities
In addition to implementing preventive measures, it is crucial to thoroughly test and audit your smart contracts for reentrancy vulnerabilities. Here are some steps to follow:
- Unit Testing: Write unit tests that simulate reentrancy attacks to ensure your contract behaves correctly under such conditions.
- Static Analysis: Use tools like Mythril and Slither to automatically detect potential reentrancy vulnerabilities in your code.
- Manual Auditing: Have experienced smart contract auditors review your code for any potential reentrancy issues. Manual audits can uncover complex vulnerabilities that automated tools might miss.
Best Practices for Smart Contract Development
To further reduce the risk of reentrancy attacks, consider the following best practices:
- Keep Contracts Simple: Complex contracts are more likely to contain vulnerabilities. Keep your contracts as simple and straightforward as possible.
- Use Established Libraries: Leverage well-audited libraries and frameworks, such as OpenZeppelin, which provide secure implementations of common contract patterns.
- Regular Updates: Stay informed about the latest security best practices and update your contracts accordingly.
Frequently Asked Questions
Q: Can reentrancy attacks occur in other blockchain platforms besides Ethereum?
A: While reentrancy attacks are most commonly associated with Ethereum due to its widespread use of smart contracts, similar vulnerabilities can occur on other blockchain platforms that support smart contracts, such as Binance Smart Chain and Solana. The principles of preventing reentrancy attacks remain the same across different platforms.
Q: Are there any tools specifically designed to detect reentrancy vulnerabilities?
A: Yes, several tools are designed to detect reentrancy vulnerabilities in smart contracts. Mythril and Slither are popular static analysis tools that can identify potential reentrancy issues. Additionally, Echidna is a property-based testing tool that can be used to test for reentrancy vulnerabilities through automated test case generation.
Q: How can I ensure that my smart contract is secure against reentrancy attacks if I am not a security expert?
A: If you are not a security expert, it is highly recommended to engage professional smart contract auditors to review your code. Additionally, using established libraries like OpenZeppelin and following best practices such as the Checks-Effects-Interactions pattern can significantly reduce the risk of reentrancy vulnerabilities. Regularly updating your knowledge on smart contract security and participating in community discussions can also help you stay informed about the latest security practices.
Disclaimer:info@kdj.com
The information provided is not trading advice. kdj.com does not assume any responsibility for any investments made based on the information provided in this article. Cryptocurrencies are highly volatile and it is highly recommended that you invest with caution after thorough research!
If you believe that the content used on this website infringes your copyright, please contact us immediately (info@kdj.com) and we will delete it promptly.
- If You Missed Solana's Explosive Breakout, There May Still Be Time to Catch the Next Big Opportunity—Lightchain AI
- 2025-04-28 01:20:13
- DungeonQuest
- 2025-04-28 01:20:13
- Bitcoin: the paradox of the discount and the voracious appetite of institutions
- 2025-04-28 01:15:12
- At $0.006695, Unstaked Could Outperform $0.2412 TRX and $0.57 ADA by 28x
- 2025-04-28 01:15:12
- Bitcoin (BTC) Price Cycle Theory Under Threat, Investors Could See The First Unique Cycle In Bitcoin's History
- 2025-04-28 01:10:13
- PEPE's 24% rally shows resilience. Despite a dip, the meme coin could break past $0.000010 in May. Is PEPE's growth momentum here to stay.
- 2025-04-28 01:10:13
Related knowledge
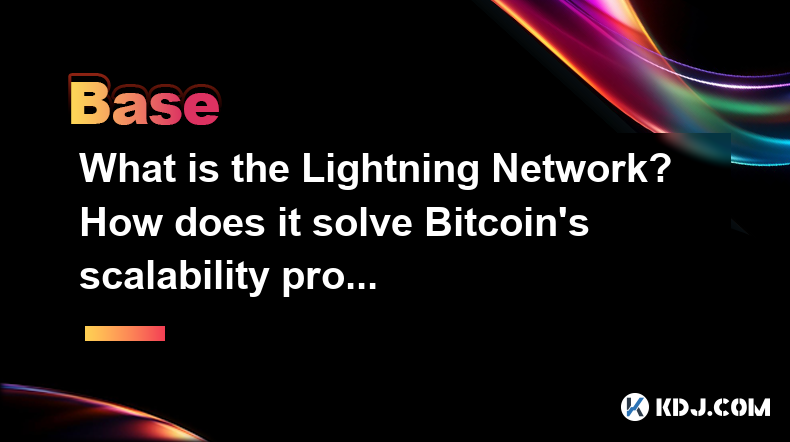
What is the Lightning Network? How does it solve Bitcoin's scalability problem?
Apr 27,2025 at 03:00pm
The Lightning Network is a second-layer solution built on top of the Bitcoin blockchain to enhance its scalability and transaction speed. It operates as an off-chain network of payment channels that allow users to conduct multiple transactions without the need to commit each transaction to the Bitcoin blockchain. This significantly reduces the load on t...
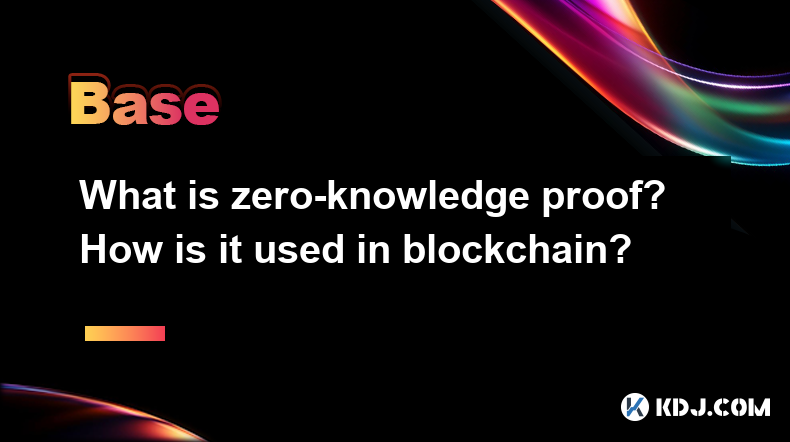
What is zero-knowledge proof? How is it used in blockchain?
Apr 27,2025 at 01:14pm
Zero-knowledge proof (ZKP) is a cryptographic method that allows one party to prove to another that a given statement is true, without conveying any additional information apart from the fact that the statement is indeed true. This concept, which emerged from the field of theoretical computer science in the 1980s, has found significant applications in t...
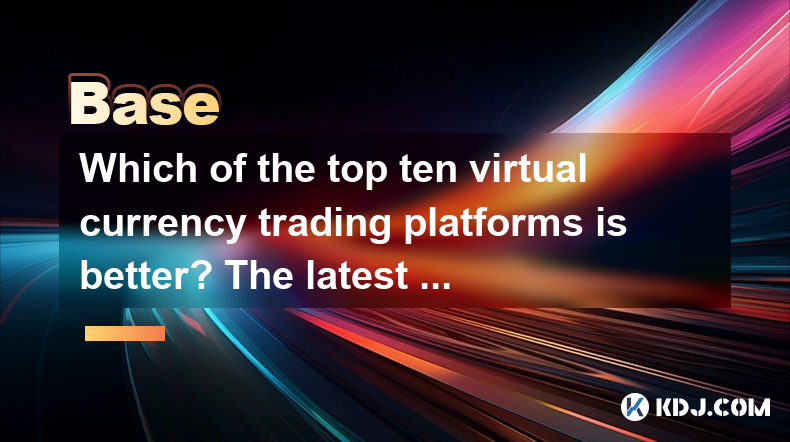
Which of the top ten virtual currency trading platforms is the latest ranking of cryptocurrency trading platforms apps
Apr 26,2025 at 11:57pm
Which of the top ten virtual currency trading platforms is the latest ranking of cryptocurrency trading platforms apps When choosing a digital currency trading platform, it is crucial to consider factors such as user experience, security, transaction volume and currency support. The following is the ranking of the top ten digital currency trading platfo...
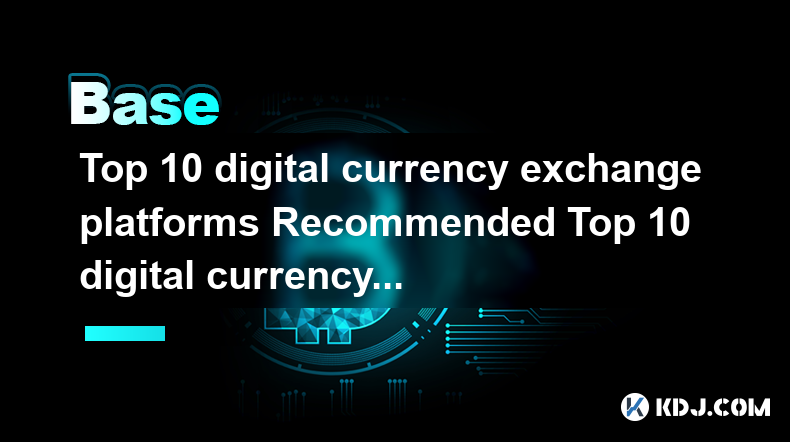
Top 10 digital currency exchange platforms recommend digital currency exchanges
Apr 26,2025 at 01:00pm
In the cryptocurrency space, choosing a reliable trading platform is crucial. This article will introduce the latest rankings of the top ten exchanges in the currency circle in detail, and will explore the characteristics and advantages of each platform in depth. These rankings are selected based on many factors such as user experience, security, and tr...
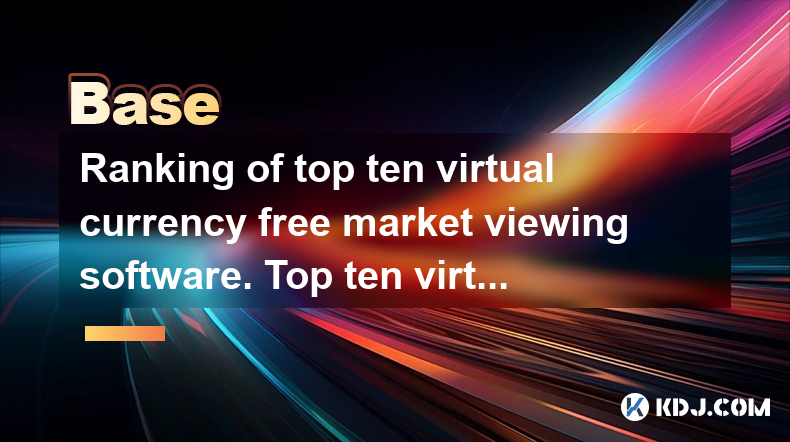
Top 10 Virtual Currency Free Market Viewing Software Rankings of Top 10 Virtual Currency Exchanges in 2025
Apr 25,2025 at 09:21pm
In the cryptocurrency market, it is crucial to choose a reliable and powerful exchange app. This article will provide you with a detailed analysis of the top ten virtual currency exchange rankings in 2025 to help you better understand the top platforms in the market. Binance Binance is one of the world's leading cryptocurrency exchanges, known for i...
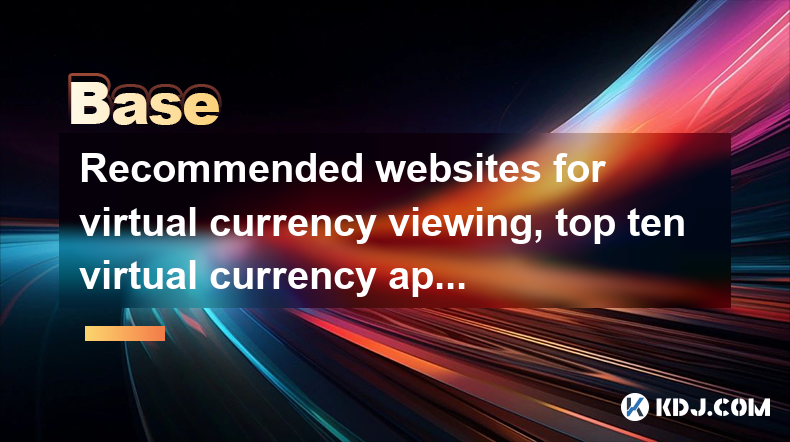
The top ten virtual currency app exchange platforms recommended in the currency circle
Apr 26,2025 at 06:50pm
Recommended virtual currency trading websites and top ten virtual currency exchange platforms In the field of digital currency trading, choosing a reliable and powerful trading platform is crucial. The following is the ranking of the top ten digital currency trading platforms selected based on multiple factors such as user experience, security, transact...
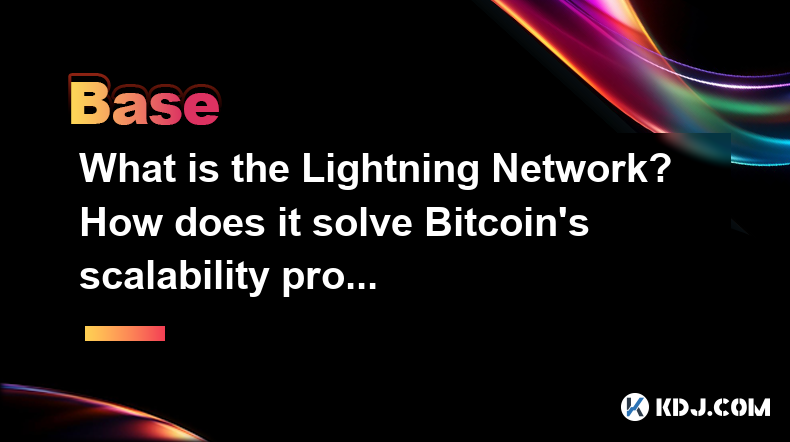
What is the Lightning Network? How does it solve Bitcoin's scalability problem?
Apr 27,2025 at 03:00pm
The Lightning Network is a second-layer solution built on top of the Bitcoin blockchain to enhance its scalability and transaction speed. It operates as an off-chain network of payment channels that allow users to conduct multiple transactions without the need to commit each transaction to the Bitcoin blockchain. This significantly reduces the load on t...
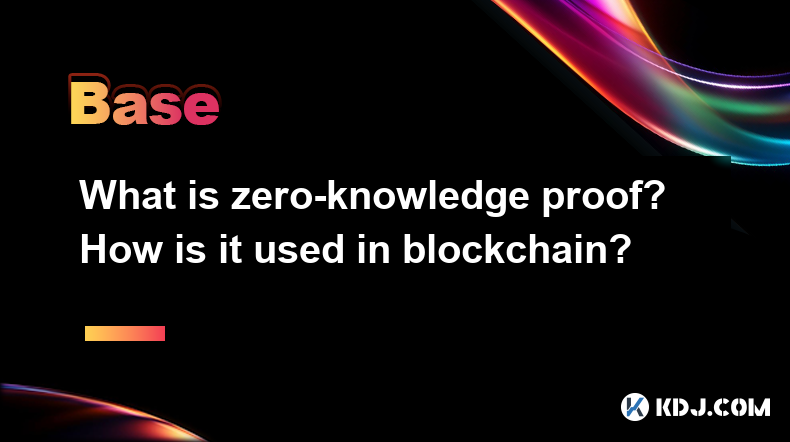
What is zero-knowledge proof? How is it used in blockchain?
Apr 27,2025 at 01:14pm
Zero-knowledge proof (ZKP) is a cryptographic method that allows one party to prove to another that a given statement is true, without conveying any additional information apart from the fact that the statement is indeed true. This concept, which emerged from the field of theoretical computer science in the 1980s, has found significant applications in t...
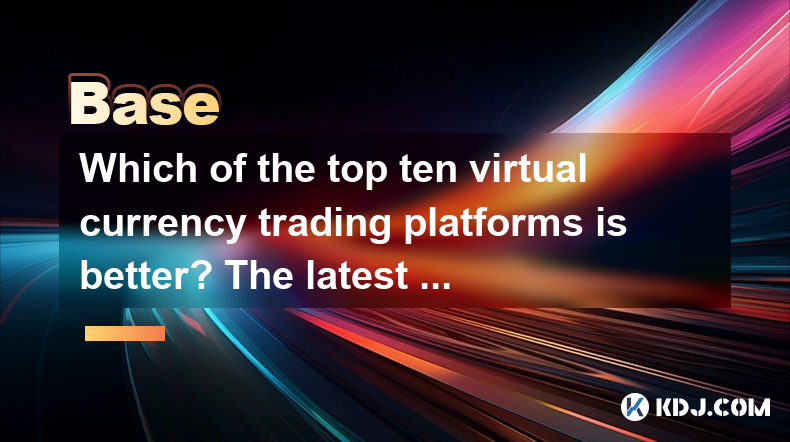
Which of the top ten virtual currency trading platforms is the latest ranking of cryptocurrency trading platforms apps
Apr 26,2025 at 11:57pm
Which of the top ten virtual currency trading platforms is the latest ranking of cryptocurrency trading platforms apps When choosing a digital currency trading platform, it is crucial to consider factors such as user experience, security, transaction volume and currency support. The following is the ranking of the top ten digital currency trading platfo...
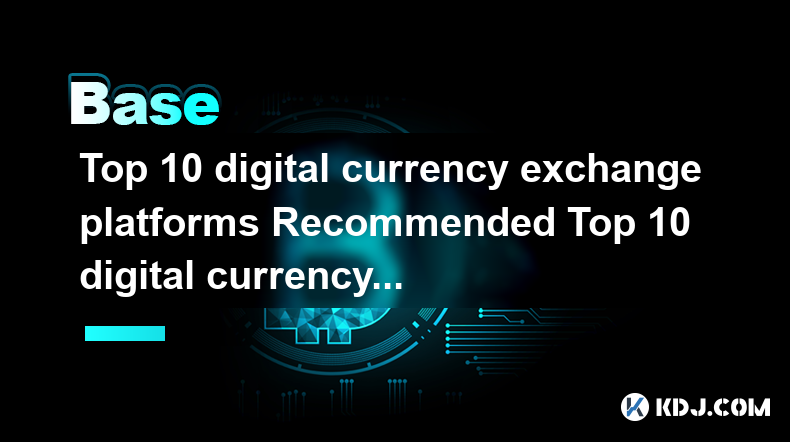
Top 10 digital currency exchange platforms recommend digital currency exchanges
Apr 26,2025 at 01:00pm
In the cryptocurrency space, choosing a reliable trading platform is crucial. This article will introduce the latest rankings of the top ten exchanges in the currency circle in detail, and will explore the characteristics and advantages of each platform in depth. These rankings are selected based on many factors such as user experience, security, and tr...
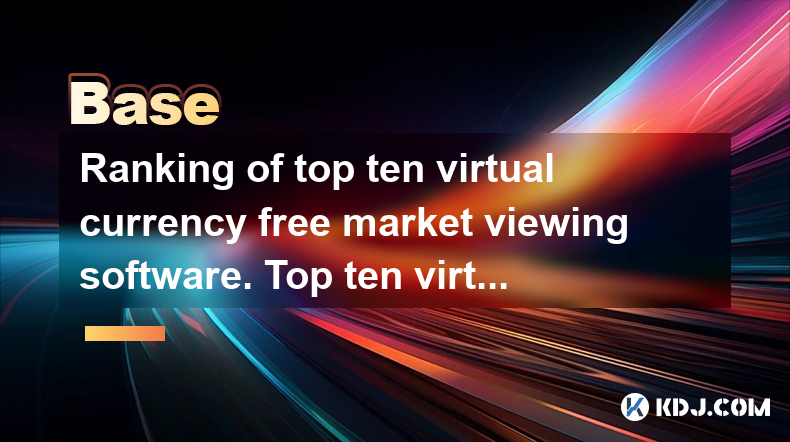
Top 10 Virtual Currency Free Market Viewing Software Rankings of Top 10 Virtual Currency Exchanges in 2025
Apr 25,2025 at 09:21pm
In the cryptocurrency market, it is crucial to choose a reliable and powerful exchange app. This article will provide you with a detailed analysis of the top ten virtual currency exchange rankings in 2025 to help you better understand the top platforms in the market. Binance Binance is one of the world's leading cryptocurrency exchanges, known for i...
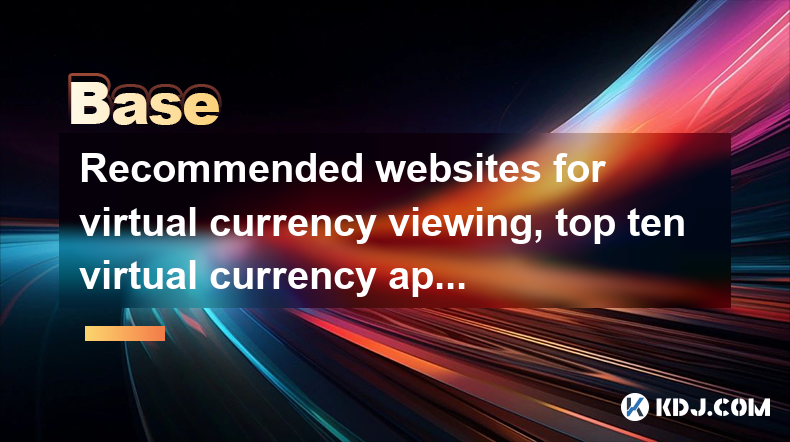
The top ten virtual currency app exchange platforms recommended in the currency circle
Apr 26,2025 at 06:50pm
Recommended virtual currency trading websites and top ten virtual currency exchange platforms In the field of digital currency trading, choosing a reliable and powerful trading platform is crucial. The following is the ranking of the top ten digital currency trading platforms selected based on multiple factors such as user experience, security, transact...
See all articles
